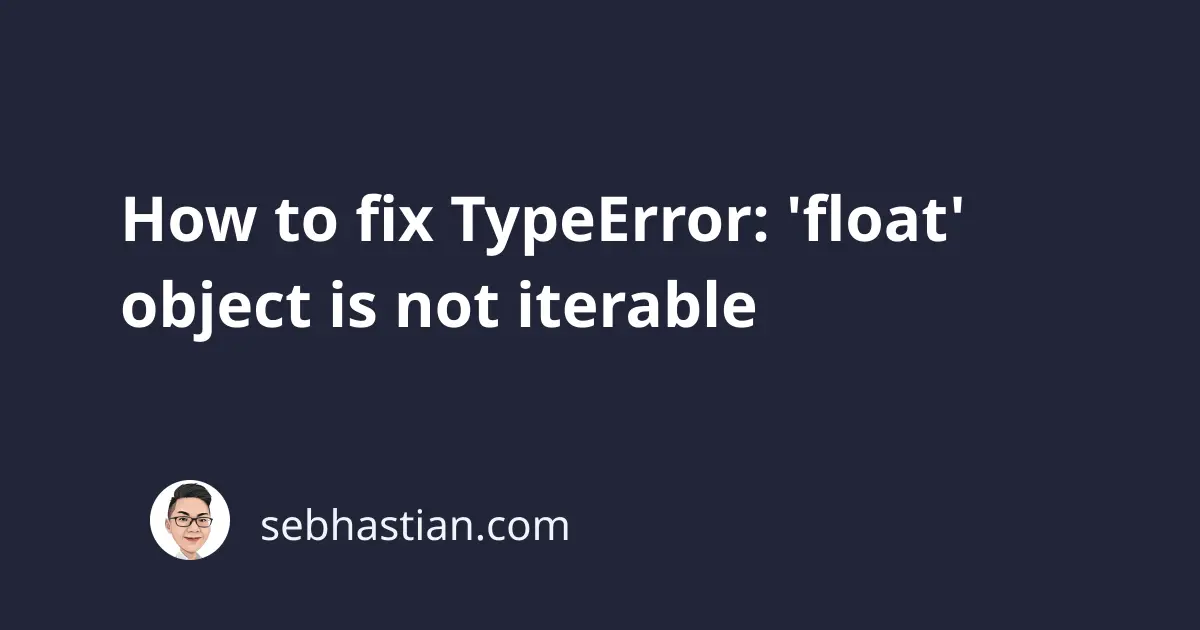
One error that you might encounter when coding in Python is:
TypeError: 'float' object is not iterable
This error usually occurs when you pass a float
object into a function or syntax where an iterable is expected. There are three common scenarios in which this error occurs:
- Using a float in a for loop
- Call list() with float objects
- Call the sum() function and pass float objects
This tutorial shows you examples that cause this error and how to fix it.
1. Using a float in a for loop
One common cause of this error is when you pass a float when attempting to iterate using a for
loop.
Suppose you have a for
loop code as follows:
num = 4.5
for i in num:
print(i)
The num
variable is a float object, so it’s not iterable and causes the following error when used in a for
loop:
Traceback (most recent call last):
File "main.py", line 3, in <module>
for i in num:
TypeError: 'float' object is not iterable
A for
loop requires you to pass an iterable object, such as a list
or a range
object.
If you want to use a float, you need to convert it to an int
using the int()
function, then convert it to a range with range()
as follows:
num = 4.5
for i in range(int(num)):
print(i)
Output:
0
1
2
3
Unlike the for
loop in other programming languages where you can use an integer value, the for
loop in Python requires you to pass an iterable. Both int
and float
objects are not iterable.
2. Call list() with float objects
This error also occurs when you call the list()
function and pass float objects to it.
Suppose you want to create a list from float values as follows:
num = 4.5
my_list = list(num)
Python shows “TypeError: ‘float’ object is not iterable” because you can’t pass a float when creating a list.
To create a list from a float, you need to surround the argument in square brackets:
num = 4.5
my_list = list([num])
print(my_list) # [4.5]
The same error also occurs when you create a dictionary, tuple, or set using a float object. You need to use the appropriate syntax for each function:
num = 5.5
# Float to dictionary
val = dict(num=num)
print(val) # {'num': 5.5}
# Float to tuple
val = tuple([num])
print(val) # (5.5,)
# Float to set
val = set([num])
print(val) # {5.5}
For the dict()
function, you need to pass arguments in the form of key=value
for the key-value pair.
3. Call the sum() function and pass float objects
Another condition where this error might occur is when you call the sum()
function and pass float objects to it.
Suppose you try to sum floating numbers as shown below:
x = 4.5
y = 6.8
z = sum(x, y)
You’ll get “float is not iterable” error because the sum()
function expects a list.
To fix this, surround the arguments you passed to sum()
with square brackets as follows:
x = 4.5
y = 6.8
z = sum([x, y])
print(z) # 11.3
Notice how the sum
function works without any error this time.
Conclusion
The Python error “‘float’ object is not iterable” occurs when you pass a float object when an iterable is expected.
To fix this error, you need to correct the assignments in your code so that you don’t pass a float in place of an iterable, such as a list or a range.
If you’re using a float in a for
loop, you can use the range()
and int()
functions to convert that float into a range. You can also convert a float into a list by adding square brackets []
around the float objects.
I hope this tutorial is helpful. Happy coding! 👍