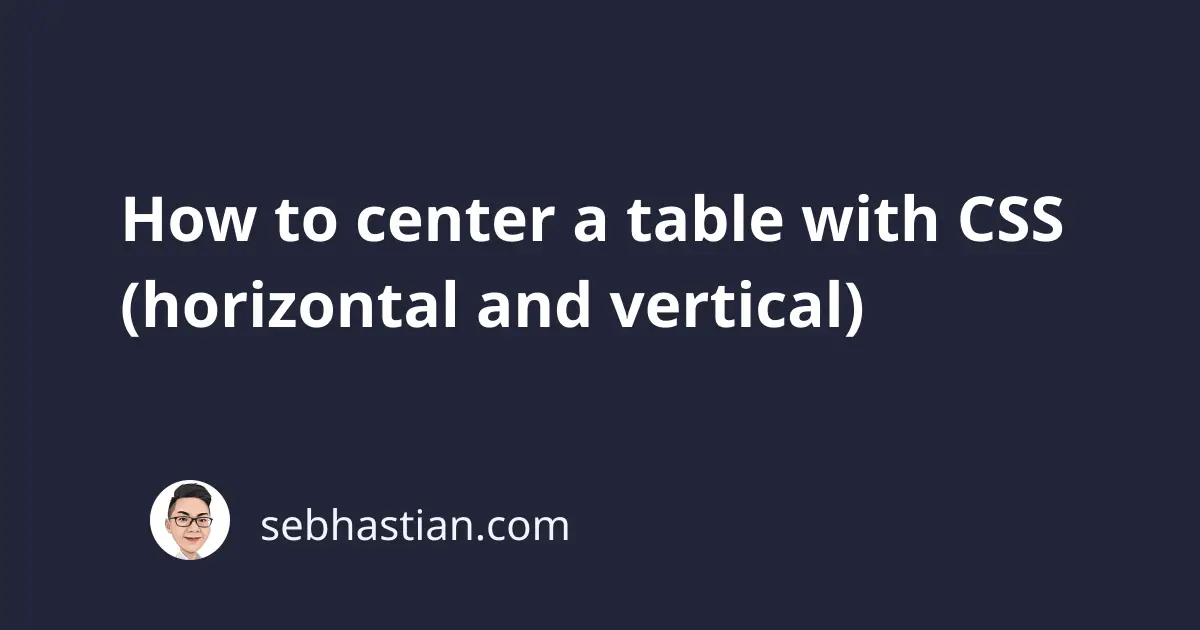
The CSS style to center a table differs depending on whether you want the table to be centered horizontally or vertically.
Center table horizontally
To center a table horizontally, you need to set the margin-left
and margin-right
properties to auto
.
It’s recommended that you create a class that applies the CSS style so that it won’t affect all of your tables. Here is an example of how to do this:
.center {
margin-left: auto;
margin-right: auto;
}
Add that class to your table as follows:
<table class="center">
<tr>
<th>Name</th>
<th>Age</th>
</tr>
<tr>
<td>Alice</td>
<td>25</td>
</tr>
<tr>
<td>Bob</td>
<td>32</td>
</tr>
<tr>
<td>Charlie</td>
<td>28</td>
</tr>
</table>
You will have the table centered horizontally on the browser as shown below:
Alternatively, you can also define a single margin
property and set the value to 0 auto
like this:
.center {
margin: 0 auto;
}
The margins on top and bottom will be set to 0
while the left and right margins will be set to auto
.
Center table vertically
To center the table vertically on the browser, you need to make use of CSS Flexbox layout and create a container element for the table (like a <div>
).
The container element must also have a fixed height
property for the vertical centering to work.
First, you need to write the CSS for the container as follows:
.flex-center {
display: flex;
align-items: center;
height: 500px; /* adjust as you need */
}
The height
property of the container must be higher than the table, or the centering won’t work.
Add that CSS to the element that contains your table as shown below:
<div class="flex-center">
<table>
...
</table>
</div>
You will see the table rendered in the center vertically like this:
Center table vertically and horizontally
If you want the table to be centered vertically and horizontally, add justify-content: center;
property to the flex-center
class:
.flex-center {
display: flex;
align-items: center;
justify-content: center;
height: 500px; /* adjust as you need */
}
With that property, the table will be centered horizontally and vertically inside the <div>
as follows:
Conclusion
To conclude, centering a table with CSS can be done vertically or horizontally, or both.
You can use the CSS styles shown in this tutorial to help you achieve the desired output.
Another element that you might want to center a lot is a button, so I also created a guide on centering button elements with CSS.
You might want to check it out.