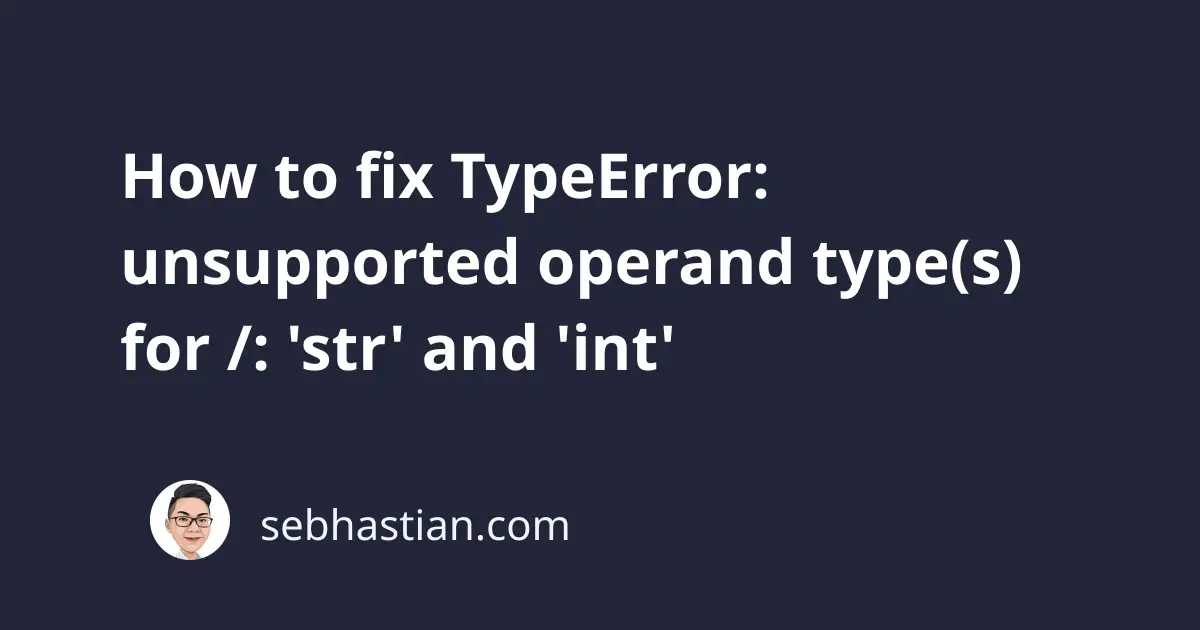
One error that you might encounter when running Python code is:
TypeError: unsupported operand type(s) for /: 'str' and 'int'
This error occurs when you attempt to divide an str
type value with an int
value using the division /
operator.
This tutorial will show you an example that causes this error and how to fix it.
How to reproduce this error
Suppose you have two variables: one is a string and another is an integer as shown below:
x = "8"
y = 4
Now suppose you try to divide the x
value by y
as shown below:
z = x / y
Output:
Traceback (most recent call last):
File "main.py", line 4, in <module>
z = x / y
~~^~~
TypeError: unsupported operand type(s) for /: 'str' and 'int'
The above error shows that Python doesn’t support dividing a string type value with an integer type value.
This error also occurs when you try to perform division in pandas DataFrame object. Suppose you have a DataFrame object as follows:
import pandas as pd
df = pd.DataFrame({
"total_price": [5000, 2000, 3000],
"quantity": ['4', '2', '6']
})
Next, you want to divide the total_price
by quantity
to find the price of each item. You’ll get the same error:
df['item_price'] = df.total_price / df.quantity
# TypeError: unsupported operand type(s) for /: 'int' and 'str'
The error occurs because the quantity
column is of str
type.
Python arithmetic operators are strict. You can’t use +
, -
, /
, and *
to operate on data of different types.
How to resolve this error
To resolve this error, you need to convert the variable or column that has the str
type to int
type before performing the division.
You can convert a string type into an integer by calling the int()
function as follows:
x = "8"
y = 4
z = int(x) / y # ✅
print(z) # 2.0
By converting x
to an integer, the division operation runs without raising an error.
When you have a pandas DataFrame, you need to call the astype()
method on the column object you want to convert.
The example below converts the quantity
column to the int
type:
import pandas as pd
df = pd.DataFrame({
"total_price": [5000, 2000, 3000],
"quantity": ['4', '2', '6']
})
df['item_price'] = df.total_price / df.quantity.astype(int) # ✅
print(df)
Output:
total_price quantity item_price
0 5000 4 1250.0
1 2000 2 1000.0
2 3000 6 500.0
Note that the quantity
column is still a string type as it only gets converted when you perform the division operator.
If you want to convert the column permanently, you need to reassign the column as shown below:
import pandas as pd
df = pd.DataFrame({
"total_price": [5000, 2000, 3000],
"quantity": ['4', '2', '6']
})
df['quantity'] = df.quantity.astype(int)
df['item_price'] = df.total_price / df.quantity
print(df)
Now the quantity
column is changed to integer permanently. Here, you don’t need to call the astype()
method again when calculating the price of each item.
Conclusion
The TypeError: unsupported operand type(s) for /: 'str' and 'int'
occurs when you try to divide a string type data with an integer type data.
To fix this error, you need to convert the string value into an integer value. Use the int()
function if you have a regular Python variable, or use the astype(int)
method when you have a pandas DataFrame column.
I hope this tutorial is helpful. Happy coding and see you again! 🙌