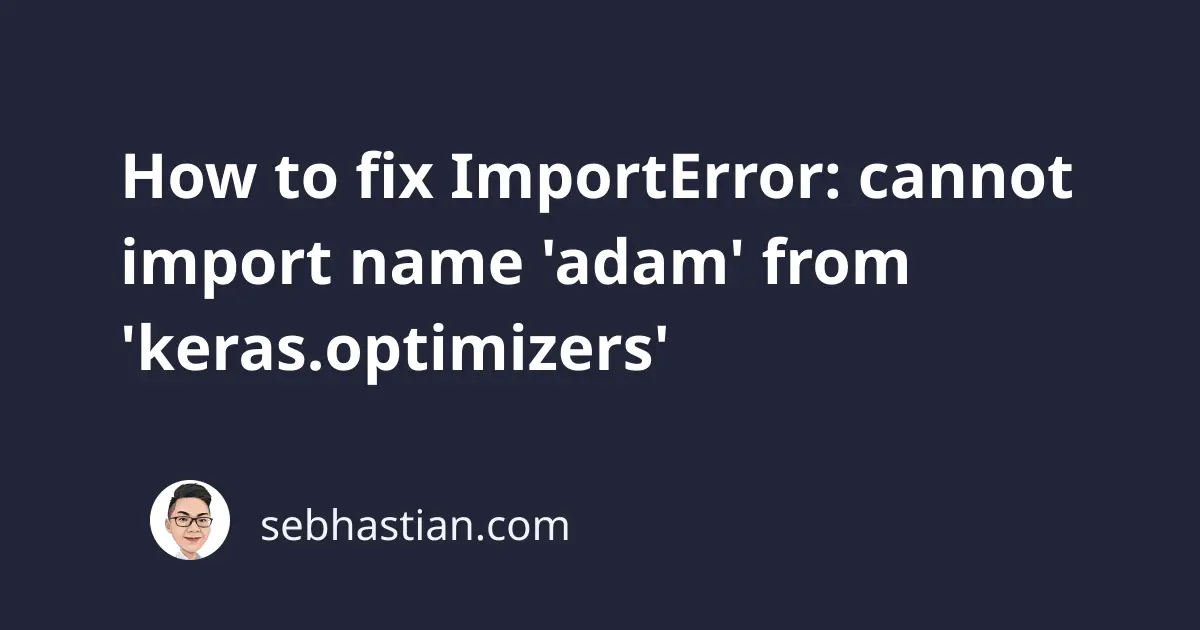
When using TensorFlow, you might encounter the following error:
ImportError: cannot import name 'adam' from 'keras.optimizers'
This error occurs when Python can’t find the class Adam
from the keras.optimizers
module.
You might have an import
statement as follows:
from keras.optimizers import adam
The right package name is Adam
with a capital ‘A’, so you need to change the import name in your source code like this:
from keras.optimizers import Adam
If that doesn’t work, try adding tensorflow
before keras
as follows:
from tensorflow.keras.optimizers import Adam
Now you can import the Adam optimizer without receiving the error.
UPDATE: In the latest TensorFlow version, a newer Adam optimizer implementation named adam
has been added. These import
statements can work:
from keras.optimizers import adam
from keras.optimizers import Adam
print(adam)
print(Adam)
Output:
<module 'keras.optimizers.adam' from 'python3.8/site-packages/keras/optimizers/adam.py'>
<class 'keras.optimizers.legacy.adam.Adam'>
The adam
with lowercase ‘a’ is the new optimizer implementation. You can call adam.Adam()
to create the optimizer:
from keras.optimizers import adam
optimizer = adam.Adam(learning_rate=0.0001)
I’ve tested the import to work in TensorFlow version 2.12.0. If you use older versions, you can use Adam
so you don’t need to upgrade TensorFlow.
I hope this tutorial helps. Happy pythoning! 🐍