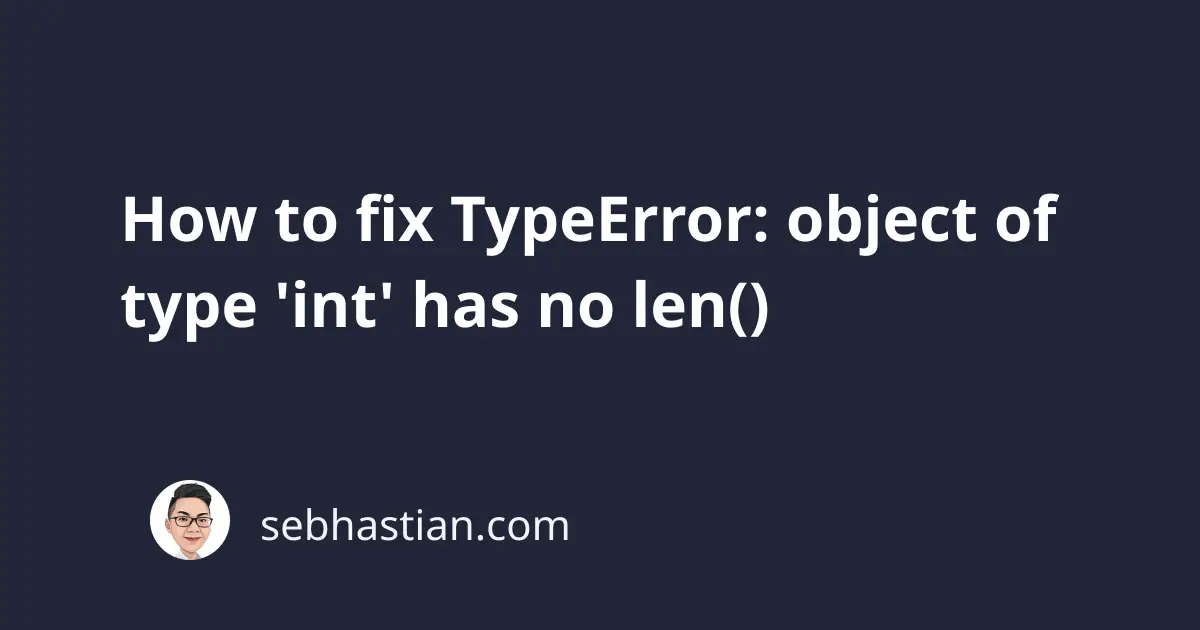
When running Python code, one error that you might encounter is:
TypeError: object of type 'int' has no len()
This error occurs when you call the len()
function and pass an int
type object as its argument.
Let’s see an example that causes this error and how to fix it.
How to reproduce this error
Suppose you assigned an int
value to a variable as follows:
x = 7
Next, you try to find the length of the x
variable above using the len()
function:
x = 7
print(len(x))
The call to the len()
function causes an error:
Traceback (most recent call last):
File "main.py", line 3, in <module>
print(len(x))
TypeError: object of type 'int' has no len()
This error occurs because an int
object doesn’t have the __len__
attribute, which is the value returned by Python when you call the len()
function.
In Python, only sequences like Strings, Lists, Tuples, .etc can be used as an argument of the len()
function
How to fix this error
To resolve this error, you need to make sure that you don’t pass an int
object to the len()
function
You can check the tipe of the object first by calling the isinstance()
function as follows:
x = 7
if isinstance(x, int):
print("Variable is an integer")
else:
print(len(x))
By putting the len()
function inside the else
block, it won’t be called when the x
variable is an int
object.
You might want to call the len()
function on a list or a string, so you need to check the type of the variable first using the type()
function:
x = 7
y = [1, 2, 3]
z = "Hello!"
print(type(x)) # <class 'int'>
print(type(y)) # <class 'list'>
print(type(z)) # <class 'str'>
If you have an int
object when you should have another type (like a list
) then you might have assigned the wrong value somewhere in your code.
For example, the list.pop()
method returns the removed item. If the list contains int
objects, then an int
will be returned:
y = [12, 13, 14]
item = y.pop(1) # returns 13
print(len(item)) # 13
When you called len()
on the return value of the pop()
method, you’re calling the function on an int
object. This causes the error.
If you only want to remove items from your list and then get the lenght of the list, call the len()
function on the list object as follows:
y = [12, 13, 14]
y.pop(1)
print(len(y)) # 2
You don’t need to save the value returned by the pop()
method, and just call len()
on the same list object.
You need to carefully review your code and change the variable assignment as necessary to fix this error.
I hope this tutorial helps. Happy coding! 🙌