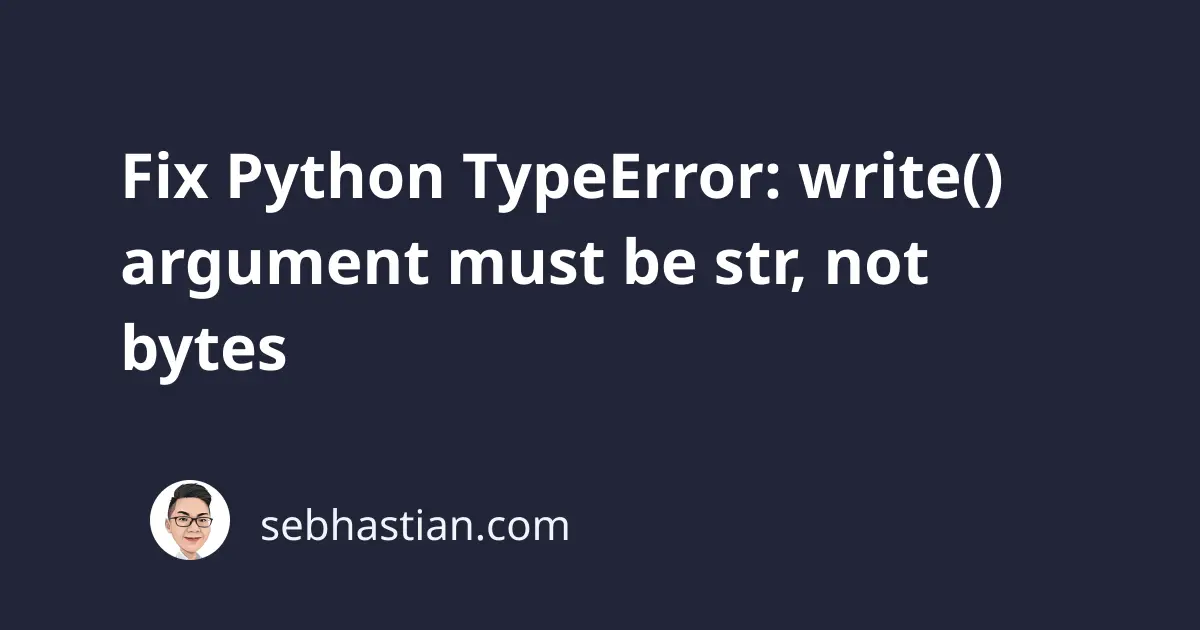
Python shows the error message “TypeError: write() argument must be str, not bytes” when you are attempting to write bytes to a file object that is expecting a string.
To write bytes to a file, you need to open the file in binary mode by passing wb
as the second argument to the open()
function.
Suppose you try to write bytes to a file called output.txt
as shown below:
with open('output.txt', 'w') as file_obj:
file_obj.write(b'Python is awesome') # ❗️TypeError
Because you are opening the file in write mode, Python will respond with the TypeError as shown below:
To fix this error, you need to pass wb
instead of w
as the mode of the operation when calling the open()
function.
This allows you to write bytes object directly to the file:
with open('output.txt', 'wb') as file_obj:
file_obj.write(b'Python is awesome') # ✅
With the wb
mode, Python will be able to write the bytes object passed as the argument to the write()
method above.
Alternatively, you can also convert the bytes object into a string using the decode()
method.
See the example below:
bytes_data = b'Python is awesome'
# 👇 Convert bytes to string
str_data = bytes_data.decode('utf-8')
# 👇 write string to file
with open('output.txt', 'w') as file_obj:
file_obj.write(str_data)
To conclude, the message TypeError: write() argument must be str, not bytes occurs in Python when you try to write bytes to a file object that’s opened in w
mode.
The w
mode only accepts a string as input, so if you want to write bytes to the file, you need to open the file in wb
mode.
Alternatively, you can also convert the bytes object to a string using the decode()
method.
By following the examples in this article, you can successfully write bytes data to a file object or stream in Python.