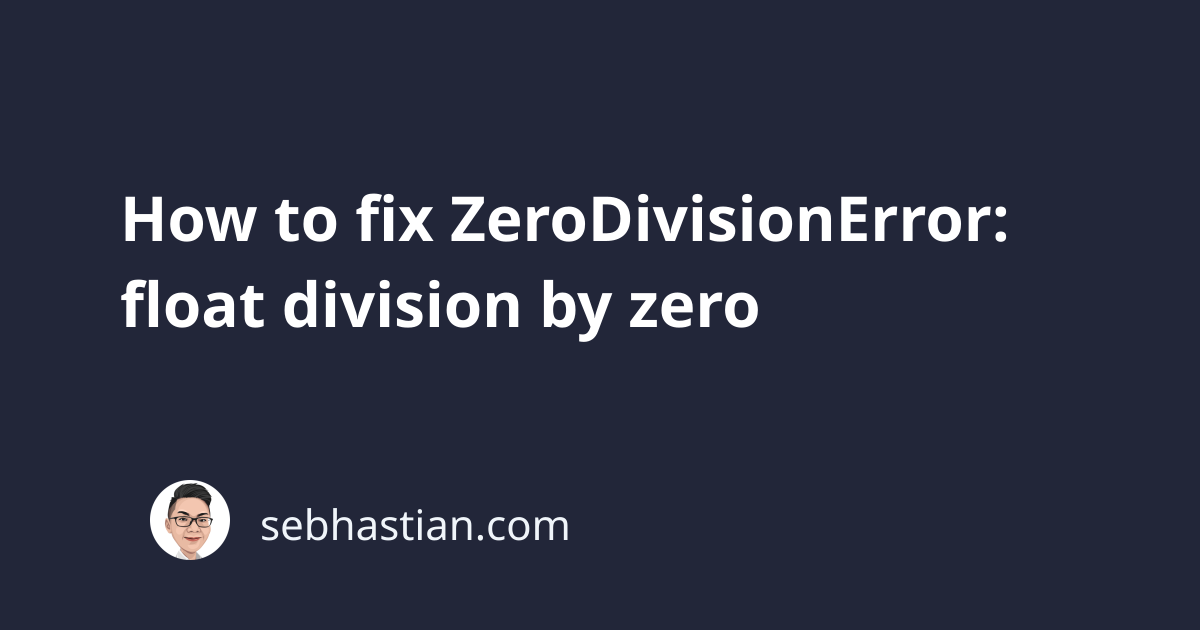
When working with numbers in Python, you might encounter the following error:
ZeroDivisionError: float division by zero
This error occurs when you attempt to divide a floating number with zero.
Python raises the ZeroDivisionError
because dividing a number with a zero returns an infinite number, which is impossible to measure in a programming language.
This tutorial will show you an example that causes this error and how to fix it
How to reproduce this error
Suppose you have two number variables where one of them is a float and the other is zero.
When you divide the float by zero as follows:
x = 9.5
y = 0
z = x / y
You get this output:
Traceback (most recent call last):
File "main.py", line 4, in <module>
z = x / y
ZeroDivisionError: float division by zero
The error occurs because the y
variable is zero, so the division yields an infinite number that can’t be counted.
How to fix this error
To resolve this error, you need to prevent a division by zero from happening in your code.
One way to do this is to use the if
statement to check if the dividing number is zero. In this case, you have to check the value of the y
variable:
x = 9.5
y = 0
if y == 0:
print("Can't perform division: the y variable is 0")
z = 0
else:
z = x / y
Or you can also use a one-line if
statement as follows:
z = 0 if y == 0 else (x / y)
When the value of y
is zero, then set the value of z
as zero too. Otherwise, divide x
by y
and assign the result to z
.
Other similar errors
Besides float division by zero, the ZeroDivisionError
also has some alternatives:
ZeroDivisionError: integer division by zero
ZeroDivisionError: integer modulo by zero
ZeroDivisionError: float modulo by zero
Although the error message is slightly different, all these variants are caused by the same problem: you tried to divide or modulo the number by zero.
Here are some examples:
x = 5 / 0 # integer division by zero
y = 5 % 0 # integer modulo by zero
z = 7.5 % 0 # float modulo by zero
The solution to these errors is the same, you need to prevent the numbers from being divided or reduced using a zero.
I hope this tutorial helps you solve the error. Cheers! 🙌