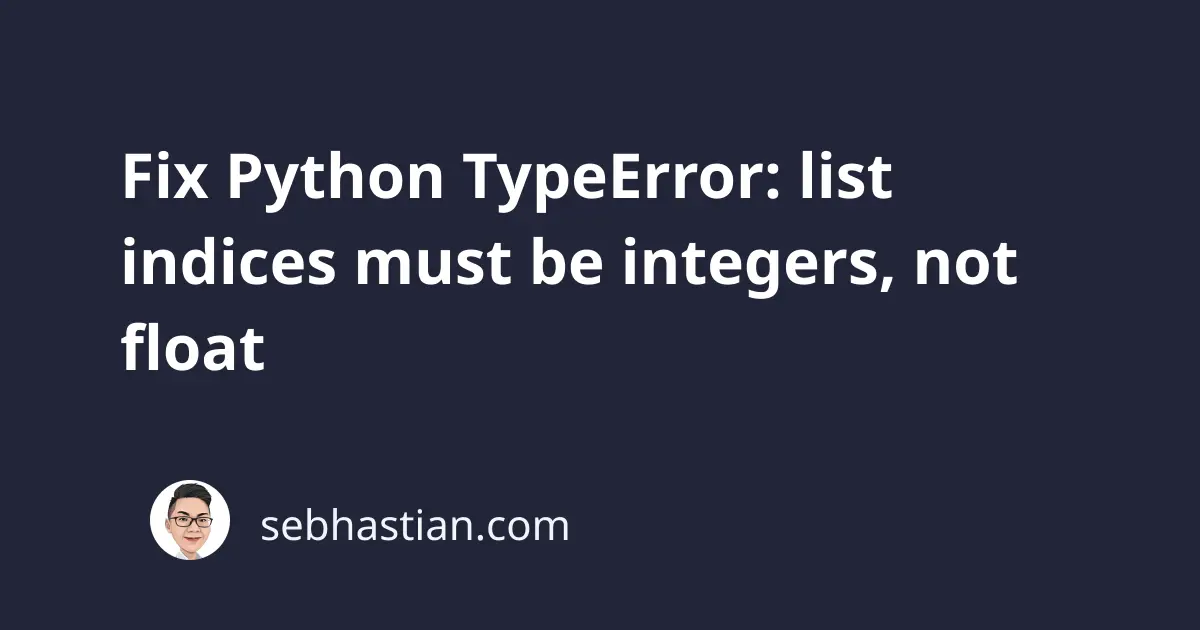
Python TypeError: list indices must be integers or slices, not float occurs when you try to access a list item using a number with floating points (like 2.5
or 4.5
)
To solve this error, you need to make sure you are using integers when accessing an element from a list.
Consider the Python code below:
fish = ['salmon', 'tuna', 'sardine']
# Access fish using an integer ✅
print(fish[0]) # salmon
# Access fish using a float ❌
print(fish[1.5]) # ❗️TypeError
When accessing the fist
list item using a float value like 1.5
as shown above, Python will respond with the TypeError:
This is because Python internally assigns integer value starting from 0
as the index numbers for items in a list.
You can access an element at a specific index using an integer or a slice, but you can’t use a float because Python doesn’t use that type as the index number.
To fix this error, you need to convert the floating number into a whole number using the int()
function:
fish = ['salmon', 'tuna', 'sardine']
index = int(1.5) # returns 1
print(fish[index]) # tuna
The int()
function returns an integer value gained from the argument you provided to it.
One common cause for this TypeError is when you use the result of division operator (/
) to access a list item.
The division operator in Python always returns a floating number even when the result should have been an integer:
fish = ['salmon', 'tuna', 'sardine']
index = 2 / 1
print(index) # 2.0
print(fish[index]) # ❗️TypeError
There are two ways you can avoid the error above:
- Convert the result to integer using the
int()
function - Use the floor division operator
//
instead of/
The floor division operator rounds down the result of the division so that you get an integer number instead of a decimal number:
fish = ['salmon', 'tuna', 'sardine']
index = 2 // 1
print(index) # 2
print(fish[index]) # sardine
Besides an integer, You can also use a slice to access a range of elements from a list.
For example, the following code would print a sublist containing the second and third elements of the fish
list:
fish = ['salmon', 'tuna', 'sardine']
print(fish[1:3]) # ['tuna', 'sardine']
When using a slice, keep in mind that the list index starts from 0
, so the second element has 1
as its index number.
You need to separate the start index and the end index using a colon :
like this:
fish = ['salmon', 'tuna', 'sardine']
# The end index is excluded
print(fish[0:2]) # ['salmon', 'tuna']
# Omit the end index
print(fish[1:]) # ['tuna', 'sardine']
The element at the start index is included in the slice, while the element at the end index is excluded.
You can also omit the end index to get the element from start index to the end of the list.
Conclusion
The Python TypeError: list indices must be integers or slices, not float occurs when you attempt to access a list item using a number with floating points.
To fix this error, you can convert the floating number to an integer using the int()
function or use a slice to access a range of elements in the list.
It is important to remember that Python uses integer index numbers starting from 0
for items in a list. Make sure you are using the right index number or you’ll get the wrong element from the list.
Finally, some examples of using a slice to access a list are added so that you see the right way to access a Python list.