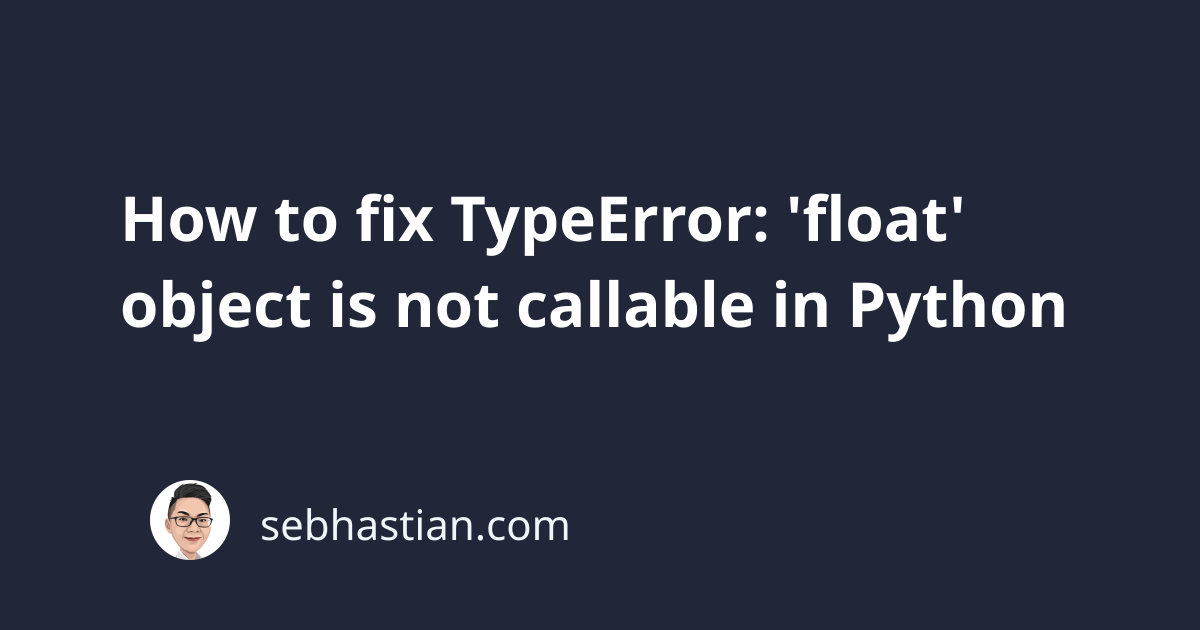
One error that you might encounter when working with Python is:
TypeError: 'float' object is not callable
This error occurs when you mistakenly call a float
object as if it was a function.
The following code is an example that causes this error:
5.555()
There are three possible scenarios that cause this error:
- You attempt to multiply a float without adding the
*
operator - You override the
float()
function with a float object - You have a function and a float variable with the same name
This tutorial shows how to reproduce the error in each scenario and how to fix it.
1. You attempt to multiply a float without adding the *
operator
First, you declared a simple float variable as follows:
my_float = 7.2
Next, you attempt to multiply the float variable with a number acquired from math operations as follows:
x = my_float(5 - 2)
Output:
Traceback (most recent call last):
File "main.py", line 3, in <module>
x = my_float(5 - 2)
^^^^^^^^^^^^^^^
TypeError: 'float' object is not callable
The error occurs because you’re adding parentheses next to a float object.
While the above is a valid multiplication formula in real life, this makes Python thinks you’re trying to call a function named my_float()
instead of trying to do a multiplication.
To resolve this error, you need to make sure that you’re not adding parentheses next to a float object.
Since you want to multiply the variable, add the multiplication operator *
as follows:
my_float = 7.2
x = my_float * (5 - 2)
print(x) # 21.6
This time, the multiplication succeeds and we didn’t receive an error.
2. You override the float()
function with a float object
The built-in function named float()
is used to convert an object of another type into a float object.
For example, here’s how to convert a string into a float:
my_string = "7.55"
my_float = float(my_string)
print(my_float) # 7.55
If you mistakenly create a variable named float
, then the float()
function would be overwritten.
When you call the float()
function, the error would occur as follows:
float = 3.45
another_float = float("7.55")
Output:
Traceback (most recent call last):
File "/main.py", line 3, in <module>
another_float = float("7.55")
^^^^^^^^^^^^^
TypeError: 'float' object is not callable
Because you created a variable named float
, the float()
function gets replaced with that variable, causing you to call a float object instead of the built-in function.
To avoid this error, you need to declare the variable using another name:
my_float = 3.45 # ✅
another_float = float("7.55")
This way, the keyword float
would still point to the float function, and the error won’t be raised.
You have a function and a float variable with the same name
Suppose you have a function named get_name()
that returns a string as follows:
def get_name():
return "Nathan"
Then down the line, you declared a variable using get_name
as the identifier:
get_name = 7.67
This cause the get_name
variable to shadow the get_name()
function. When you try to call the function, Python thinks you’re calling the variable instead:
def get_name():
return "Nathan"
get_name = 7.67
res = get_name() # TypeError: 'float' object is not callable
To resolve this error, make sure that there’s no duplicate name in your source code.
Each function and variable must have a unique name:
def get_name():
return "Nathan"
x = 7.67
res = get_name() # ✅
Now that the name for the function and the variable are unique, we didn’t receive any error.
Conclusion
The TypeError: 'float' object is not callable
occurs in Python when you call a float object using parentheses like it’s a function.
To resolve this error, you need to make sure that you’re not calling a float object in your source code.
I hope this tutorial helps. Happy coding!