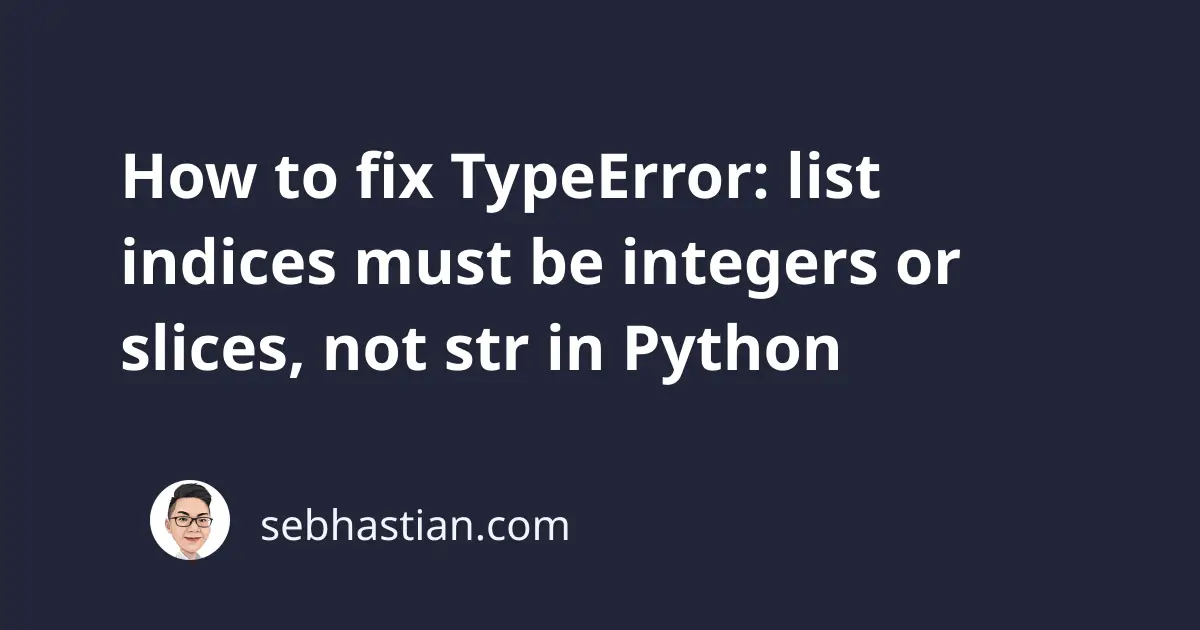
One error that you might encounter when running Python code is:
TypeError: list indices must be integers or slices, not str
There are two possible causes for this error:
- Accessing an element of a list using a string
- Slicing a list using a string in the slice syntax
This tutorial will show you examples that cause this error and how I fix it in practice.
1. Accessing an element of a list using a string
This error frequently occurs when you try to access an element of a list using a string.
The most common scenario is you’re using the input()
function to make the user choose the element to select as follows:
my_list = ["Apple", "Banana", "Orange"]
print(my_list)
choice = input("Which fruit you want to pick? (0, 1, or 2): ")
print(f"You chose {my_list[choice]}")
When you execute the code, Python will show an error after you send an input:
['Apple', 'Banana', 'Orange']
Which fruit you want to pick? (0, 1, or 2): 2
Traceback (most recent call last):
File "main.py", line 6, in <module>
print(f"You chose {my_list[choice]}")
TypeError: list indices must be integers or slices, not str
The error happens because the input()
function always returns a str
object.
To resolve this error, you need to call the int()
function to convert the choice
to an integer:
my_list = ["Apple", "Banana", "Orange"]
print(my_list)
choice = input("Which fruit you want to pick? (0, 1, or 2): ")
choice = int(choice)
print(f"You chose {my_list[choice]}")
Now you can run the code without triggering the error:
['Apple', 'Banana', 'Orange']
Which fruit you want to pick? (0, 1, or 2): 2
You chose Orange
2. Slicing a list using a string in the slice syntax
The same error also occurs when you try to slice a list using a string.
For example:
my_list = ["Apple", "Banana", "Orange"]
# Get the last two items
sliced_list = my_list["1":]
Because slicing can only be done using integers, you need to convert the string into a number using the int()
function:
my_list = ["Apple", "Banana", "Orange"]
# Get the last two items
sliced_list = my_list[int("1"):]
print(sliced_list)
Output:
['Banana', 'Orange']
As you can see, the code works when you use an integer number for the slicing.
Conclusion
The TypeError: list indices must be integers or slices, not str
occurs when you use strings to access a list at a specific position.
To resolve this error, you need to make sure you put integers or slices inside the square brackets []
to access a list.
I hope this tutorial helps. Happy coding! 👍