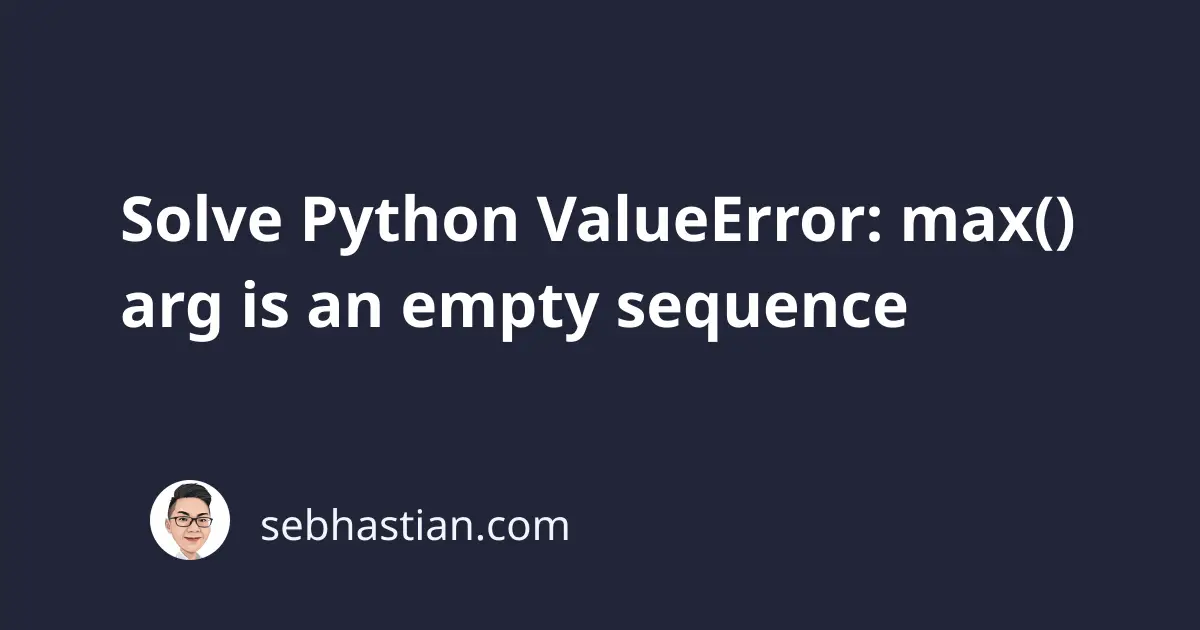
The Python exception “ValueError: max() arg is an empty sequence” happens when you call the max()
function while passing an empty sequence as its parameter.
You need to pass a non-empty sequence to solve this error. Read this tutorial to see how.
The max()
function is a built-in function in Python that returns the largest element in a sequence as shown below:
result = max(100, 20, 55, 79)
print(result) # 100
However, if you try to call max()
on an empty sequence, the function has nothing to compare and Python will raise the “ValueError: max() arg is an empty sequence” exception.
Suppose you run the following code:
list = []
result = max(list)
The output will be:
To fix this error, you need to make sure that the sequence passed to max()
has at least one value.
You can do this by checking the length of the sequence before calling max()
, or by using a default value for the sequence if it is empty.
Consider the code below:
list = []
if len(list) > 0:
result = max(list)
else:
result = 0
Using an if .. else
block, you can make Python calls the max()
function only when the list
length is greater than 0
.
Alternatively, you can pass the default
argument to the max()
function like this:
list = []
# Pass a default value to max()
result = max(list, default= 20)
print(result) # 20
When you pass a default
argument to the max()
function, Python will return that value instead of raising a ValueError
exception.
Finally, you can also add a try .. except
block to handle the error as shown below:
list = []
try:
result = max(list)
except ValueError:
print("An error occurred")
result = 100
print(result) # 100
In the code above, Python will run the except
block when the code in the try
block fails to execute properly.
You can modify the code in the except
block to fit your requirements.
Conclusion
To fix the Python exception ValueError: max() arg is an empty sequence, you need to pass a non-empty sequence when calling the max()
function.
You can also use an if .. else
or try .. except
block to let Python know what to do when the ValueError exception happens.
Also, this exception may also happen when you run the min()
function.
I hope this tutorial helps you become better with Python. 🙏