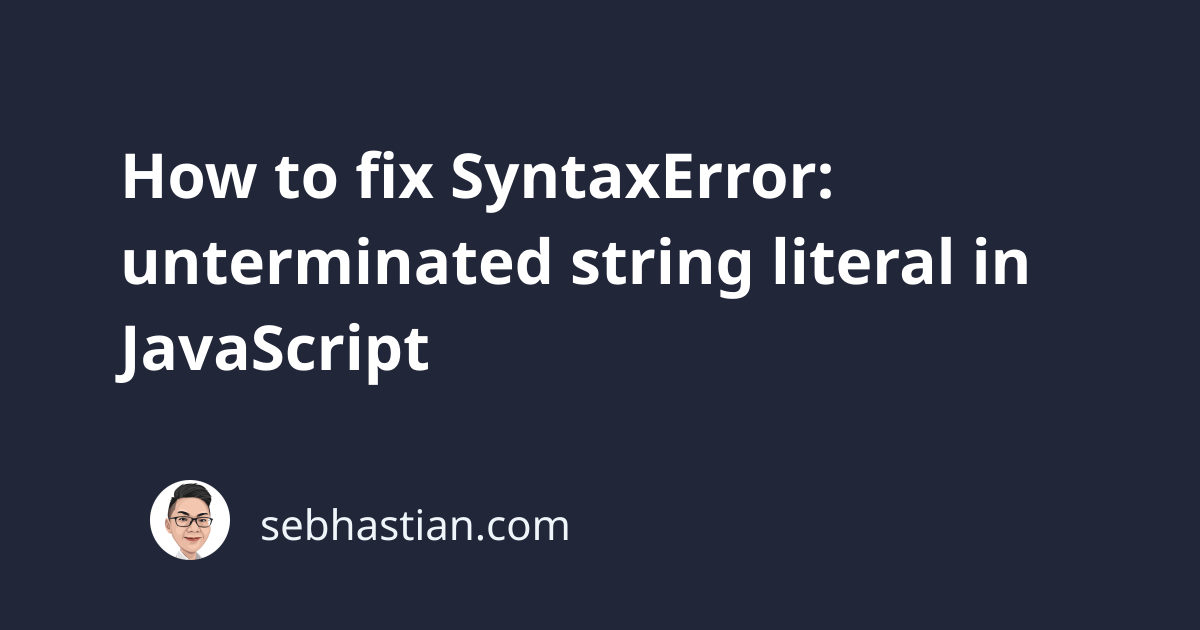
The SyntaxError: unterminated string literal occurs in JavaScript when you have a string value that’s not ended properly.
Other names for this error include:
- SyntaxError: Unterminated string constant
- SyntaxError: "" string literal contains an unescaped line break
When creating a string, you need to enclose the string in single or double quotations as follows:
console.log("Hello World!");
// or
console.log('Hello World!');
The error occurs when you didn’t add a closing quote symbol to the string as shown below:
// Error
console.log('Hello World!);
// Error
console.log('Hello World!");
To resolve this error, you need to make sure that you enclose any string value you have with the right quotation mark.
This error might also occur when you have a multi-line string as follows:
let myString = "Hello, this is a very long string which needs
to wrap across multiple lines to make it
easier to read.";
The code above causes the error because JavaScript can’t read multi-line strings wrapped in quotations.
To fix the error, you need to close the string at each line, then concatenate them together using the +
operator:
let myString = "Hello, this is a very long string which needs " +
"to wrap across multiple lines to make it " +
"easier to read.";
Here, the long string is joined together with the +
operator.
Alternatively, you can also use the backslash \
character when you put a part of a string to the next line as follows:
let myString = "Hello, this is a very long string which needs\
to wrap across multiple lines to make it\
easier to read.";
Note that you need to mind the whitespaces in the next line, because any space you specify there will be included in the string.
The third option is to use the backtick `
symbol to wrap the string as follows:
let myString = `Hello, this is a very long string which needs
to wrap across multiple lines to make it
easier to read.`;
Just like when you use the backslash, you need to mind the whitespaces when using backticks to create a multi-line string.
And that’s how you resolve the SyntaxError: unterminated string literal in JavaScript. Happy coding! 🙌