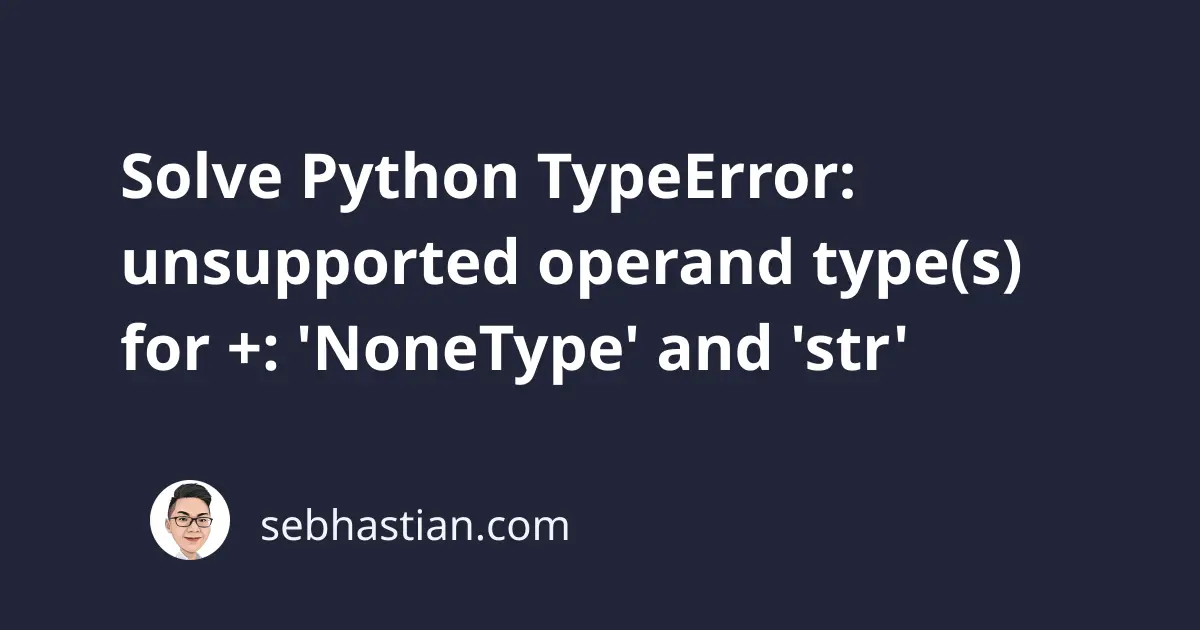
The Python TypeError: unsupported operand type(s) for +: ‘NoneType’ and ‘str’ happens when you try to concatenate a None
value with a string value.
To solve this error, make sure you are not concatenating a None
value with a string value. See this tutorial for more info.
In Python, the NoneType
data type represents the absence of a value or a null value.
You can initiate the NoneType
object using the None
keyword as follows:
# This will assign the None value to the x variable
x = None
You can also use the None
value to indicate that a function does not return a value.
For example:
def my_function():
return None # return the None value
Python allows you to concatenate (or merge) two or more string values into one string using the addition +
operator.
But when you merge a NoneType
with a string, Python will raise a TypeError. The following code:
result = None + "Morning!"
Gives the following output:
One common situation where this TypeError can occur is when using the print()
function in Python.
In Python version 2, print
is a statement so you can do concatenation like this:
# Valid in Python v2
print ("Hello ") + "World!" # Hello World!
But in Python v3, print()
is a function that returns None
.
This is why concatenating outside of the parentheses results in TypeError:
# Error in Python v3
print ("Hello ") + "World!" # ❗️TypeError
To fix this issue, you need to do the concatenation inside the parentheses as shown below:
print ("Hello " + "World!") # ✅
print ("Hello", "World!") # ✅
The print()
function is one source of the None
value in Python3, so make sure you avoid concatenating outside the parentheses.
Other cases when None causes TypeError
Another way you can have None
is when your function doesn’t return any value:
def get_name():
x = input("Enter your name: ")
if x: # If x is not an empty string
return x # Return the value of x
y = " is your name"
z = get_name() + y # may cause error
print(z)
The code above defines a function called get_name()
that prompts the user to enter their name and stores the input in the x
variable.
If the x
variable is not an empty string, the function returns the value of x
.
Next, the code defines the y
string with the value " is your name"
.
Then, it calls the get_name()
function and concatenates the returned value with the y
string using the +
operator, and assigns the result to the z
variable.
There is a potential for the code to raise a TypeError: unsupported operand type(s) for +: ‘NoneType’ and ‘str’ error.
When the user enters an empty string when prompted for their name, the get_name()
function will return the None
value. This causes the +
operator to raise the error during concatenation.
To avoid the error, you can add an else
statement as shown below:
def get_name():
x = input("Enter your name: ")
if x:
return x
else: # when x is empty
return 'Unknown'
y = " is your name"
z = get_name() + y
print(z)
Alternatively, you can store the get_name()
function result to a variable first, then check if the variable equals None
before trying to concatenate it.
See the example below:
def get_name():
x = input("Enter your name: ")
if x:
return x
y = " is your name"
# 👇 Make sure name is not empty
name = get_name()
if name is not None:
z = name + y
else:
z = "You entered an empty string"
print(z)
In this version of the code, the highlighted lines check if the name
variable is None
before trying to concatenate it with the y
string.
Both solutions above are valid to handle any None
value that may appear in your code.
Conclusion
To conclude, the TypeError: unsupported operand type(s) for +: ‘NoneType’ and ‘str’ error occurs in Python when you try to use the +
operator to concatenate a None
with a string.
To avoid this error, you need to make sure that you are not trying to concatenate a None
value with a string using the +
operator.
You can check if a variable is None
before trying to concatenate it with a string, or you can explicitly set the return
statement in a function.
You can use the examples in this article to fix the error like a professional. 👍