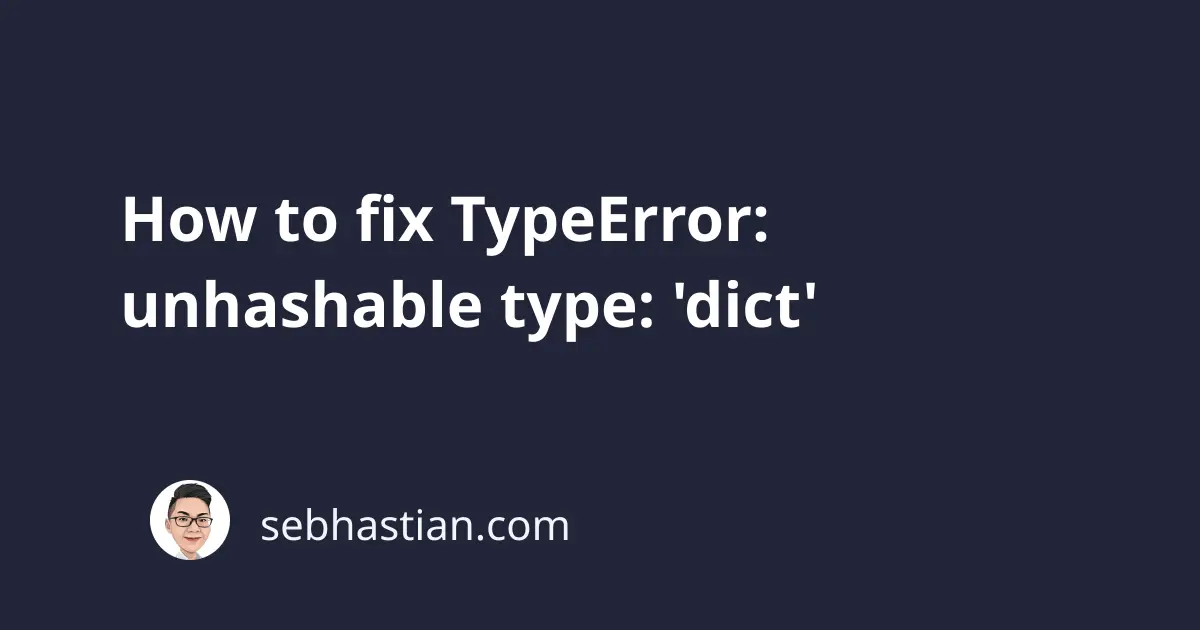
While working with Python dictionaries, you might encounter the following error:
TypeError: unhashable type: 'dict'
This error usually occurs when you attempt to set a dictionary object as the key of a key-pair value.
This tutorial will show you an example that causes this error and how to fix it in practice
How to reproduce this error
Suppose you create a dictionary object as follows:
dict_a = {"last_name": "Doe"}
Next, you tried to set this dictionary as an item in another dictionary as follows:
dict_a = {"last_name": "Doe"}
dict_b = {
"first_name": "Jane",
dict_a: "Andy"
}
Output:
Traceback (most recent call last):
File "main.py", line 1, in <module>
dict_b = {
TypeError: unhashable type: 'dict'
This error occurs because the dict_a
object is being used as the key to the second item in the dict_b
object.
In Python, dictionary keys must be a hashable type such as a string, a tuple, a boolean, or an integer. A dictionary is not hashable, so the error is raised.
How to fix this error
To resolve this error, you need to make sure that you’re using a hashable type as the key in a dictionary object.
If you need to add a value from another dictionary, you can assign the value you need to a string key as follows:
dict_a = {"last_name": "Doe"}
dict_b = {
"first_name": "Jane",
"last_name": dict_a["last_name"]
}
print(dict_b)
Output:
{'first_name': 'Jane', 'last_name': 'Doe'}
If you want to add dict_a
into dict_b
, then you need to assign the dictionary as a value to a key you specified in dict_b
:
dict_a = {"first_name": "Jane", "last_name": "Doe"}
dict_b = {
"last_login": "20th March 2023",
"detail" : dict_a
}
print(dict_b)
Output:
{
'last_login': '20th March 2023',
'detail': {'first_name': 'Jane', 'last_name': 'Doe'}
}
In the example, the dict_a
object is added under the detail
key defined in dict_b
.
You can’t add a dictionary as a key to another dictionary, but you can add one as a value.
If you want to add just the key-value pair without creating a nested dictionary, you can use a for
loop and the dict.items()
method.
The example below shows how to add all key-value pairs in dict_a
to dict_b
:
dict_a = {"first_name": "Jane", "last_name": "Doe"}
dict_b = {
"last_login": "20th March 2023"
}
for key, value in dict_a.items():
dict_b[key] = value
print(dict_b)
Output:
{
'first_name': 'Jane',
'last_name': 'Doe',
'last_login': '20th March 2023'
}
By using the for
loop, you don’t need to create a new key in dict_b
as in the previous example.
If you absolutely need to use a dictionary as a key in your dictionary object, then you need to use the json.dumps()
method to convert the dictionary into a string:
import json
dict_a = {"first_name": "Jane", "last_name": "Doe"}
dict_b = {
json.dumps(dict_a): "user"
}
print(dict_b)
Output:
{
'{"first_name": "Jane", "last_name": "Doe"}': 'user'
}
While it works, I think you have a mistake in your source code if you ever need to use a dictionary as a key in another dictionary.
Unless you have a really unique case, strings and integers should be used as keys in a dictionary object to avoid complexity.
I hope this tutorial is helpful. Until next time! 🙌