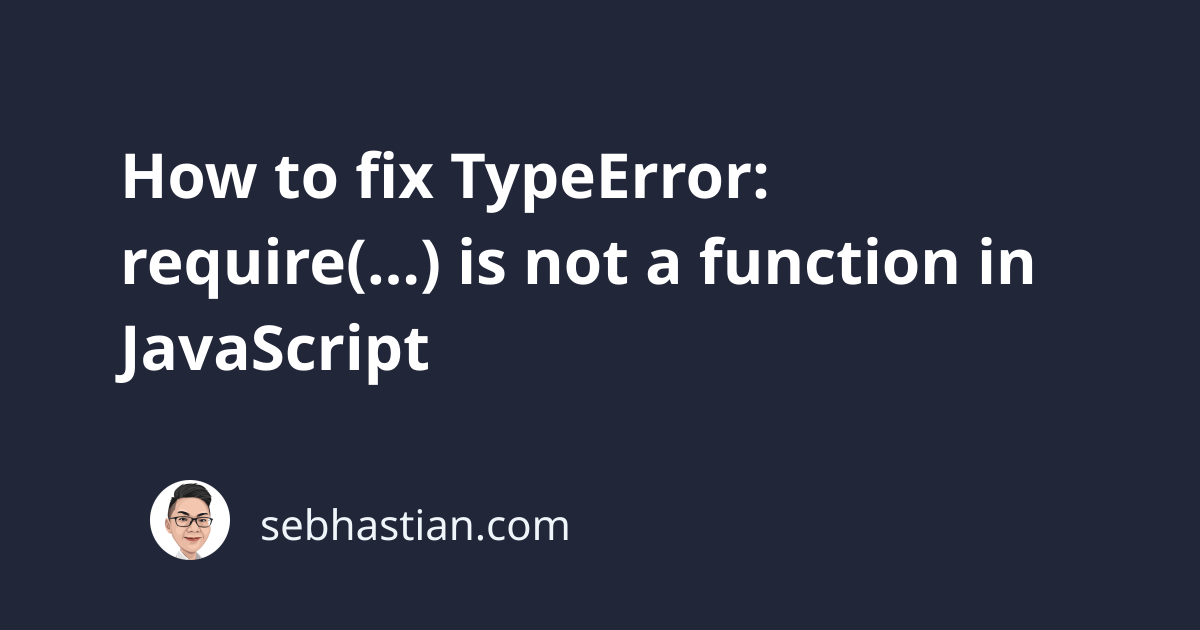
When running a JavaScript application, you might encounter the following error:
TypeError: require(...) is not a function
This error usually occurs because of two possible causes:
- You called an Immediately Invoked Function Expression (IIFE) after calling the
require()
function - You require a module that doesn’t have a function as its
export
contents.
This tutorial will show you an example that causes this error for each scenario.
1. Calling an IIFE after requiring a module
Immediately Invoked Function Expression (IIFE for short) is a function that gets executed immediately after its declaration.
To create an IIFE, you can use both the old function and new arrow function syntax:
(function() {
/* function definition */
})()
(() => {
/* function definition */
})()
When you put a call to IIFE after calling the require function like this:
const fs = require("fs")
(function() {
console.log("Hello World!")
})()
JavaScript will respond with the error:
TypeError: require(...) is not a function
This error occurs because there’s no semicolon ;
symbol after the require()
function call.
When you declare an IIFE after a function call, JavaScript doesn’t automatically add a semicolon because you might be trying to use IIFE as an argument to that function call.
When you execute the example code, JavaScript sees the code as follows:
const fs = require("fs")(function() {
console.log("Hello World!")
})()
Now because the fs
module doesn’t return a function, the error says ‘require(…) is not a function’.
To prevent this error, you need to add a semicolon manually after calling the require()
function:
const fs = require("fs");
(function() {
console.log("Hello World!")
})()
Now the code will be executed and you won’t see the error.
2. You require a module that doesn’t have a function as its export contents
Another cause of this error is when you require a module that doesn’t return a function, but you defined some arguments next to the require()
function.
For example, suppose you have a file named util.js
with the following contents:
module.exports = "Hello World!";
Next, you require that module and add some function arguments like this:
const x = require("./util")("Hello", "John")
You’ll get the same error:
const x = require("./util")("Hello", "John")
^
TypeError: require(...) is not a function
This error occurs because the require()
function doesn’t return a function, but a string. The code is executed like this:
"Hello World!"("Hello", "John")
To run the code properly, you need to make sure that the required file returns a function.
If you change the util.js
exports as follows:
function greet(greeting, name) {
return `${greeting}, ${name}!`;
}
module.exports = greet;
Then the require()
function would work because it’s the same as calling the greet()
function immediately:
const x = require("./util")("Hello", "John")
// similar as:
greet("Hello", "John");
The x
variable now contains the return value of the greet()
function, and the error doesn’t appear again.
Conclusion
The JavaScript TypeError: require(…) is not a function happens when you call the value returned by the require()
function like a function.
To resolve this error properly, you need to check on the value returned by the require()
function, and make sure that you’re not adding an IIFE or function arguments when the value is not a function.
I hope this guide helps you fix the error. Happy coding! 👍