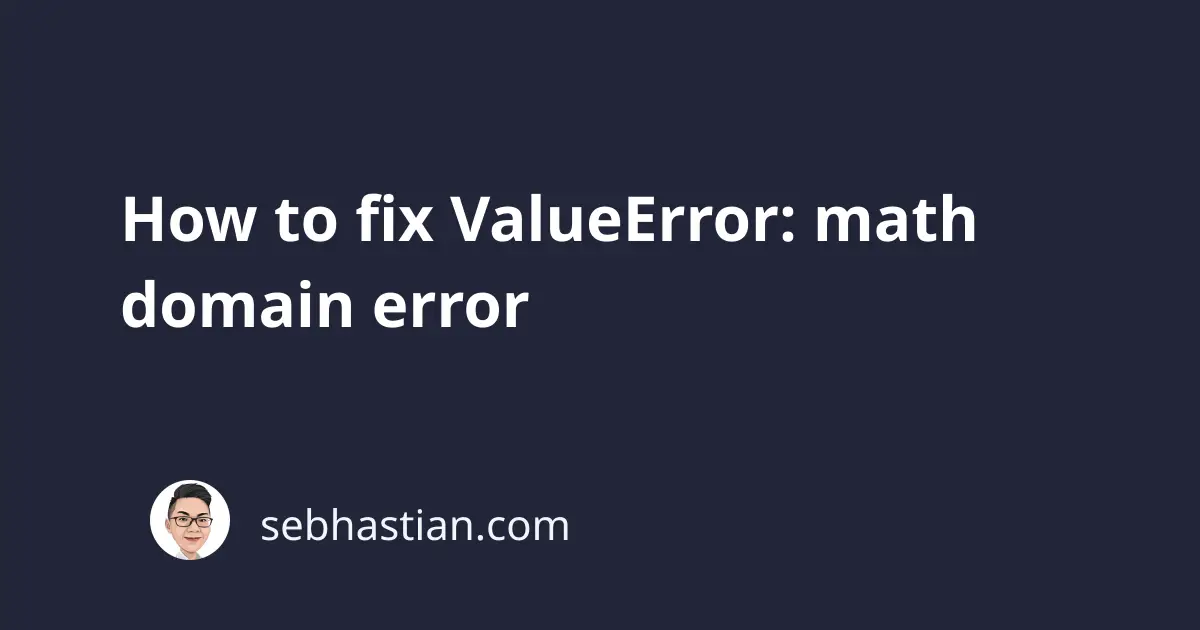
When working with the math
module in Python, you might encounter the following error:
ValueError: math domain error
This error usually occurs when you pass a number to one of the math
module functions that can’t process zero or negative numbers.
Some common scenarios that cause this error are:
- Passing a negative number to the
sqrt()
function - Passing zero or a negative number to the
log()
function
The following tutorial shows examples for each scenario and how to fix it
1. You passed a negative number to the sqrt()
function
The math.sqrt()
function is unable to calculate the square root of negative numbers.
Suppose you want to find the square root of -2
as follows:
import math
x = math.sqrt(-2)
Output:
Traceback (most recent call last):
File "main.py", line 3, in <module>
x = math.sqrt(-2)
ValueError: math domain error
To resolve this error, you need to make sure you’re passing only positive numbers to the sqrt()
function.
1. You passed zero or a negative number to the log()
function
The log()
function of the math
module can’t process a 0
or negative number.
So when you try to find the log of 0
as follows:
import math
x = math.log(0)
You’ll get this error:
Traceback (most recent call last):
File "main.py", line 3, in <module>
x = math.log(0)
ValueError: math domain error
To resolve this error, you need to ensure that you’re only using positive numbers with the log()
function.
Here’s an example of one that works:
import math
x = math.log(5)
print(x) # 1.6094379124341003
Notice that we received no error this time.
Conclusion
The “math domain error” occurs because you’re passing numbers that can’t be computed by the math
module functions.
To resolve this error, you need to make sure that you’re passing numbers that are valid for the function you called.
I hope this tutorial is helpful. Happy coding! 👋