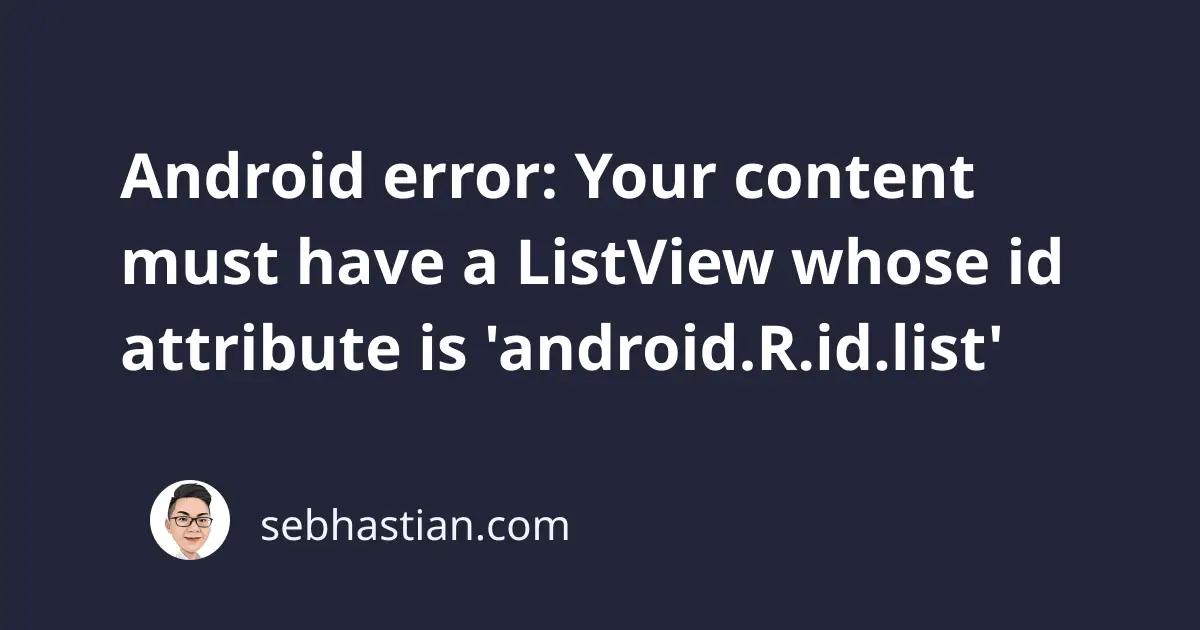
When you create a ListActivity
class to host a ListView
object, Android may complain about the lack of the id
attribute for that ListView
.
For example, suppose you have a MainActivity
class as follows:
public class MainActivity extends ListActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
}
Then, you create the following activity_main.xml
layout:
<ListView
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/list" >
</ListView>
When you build and run the Android application, a RuntimeException
error will occur with the following message:
E/AndroidRuntime: FATAL EXCEPTION: main
# ...
Caused by: java.lang.RuntimeException:
Your content must have a ListView whose id attribute is 'android.R.id.list'
at #...
The exception above happens because the ListActivity
class specifically requires a ListView
object that has an id
attribute of @android:id/list
.
The value @+id/list
resolves to R.id.list
while @android:id/list
resolves to android.R.id.list
.
To resolve the error, you need to change the android:id
attribute in your ListView
widget as shown below:
<ListView
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@android:id/list" >
</ListView>
Rebuild your application and run it again. This time, the error should disappear.
Also, please note that the ListActivity
class has been deprecated in API level 30 (Android 11)
When you need to display a UI component with a list of items, you are recommended to use either ListFragment
or RecyclerView
instead.
Now you’ve learned how to resolve the missing android.R.id.list
id attribute in ListActivity
class. Good work! 👍