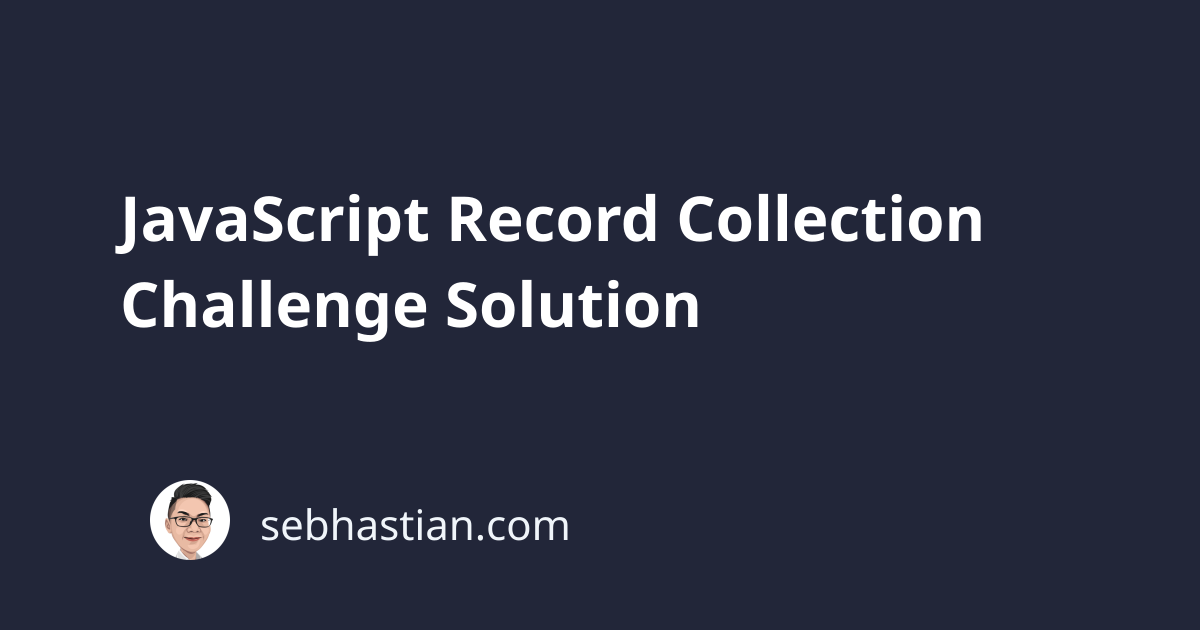
The FreeCodeCamp Record Collection challenge allows you to test your understanding of JavaScript algorithms and data structures.
In this challenge, you are given a JSON object that represents a part of your music album collection.
There’s an updateRecords()
function that can be used to modify the record collection object. It accepts 4 parameters:
- The
records
collection object - The
id
of the record you want to modify - The
prop
that determines the type of thevalue
(eithertracks
,artist
, oralbumTitle
) - And the
value
to add
You are given the task to update the updateRecords()
function with the following requirements:
- The function must always return the entire JSON object
- If
prop
is nottracks
andvalue
is not an empty string, add a new property to the album with the same name asprop
withvalue
as its value - If
prop
istracks
but the album doesn’t have atracks
property, create thetracks
property as an empty array and add thevalue
to it - if
prop
istracks
andvalue
is not an empty string, addvalue
to the end of thetracks
array - if
value
is an empty string, delete theprop
key from the album object.
The challenge gives you the following starting point:
// Setup
const recordCollection = {
2548: {
albumTitle: "Slippery When Wet",
artist: "Bon Jovi",
tracks: ["Let It Rock", "You Give Love a Bad Name"],
},
2468: {
albumTitle: "1999",
artist: "Prince",
tracks: ["1999", "Little Red Corvette"],
},
1245: {
artist: "Robert Palmer",
tracks: [],
},
5439: {
albumTitle: "ABBA Gold",
},
};
// Only change code below this line
function updateRecords(records, id, prop, value) {
return records;
}
updateRecords(recordCollection, 5439, "artist", "ABBA");
To solve this challenge, you need to update the updateRecords()
function given above.
You need to understand JavaScript conditional if..else
block and hasOwnProperty()
object method
Challenge solution
The first thing you need to do here is to check on the value of the value
parameter passed to the function.
If the value
parameter is an empty string, you delete the prop
of the object with the same id
value passed as an argument to the function.
Here’s how you do it:
function updateRecords(records, id, prop, value) {
if (value === "") {
delete records[id][prop];
}
return records;
}
In the above code, the bracket notation []
is used to access the object’s properties ([id]
and [prop]
)
Next, you need to check if the prop
variable value is equal to tracks
or not.
If it’s not, then you just add the prop
as a new property to the object as follows:
function updateRecords(records, id, prop, value) {
if (value === "") {
delete records[id][prop];
} else if (prop !== "tracks") {
records[id][prop] = value;
}
return records;
}
When the prop
value equals the string tracks
, you need to check if the record with the same id
value has the tracks
property.
When the object has the tracks
property, add the value
to the tracks
array using the push()
method:
function updateRecords(records, id, prop, value) {
if (value === "") {
delete records[id][prop];
} else if (prop !== "tracks") {
records[id][prop] = value;
} else {
if (records[id].hasOwnProperty("tracks")) {
records[id].tracks.push(value);
}
}
return records;
}
Finally, you need to create a new tracks
property to the object when it doesn’t have one as follows:
function updateRecords(records, id, prop, value) {
if (value === "") {
delete records[id][prop];
} else if (prop !== "tracks") {
records[id][prop] = value;
} else {
if (records[id].hasOwnProperty("tracks")) {
records[id].tracks.push(value);
} else {
records[id].tracks = [];
records[id].tracks.push(value);
}
}
return records;
}
With that, the updateRecords()
function is now finished.
Run the tests to see your code pass all presented scenarios.
Congratulations on passing the challenge! 😉 This challenge should improve your understanding of JavaScript control flow with if..else
block and manipulating an object.