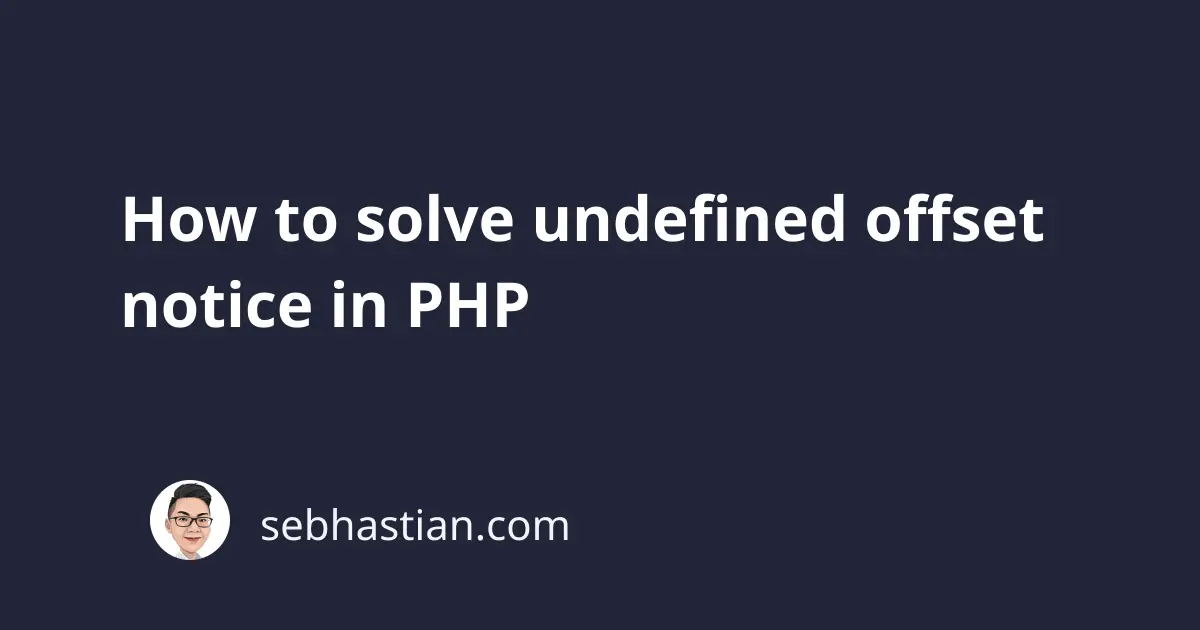
The PHP “undefined offset” notice occurs when you try to access an array element that doesn’t exist.
For example, suppose you have a non-associative array with one element as shown below:
<?php
$arr = ["Jack"];
print $arr[0]; // Jack
print $arr[1]; // Notice: Undefined offset
When you try to access an element using its number key, PHP will check if the key is defined in your array.
In the above example, the key [1]
doesn’t exist in the $arr
variable, which is why PHP responded with “Notice: Undefined offset” message.
To fix this message, you need to make sure that you are accessing an array element that exists in your array.
You can write an if
statement that checks whether the array key and element exist using the isset()
function as shown below:
<?php
$arr = ["Jack"];
if (isset($arr[1])) {
print $arr[1];
} else {
print "Array key [1] is undefined";
}
// Output: Array key [1] is undefined
By calling the isset()
function to check on the $arr[1]
variable, the “undefined offset” message won’t be shown.
The else
statement allows PHP to gracefully notifies you that the array key is undefined.
Now you’ve learned how to solve the undefined offset message in PHP. Good work!