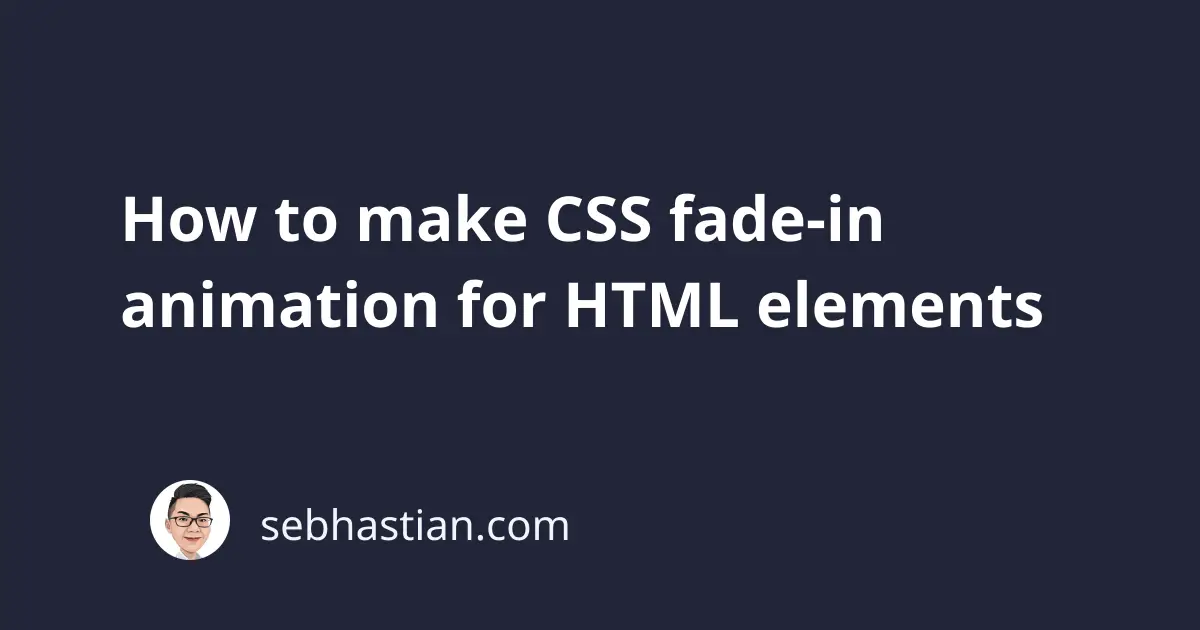
With the release of CSS3, you can now create a native CSS animation for animating your HTML elements.
One animation that you can create with CSS is the fade-in animation, where the element will slowly be turned from invisible to visible.
Here’s the code for CSS fade-in animation. I will explain the code below:
.fade-in:hover {
animation-name: FadeIn;
animation-duration: 3s;
transition-timing-function: linear;
}
@keyframes FadeIn {
0% {
opacity: 0;
}
100% {
opacity: 1;
}
}
@-moz-keyframes FadeIn {
0% {
opacity: 0;
}
100% {
opacity: 1;
}
}
@-webkit-keyframes FadeIn {
0% {
opacity: 0;
}
100% {
opacity: 1;
}
}
@-o-keyframes FadeIn {
0% {
opacity: 0;
}
100% {
opacity: 1;
}
}
@-ms-keyframes FadeIn {
0% {
opacity: 0;
}
100% {
opacity: 1;
}
}
First, a CSS rule is applied to the fade-in:hover
pseudo-class so that the animation will run when the user hovers over the element with the class.
The animation that we want to make is a fade-in animation, and it can be created by slowly transitioning the opacity of the element from 0
to 1
.
Animations in CSS are created using the @keyframes
rule, so we put the rule inside @keyframes
as follows:
0% {opacity:0;}
When the animation starts at0%
, set theopacity
to zero100% {opacity:1;}
When the animation ends at100%
, set theopacity
to one
The rules above are enough to create a fade-in animation. The prefix in front of keyframe rules above are added to support older browsers as well.
Next, the @keyframes
rules is applied to the class fade-in
by using the animation-name
property.
The animation-name
property specifies the name of the @keyframes
CSS rule that you want to apply to the element. t
The animation-duration
property specifies the duration of the animation in seconds. In the example above, it will take 3 seconds for the animation to go from 0%
to 100%
.
The last property transition-timing-function
is to control the acceleration speed of the animation effect. The linear
value means the animation will be done at a constant speed.
You can put other values in the transition-timing-function
such as:
ease
- the animation will speed up towards the middle of the transition then slows againease-in
- the animation starts slowly and then speeds upease-in
- the animation starts fast and then slows down until the endease-in-out
- like ease but with a different precise value
But if you don’t have any preference, then linear
will be a good choice because the animation will have a constant transition speed.
You can add the fade-in
class to any HTML elements, including texts, lists, tables, and images.
Let’s test the animation we’ve created above in a <div>
element. I will add a background color and paddings to make the element bigger:
<div
class="fade-in"
style="background-color: aqua; border-radius: 5px; padding: 10px"
>
<h1>Hover on me and see the animation</h1>
</div>
Now you can see the fade-in animation each time you hover over the <div>
element. Nice work 👍