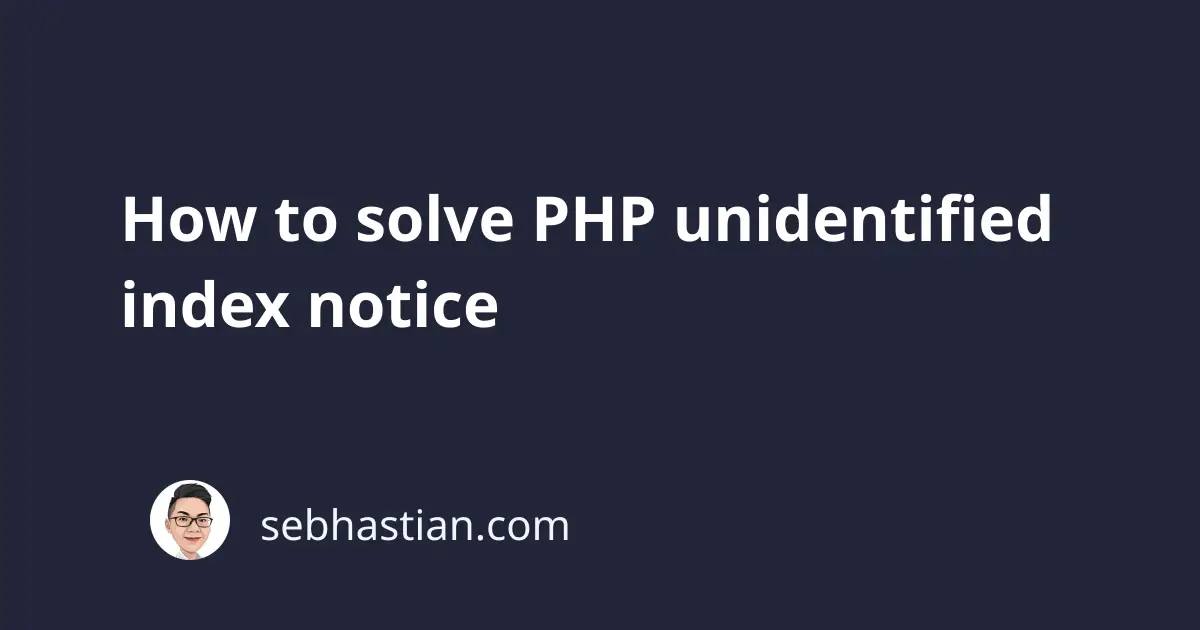
The unidentified index notice in PHP appears when you try to access an array variable with a key that doesn’t exist.
For example, suppose you have an associative array named $user
with the following values:
<?php
$user = [
"name" => "Nathan",
"age" => 28,
"hobby" => "programming",
];
Suppose you try to access the $user
variable with the key user_id
.
Because the $user
variable doesn’t have a user_id
key, PHP will respond with the unidentified index notice:
<?php
print $user["user_id"];
The code above will produce the following output:
Notice: Undefined index: user_id in ... on line ...
The notice above means PHP doesn’t know what you mean with user_id
index in the code.
To solve this issue, you need to make sure that the array key exists by calling the isset()
function:
<?php
$user = [
"name" => "Nathan",
"age" => 28,
"hobby" => "programming",
];
if (isset($user["user_id"])) {
print $user["user_id"];
} else {
print "user_id does not exists";
}
A fellow once asked me, “isn’t it enough to put the variable inside an if
statement without isset()
?”
Without the isset()
function, PHP will still emit the “undefined index” notice.
You need both the isset()
function and the if
statement to remove the notice.
This issue frequently appears when you are accessing data from the $_POST
or $_GET
variable.
The solution also works for these global variables:
// 👇 check if the name variable exists in $_POST
if (isset($_POST["name"])) {
print $_POST["name"];
}
// 👇 check if the query variable exists in $_GET
if (isset($_GET["query"])) {
print $_GET["query"];
}
If you are assigning the value to a variable, you can use the ternary operator to assign a default value to that variable.
Consider the example below:
// assign name from $_POST into $name
// otherwise, put User0 to $name
$name = isset($_POST["name"]) ? $_POST["name"] : "User0";
The ternary operator allows you to write a shorter code for the if..else
check.
Now you’ve learned how to fix the unidentified index notice in PHP. Good work! 👍