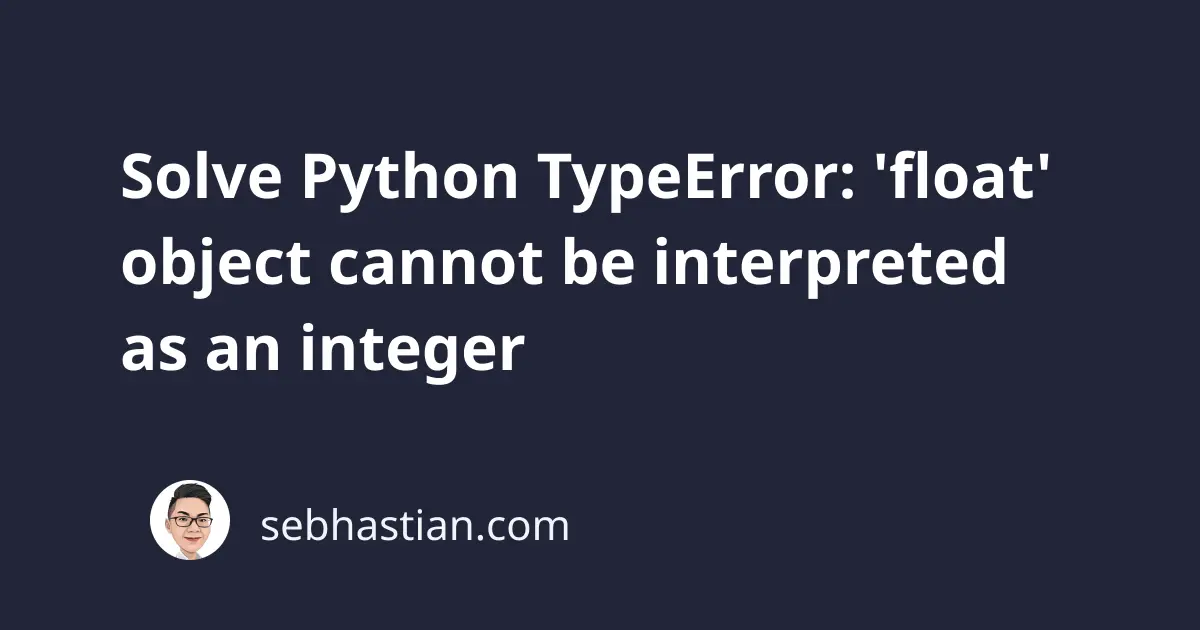
The Python TypeError: ‘float’ object cannot be interpreted as an integer happens when you pass a float value to a function that needs an integer.
You need to convert the float value into an integer to resolve this error. Let’s see how.
In Python, a float is a data type that represents a decimal number, while an integer is a data type that represents a whole number.
Here are some examples of float and integer values in Python:
# Float values
x = 3.14 # This is a float
y = 0.5 # This is also a float
# Integer values
a = 10 # This is an integer
b = -5 # This is also an integer
If you pass a float where Python expects an integer, the error occurs.
One common situation where this error can occur is when using the range()
function in Python.
The range()
function is a built-in function that generates a sequence of numbers, and it requires integer arguments to define the range of numbers to generate.
If you try to use a float value as an argument for the range()
function, Python will raise a TypeError because it cannot interpret the float value as an integer:
x = 3.5
for i in range(x): # ❗️TypeError
print(i)
To solve this error, you need to convert the float value into integer using the int()
function:
# 👇 convert float to int
x = int(3.5)
for i in range(x): # ✅
print(i)
The float value may also appear as a result of a division operation.
When you use the division operator (/
), Python always returns a float value:
x = 10 / 5
print(x) # 2.0
for i in range(x): # ❗️TypeError
print(i)
Even though 10
divided by 5
should return a whole number 2
, the division operator in Python is programmed to always return a decimal number.
When you need an integer number, you need to use the floor division operator (//
).
See the example below:
x = 10 // 5
print(x) # 2
for i in range(x):
print(i)
The floor division operator rounds down the result of the division so that you get a whole number instead of a decimal number.
Finally, you can also use the Python round()
function to round a decimal number up or down depending on the closest whole number.
Consider the following example:
x = 2 * 3.4 # 6.8
x = int(x) # 6
for i in range(x):
print(i)
The result of 2 * 3.4
is 6.8
, but the int()
function converts the specified value without considering the floating point number at all. As a result, it always rounds down.
To consider the floating point number, use the round()
function instead of the int()
function like this:
x = 2 * 3.4 # 6.8
x = round(x) # 7
for i in range(x):
print(i)
When you use the round()
function, the number can be rounded up when the floating point is .6
or greater.
Conclusion
To conclude, the TypeError: ‘float’ object cannot be interpreted as an integer happens in Python when you try to use a float value in place of an integer, such as when using the range()
function.
To fix this error, you need to convert the float value to an integer using the int()
or round()
functions.
When doing a division, you can also use the floor division operator (//
) so that the result is an integer.
By following the examples in this article, you can fix this error in your Python code. 👍