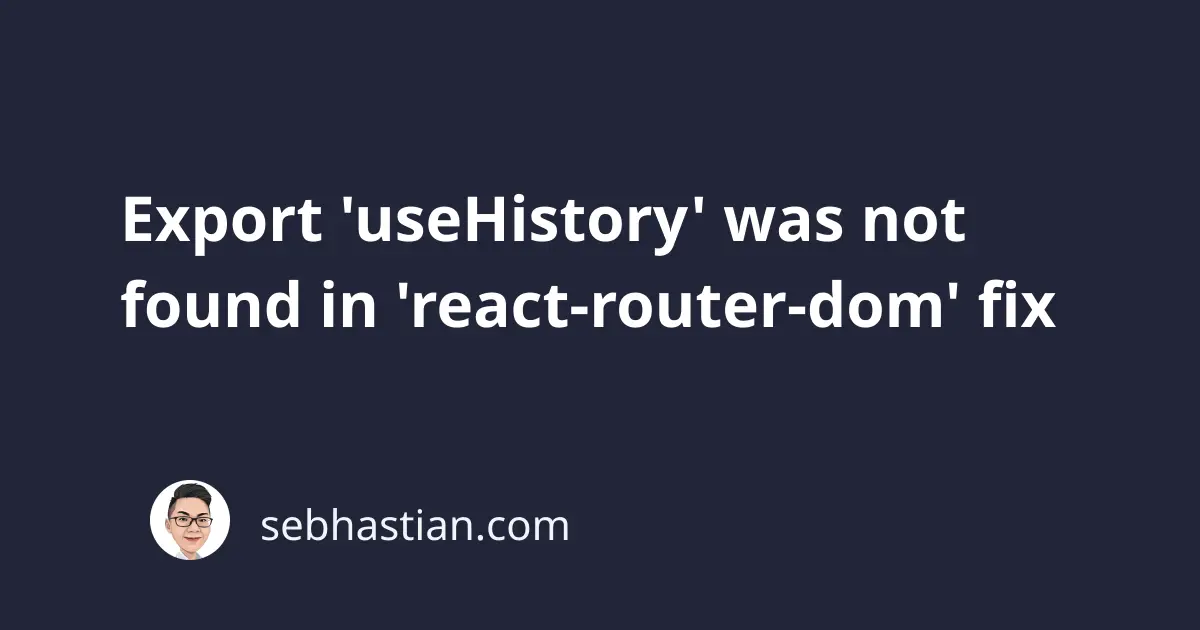
If you use React Router in your React application, you might encounter this error:
export 'useHistory' was not found in 'react-router-dom'
This error occurs because the useHistory
hook has been deprecated and removed in React Router version 6.
To fix this error, you need to replace useHistory
with the useNavigate
hook.
The useNavigate
hook is introduced in React Router version 6 to provide better compatibility with React apps that enable suspense mode.
How to reproduce this error
Suppose you upgraded your react-router-dom
package to version 6, then you run the following code:
import { useHistory } from "react-router-dom";
function App() {
const history = useHistory();
return <div>Hello World!</div>;
}
The line where you called the useHistory
hook will cause the following error:
ERROR in ./src/App.js
export 'useHistory' (imported as 'useHistory') was not found in 'react-router-dom'
To fix this error, you need to replace all occurrences of useHistory
with useNavigate
as follows:
import { useNavigate } from "react-router-dom";
function App() {
const navigate = useNavigate();
return <div>Hello World!</div>;
}
Of course, there are also differences in how the useNavigate
hook works compared to useHistory
.
For example, the history.push()
method has been replaced with navigate()
as follows:
import { useNavigate } from "react-router-dom";
function App() {
let navigate = useNavigate();
function handleClick() {
// previously
// history.push("/store");
navigate("/store");
}
return (
<div>
<button onClick={handleClick}>Store</button>
</div>
);
}
If you want to replace the current location instead of pushing a new one into the history, you need to use navigate(to, { replace: true })
like this:
import { useNavigate } from "react-router-dom";
function App() {
let navigate = useNavigate();
function handleClick() {
navigate("/store", { replace: true });
}
return (
<div>
<button onClick={handleClick}>Store</button>
</div>
);
}
The replace
config replaces the current entry in the history stack, preventing the user from going back to the page using the back button in the browser.
If you use the declarative API from useHistory
such as <Redirect>
, you need to replace it with <Navigate>
as follows:
// Change this:
<Redirect to="store" />
<Redirect to="home" push />
// to this:
<Navigate to="store" replace />
<Navigate to="home" />
The <Redirect>
component uses replace
logic by default, while <Navigate>
uses push logic.
If you want to use the replace
logic with the <Navigate>
component, you need to add the replace
prop.
The go
, goBack
and goForward
functions have also been replace with the navigate()
function.
To goBack
, you need to pass -1
as the argument to navigate
. To goForward
, pass 1
instead.
Here are some examples:
import { useNavigate } from "react-router-dom";
function App() {
const navigate = useNavigate();
return (
<>
<button onClick={() => navigate(-1)}>Go back</button>
<button onClick={() => navigate(1)}>Go forward</button>
<button onClick={() => navigate(-2)}>Go 2 pages back</button>
<button onClick={() => navigate(2)}>Go 2 pages forward</button>
</>
);
}
The navigate()
function can replace the functionalities of go
, goBack
and goForward
so they have been removed in React Router version 6.
Now you’ve learned how to fix “Export ‘useHistory’ was not found in ‘react-router-dom’” error. Happy coding! 👍