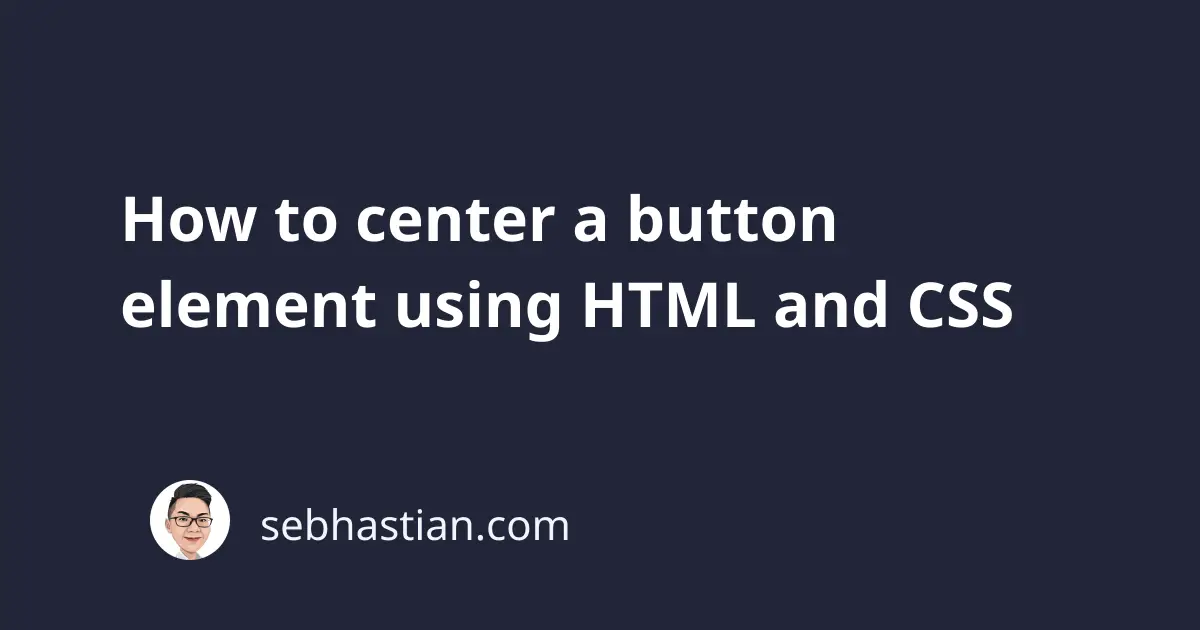
To center an HTML <button>
element, you need to add certain CSS properties that will put the button at the center of your HTML web page.
Button center horizontally with text-align
The text-align
property is used to specify the horizontal alignment of text in a block element.
One trick of this property is that it can also be used to set the alignment of children elements in a parent <div>
tag.
For example, suppose you have a <div>
tag that contains two buttons. You need to add text-align
to the <div>
tag as follows:
<div style="text-align:center">
<button>Click me</button>
<button>No, click me instead</button>
</div>
The output will be as shown below:
Button center horizontally using margin auto
Alternatively, you can also set the button in the middle of the <body>
tag.
Set the display
property to block
and add margin: 0 auto
to the <button>
tag as shown below:
<!DOCTYPE html>
<html>
<head>
<!-- head content... -->
</head>
<body>
<button style="display:block; margin: 0 auto;">
Click me
</button>
</body>
</html>
Here’s the result:
This is useful when your button has no parent element.
How to center button horizontally and vertically
To center a <button>
horizontally and vertically, you can use a combination of display
, justify-content
, and align-items
properties.
Suppose you have a <div>
as the container of the <button>
element.
To center the button horizontally:
- Set the
display
property toflex
orgrid
- Set the
justify-content
property ascenter
Use css class to make your code tidy as follows:
.center-h {
display: flex; /* or display:grid */
justify-content: center;
}
Next, create another CSS class rule with the align-items
property and set it to center
.
Also add the display
property here so that you can center vertically without the center-h
class.
.center-v {
display: flex;
align-items: center;
}
To see the content centered vertically, let’s add one more class to set the <div>
element height
to 500
:
.h-500 {
height: 500;
}
Finally, add all the classes assigned to the CSS rule above to the containing <div>
tag as follows:
<div class="h-500 center-v center-h">
<button>Click me</button>
<div></div>
</div>
The output will be as shown below:
To center the button vertically but not horizontally, remove the center-h
class:
<div class="h-500 center-v">
<button>Click me</button>
<div></div>
</div>
Now you’ve learned how to center a <button>
And that’s how you can center an HTML button element using CSS properties!
I’ve also written more articles on CSS tricks that you may be interested in:
- Using CSS to center image inside a div tag
- How to make CSS fade-in animation for HTML elements
- Hide browser scrollbar with CSS
CSS is very useful for styling your web page elements, but it’s quite tricky to learn what rule to write and what property to use.
The guides I’ve written will help you to understand how to style HTML elements with CSS.
Thanks for reading! 😉