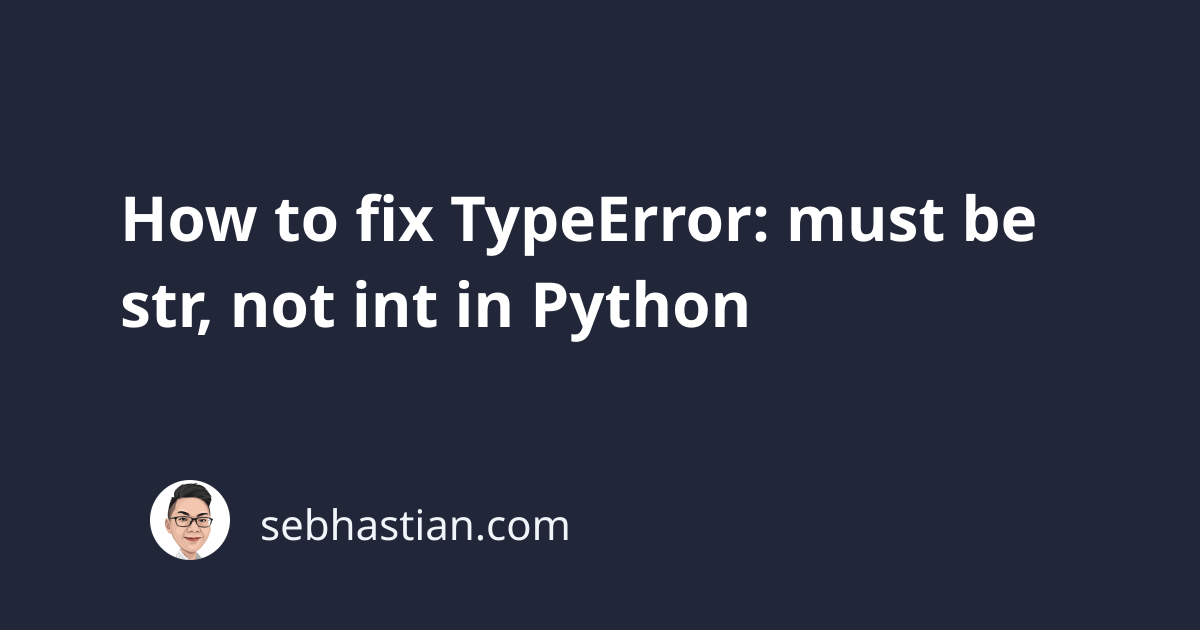
One error that you might encounter when working with Python is:
TypeError: must be str, not int
This error occurs when you specify an integer in the place where Python expects a string.
This tutorial will show you examples that cause this error and how to fix it.
How to reproduce this error
Suppose you have an integer variable that stores today’s temperature as follows:
temp_f = 42
Next, you try to print a sentence that includes the temperature value as follows:
temp_f = 42
print("Today's temperature is: " + temp_f + "F")
Output:
Traceback (most recent call last):
File "main.py", line 3, in <module>
print("Today's temperature is: " + (temp_f) + "F")
TypeError: must be str, not int
Python doesn’t allow you to concatenate a string together with an integer.
How to fix this error
To resolve this error, you can convert any integer value in your print statement into a string using the str()
function.
Example 1:
temp_f = 42
print("Today's temperature is: " + str(temp_f) + "F")
Example 2:
temp_f = 42
today_temp = "Today's temperature is: " + str(temp_f) + "F"
Run the code again and notice that you didn’t receive any error this time.
I hope this tutorial is helpful. Happy coding! 👋