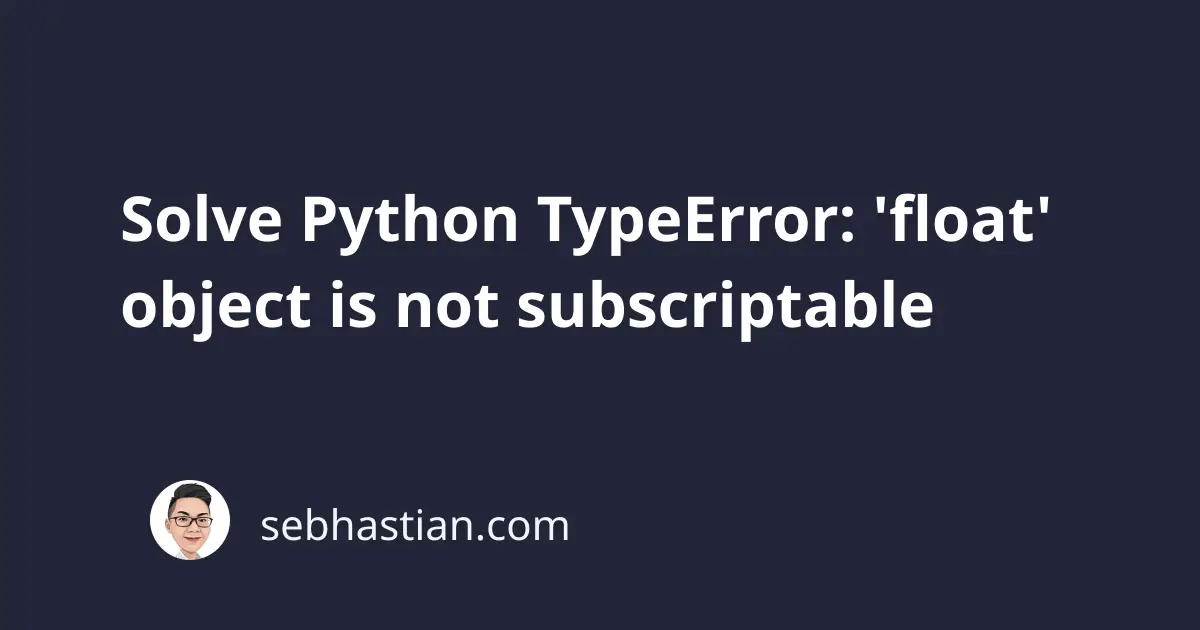
The “TypeError: ‘float’ object is not subscriptable” error in Python occurs when you try to use the square brackets (i.e., []
) to access a float type value at a specific index.
In Python, the square brackets are used to index or slice sequences, such as strings, lists, and tuples. However, floats are not sequences, and they do not support indexing or slicing.
If you try to use the square brackets to access a float value, you will get the error. The code below:
score = 7.89
# Access 7 from the variable
print(score[0])
Gives the following error:
Traceback (most recent call last):
File "/script.py", line 3, in <module>
print(score[0])
~~~~~^^^
TypeError: 'float' object is not subscriptable
To fix this error, you need to make sure that you are not trying to index a float value.
If you need to access a specific digit of a float, you need to convert the float to a string first.
This is because a string is subscriptable, while a float is not:
score = 7.89
# Access the score as string
print(str(score)[0]) # 7
print(str(score)[1]) # .
print(str(score)[2]) # 8
print(str(score)[3]) # 9
When you are doing math operations on the value, you need to convert the string value to an integer before running the next operation:
score = 7.89
# Access the first digit
first_digit = str(score)[0] # 7
# Add to the first digit
result = int(first_digit) + 10 # 7 + 10
print(result) # 17
By combining the str()
and int()
functions, you can access a float value at a specific index and then convert it into an integer value.
This allows you to do mathematical operations on the values you have created.
Conclusion
To conclude, the Python TypeError: float object is not subscriptable issue occurs when you try to access a float value using the square brackets.
When you need to access a float value at a specific index, you need to convert that value into a subscriptable type first, such as a string.
Thanks for reading. I hope this article helps you. 🙏