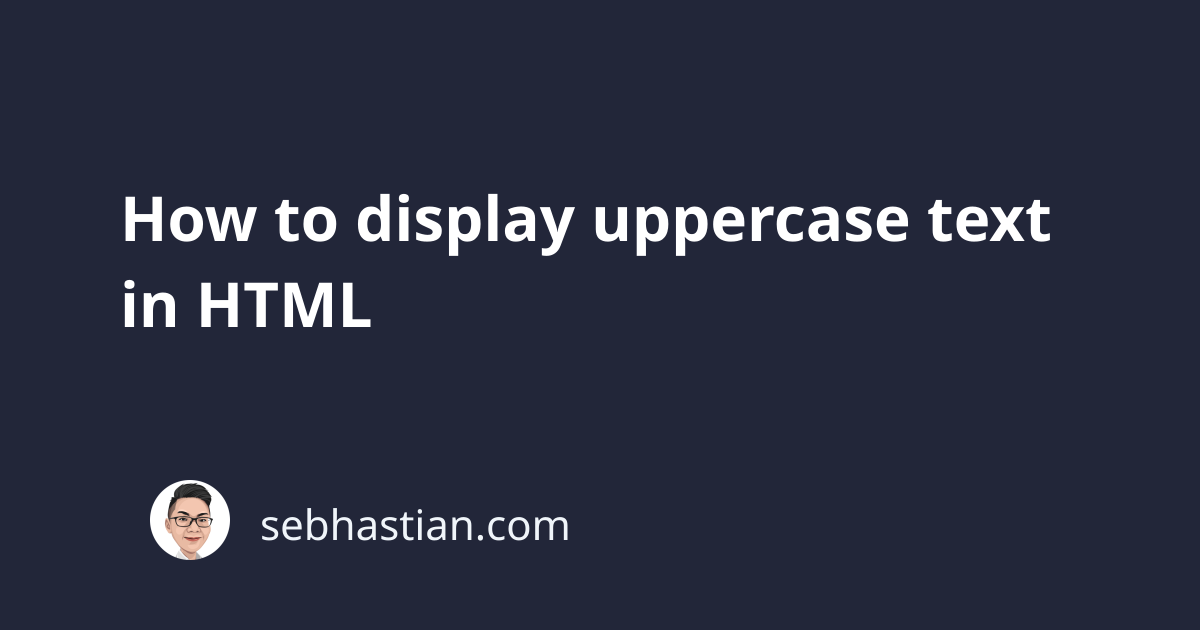
You can use uppercase letters directly inside your HTML text content without using any tricks as shown below:
<p>HELLO WORLD! THESE ARE UPPERCASE LETTERS</p>
Since HTML itself is case-insensitive, you can even write your HTML tags using uppercase letters:
<div>THIS IS PERFECTLY VALID HTML</div>
But uppercase letters are bad for readability, and there’s a non-spoken agreement between web developers to always use lowercase for HTML tags and content.
This is why if you want to use uppercase letters – and keep your friends from YELLING at you – I’d recommend that you use the CSS text-transform
style property.
With the text-transform
property, you can style your text content as all lowercase
, all uppercase
, or capitalize
only the first letter of each word.
The following example:
<body>
<div style="text-transform:uppercase;">hello world (upper)</div>
<div style="text-transform:lowercase;">happy friday (lower)</div>
<div style="text-transform:capitalize;">
it's a nice day to code (capitalize)
</div>
</body>
Will produce the following output:
When you need to uppercase only a certain word inside a <div>
or a <p>
element, you need to wrap the word using the <span>
tag and apply the text-transform:uppercase
style to that <span>
element:
<body>
<p>
you can <span style="text-transform:uppercase;">uppercase</span> a word
</p>
<p>
Up to <span style="text-transform:uppercase;">as many words</span> as you
want
</p>
</body>
You can include as many words as you need to uppercase inside the <span>
tag.
Uppercase for input elements
You can also render the value inside an <input>
element using uppercase letters with the text-transform
property as shown below:
<body>
<form method="GET">
<label for="username">Username:</label>
<input type="text" id="username" name="username"
style="text-transform:uppercase;" />
<input type="submit" />
</form>
</body>
But using the text-transform
style does not actually change the input values to uppercase. When you submit the form, the input value will still use the case as the text was typed by the user.
Using the above example, you can see that the GET
method ?username
value will use the letter case that you typed into the <input>
element.
To guard your <input>
element against accepting lowercase letters, you can add the following pattern
attribute:
<body>
<form method="GET">
<label for="username">Username:</label>
<input type="text" id="username" name="username"
pattern="[A-Z]*" title="Only uppercase letters" />
<input type="submit" />
</form>
</body>
The title
attribute above will be used as the validation message when you click on the submit button as shown below:
Although you can force the user to use only uppercase letters, it’s recommended to re-validate the form inputs in your backend server because the HTML markup can be accessed from the browser HTML inspector.
A sophisticated Internet user can access your HTML code from the inspector and change the attributes, which allows that user to submit lowercase letters.
If you’re using JavaScript for your server, you can easily transform the text to uppercase using the toUpperCase()
method.
let username = "nathan";
let newUsername = username.toUpperCase();
console.log(newUsername); // "NATHAN"
Another language like PHP has the strtoupper()
method and Python has the upper()
method.
You need to find the right method to transform the string to uppercase using your backend language.