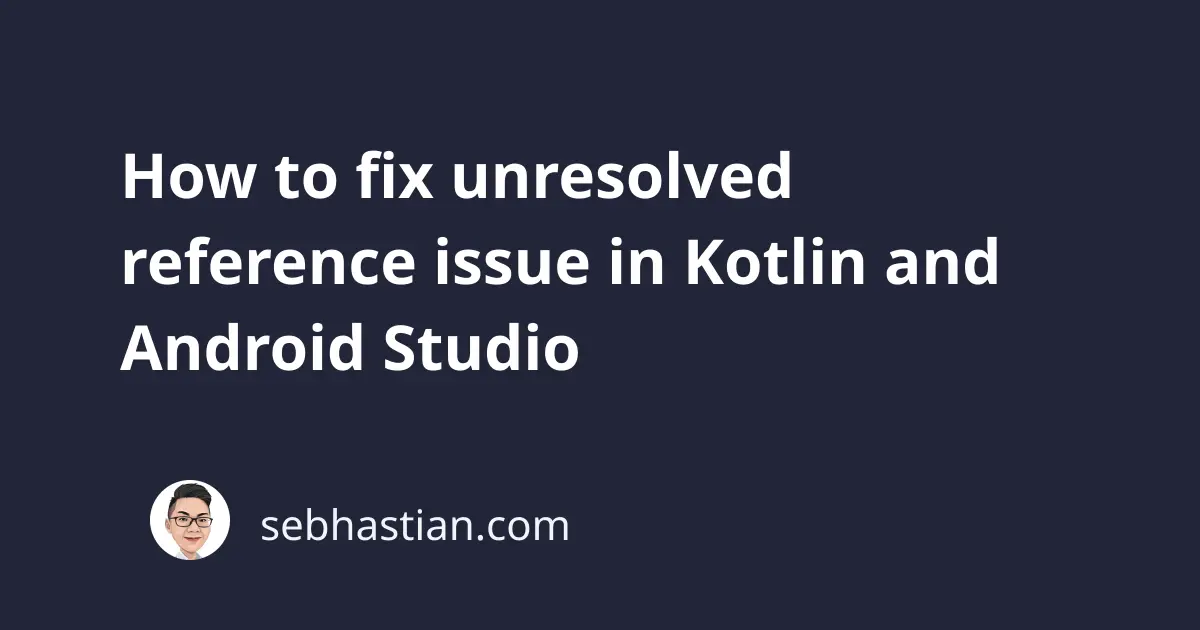
When you’re writing code for Android application and Kotlin, you may frequently encounter a static error from your IDE saying unresolved reference
for a specific keyword or variable.
For example, you can produce the error by calling a custom function that you haven’t defined in your code yet:
myFunction("Hello")
// Unresolved reference: myFunction
Or when you assign a variable without declaring them:
myStr = "Hello"
// Unresolved reference: myStr
To resolve the issue, you need to make sure that the referenced keyword (whether it’s a variable, a function, or an object) is available and defined before it’s called.
To declare a variable, use the val
or var
keyword. To declare a function, use the fun
keyword:
val myStr = "Hello"
fun myFunction(str: String) = print(str)
myFunction(myStr)
// no issues
When coding an Android application, this error may happen when you import libraries that haven’t been added to your project yet.
For example, you may import the kotlinx
library in your MainActivity.kt
file as follows:
package com.example.myapplication
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import kotlinx.android.synthetic.main.activity_main.*
Without adding the kotlin-android-extensions
in your build.gradle
file, the import kotlinx
line above will trigger the unresolved reference
issue.
To resolve it, you need to add the kotlin-android-extensions
to the build.gradle
file plugins
list as shown below:
plugins {
id 'com.android.application'
id 'kotlin-android'
id 'kotlin-android-extensions'
}
Please make sure that you add the id 'kotlin-android-extensions'
line on the build.gradle
file that’s inside your app
module.
Once you sync the Gradle build, the error should disappear.
If you still see the unresolved reference
error after fixing the problem, try to build your Android application with Command + F9
for Mac or Control + F9
for Windows and Linux.
The error should disappear after the build is completed.
To conclude, the unresolved reference
error happens when Kotlin has no idea what the keyword you’re typing in the file points to.
It may be a function, a variable, or another construct of the language that you’ve yet to declare in the code.
Or it can also be external Android and Kotlin libraries that you haven’t added to your current project yet.
For example, you may encounter an error saying Unresolved reference: Volley
as follows:
val queue = Volley.newRequestQueue(this)
// Unresolved reference: Volley
This is because the Volley
library hasn’t been added to the Android project yet.
If you click on the red bulb icon on the code line with the issue, Android Studio has several suggestions to resolve the error, one of them helps you to import the dependency automatically:
In this case, you can simply click on the highlighted option above and Android Studio will resolve the problem for you.
But other libraries may not have such an option, so you need to import them manually in the build.gradle
file of your app
module.
Once you specify the import, don’t forget to sync the Gradle build again to download the library into your project.
Good luck on fixing the unresolved reference issue! 👍