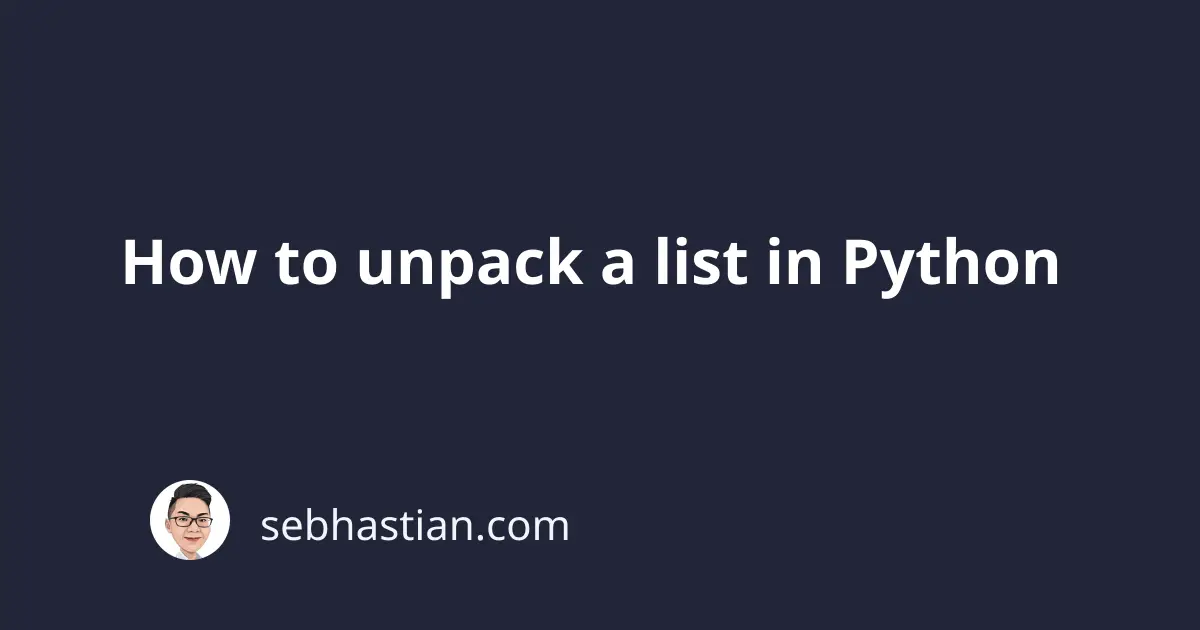
The unpacking assignment is a feature of Python language that allows you to assign elements of a sequence to variables without needing to access the elements one by one using index notation.
For example, suppose you have a list of strings as follows:
animals = ['dog', 'cat', 'lion']
Suppose you assign the list elements to variables one by one using the index notation:
dog = animals[0]
cat = animals[1]
lion = animals[2]
While this is valid, you can avoid accessing the elements repeatedly by using the unpacking assignment.
To use the unpacking assignment, simply provide the variable names on the left hand of the =
operator as follows:
dog, cat, lion = animals
print(dog) # dog
print(cat) # cat
print(lion) # lion
As you can see, the unpacking assignment assigns the first, second, and third elements to the variables in the same order as you define them.
The unpacking assignment is also flexible enough so you can discard the elements you don’t need or assign the rest as a new list.
Unpack and discard elements you don’t need
To discard the elements you don’t need, you can define _
as the variable to receive the elements you want to discard.
For example, suppose you only need the first two elements from a list:
animals = ['dog', 'cat', 'lion', 'wolf']
dog, cat, _, _ = animals
In the above example, the third and fourth elements will be assigned to the underscore variable, so you can ignore it altogether.
Note that you always need to unpack all elements in the list. You can’t choose to unpack only the first two by assigning two variables like this:
animals = ['dog', 'cat', 'lion', 'wolf']
dog, cat = animals # ❌
Python will complain with ValueError: too many values to unpack
if you ignore the elements as shown above.
This is why the underscore variable is useful when you only unpack a portion of the list.
Unpack elements as a new list
You can also unpack elements from a list as a new list by using the asterisk *
operator.
For example, suppose you only want to unpack the first element and keep the rest separate as its own list. Here’s how you do it:
animals = ['dog', 'cat', 'lion', 'wolf']
dog, *others = animals
print(dog) # dog
print(others) # ['cat', 'lion', 'wolf']
The asterisk in front of the *others
variable allows that variable to accept a number of elements as a list.
Here’s another example where you unpack the first two elements and pack the rest:
animals = ['dog', 'cat', 'lion', 'wolf']
dog, cat, *others = animals
print(dog) # dog
print(cat) # cat
print(others) # ['lion', 'wolf']
The asterisk operator is also useful when you want to discard the rest of the elements.
You can specify *_
to avoid defining _
repeatedly:
animals = ['dog', 'cat', 'lion', 'wolf']
dog, *_ = animals
print(dog) # dog
print(_) # ['cat', 'lion', 'wolf']
After the first element dog
, the rest is packed in the _
variable, which you can ignore.
Conclusion
The unpacking assignment is a convenient way to extract elements of a list in Python. This method helps you to assign certain elements of a list according to the requirements of your program.
The unpacking assignment also works with all Python sequence types, such as a tuple, a string, or a range.
Now you’ve learned how to unpack a list like a professional Python developer. Nice work! 👍