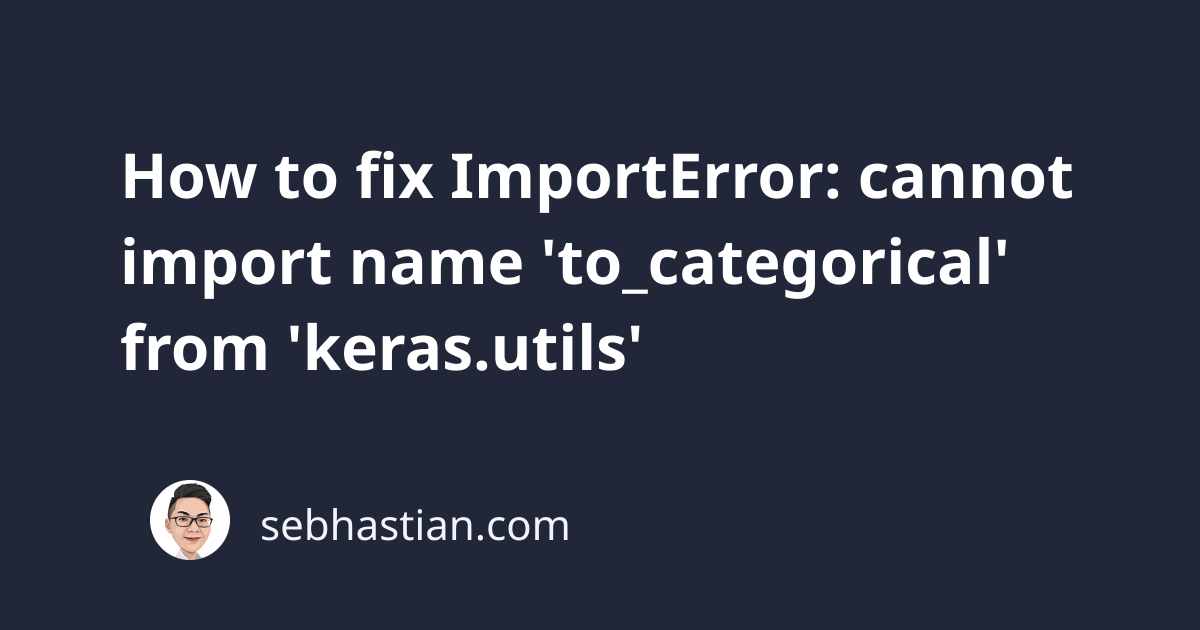
When using Keras for deep learning in TensorFlow, you might encounter the following error:
ImportError: cannot import name 'to_categorical' from 'keras.utils'
This error occurs when Python can’t find the to_categorical
function from the keras.utils
module.
The common cause for this error is that you imported to_categorical
from keras
directly as follows:
import keras
from keras.utils import to_categorical
This code works in TensorFlow version 1, but starting in TensorFlow version 2, the keras
module is now bundled with tensorflow
.
You need to change the import statement to this:
from tensorflow.keras.utils import to_categorical
So that Python can find the to_categorical()
function and you won’t receive this error.
Note that Keras is now the recommended library for deep learning in Python, and in TensorFlow version 2 Keras is no longer a standalone module. You need to change some import
statements that utilize this library.
For example, the Adam
optimizer has also been moved from keras.optimizers
to tensorflow.keras.optimizers
, so you may need to adjust your code accordingly.
I hope you find this tutorial useful. Until next time! 👋