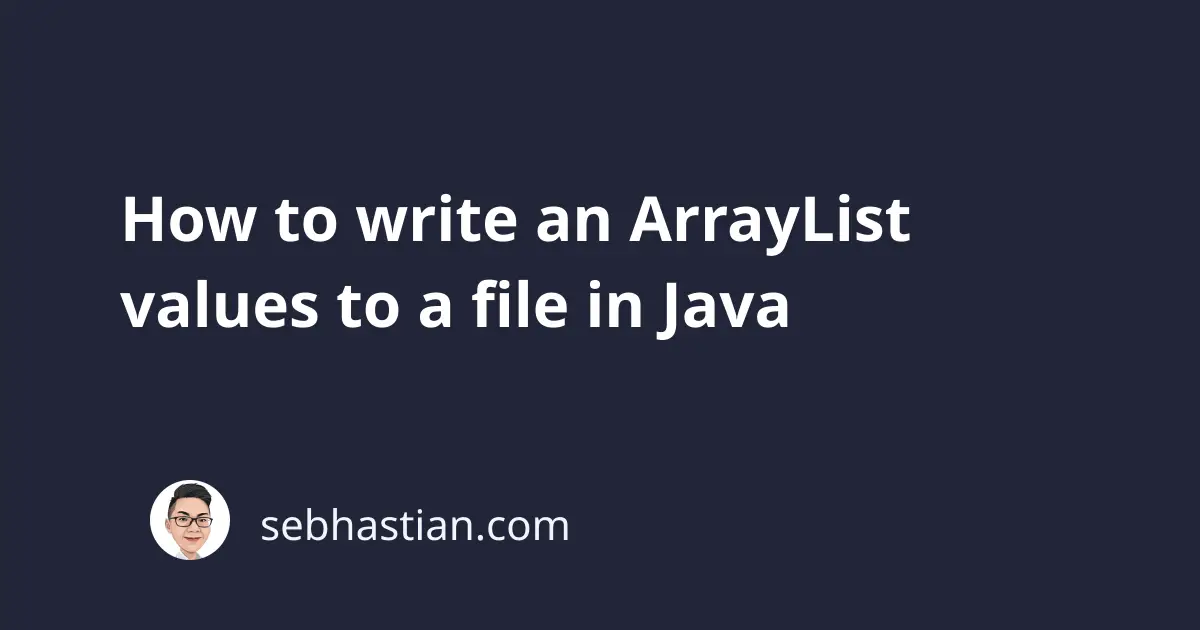
You can write an ArrayList
object values to a plain text file in Java by using the built-in java.nio.file
package.
First, create your ArrayList
object and add some values to the list as shown below:
List arrList = new ArrayList<String>();
arrList.add("Java");
arrList.add("Programming");
arrList.add("Language");
Next, use the Paths
class to get()
the file path using a String
as follows:
Path output = Paths.get("output.txt");
The Path
object represents a path to the file in your system.
After that, you need to call the write()
method from the Files
class to write the arrList
variable values to the output
path.
You need to surround the write()
method in a try...catch
block to handle any exception that might occur when writing the values to the file:
try {
Files.write(output, arrList);
} catch (Exception e) {
e.printStackTrace();
}
Once finished, you should see the output.txt
generated by JVM in the current working directory of your Java project.
To find the location of the file, you can call the toFile().getAbsolutePath()
method from the Path
object.
Add a println()
method call just below the Files.write()
line as shown below:
try {
Files.write(output, arrList);
System.out.println(
output.toFile().getAbsolutePath()
);
} catch (Exception e) {
e.printStackTrace();
}
You can open the printed path to see the output.txt
file.
The text file content should be as follows:
Java
Programming
Language
When you want the file to be generated in a different location, you can provide an absolute path as a parameter to the Paths.get()
method.
For example, I’d like the file to be generated on my Desktop
directory, so I specified the absolute path to the directory below:
public static void main(String[] args) {
List arrList = new ArrayList<String>();
arrList.add("Java");
arrList.add("Programming");
arrList.add("Language");
Path output = Paths.get("/Users/nsebhastian/Desktop/output.txt");
try {
Files.write(output, arrList);
System.out.println(output.toFile().getAbsolutePath());
} catch (Exception e) {
e.printStackTrace();
}
}
With that, the file will be generated in the Desktop/
directory as output.txt
file.
Now you’ve learned how to write an ArrayList
values to a file using Java. Good work! 👍