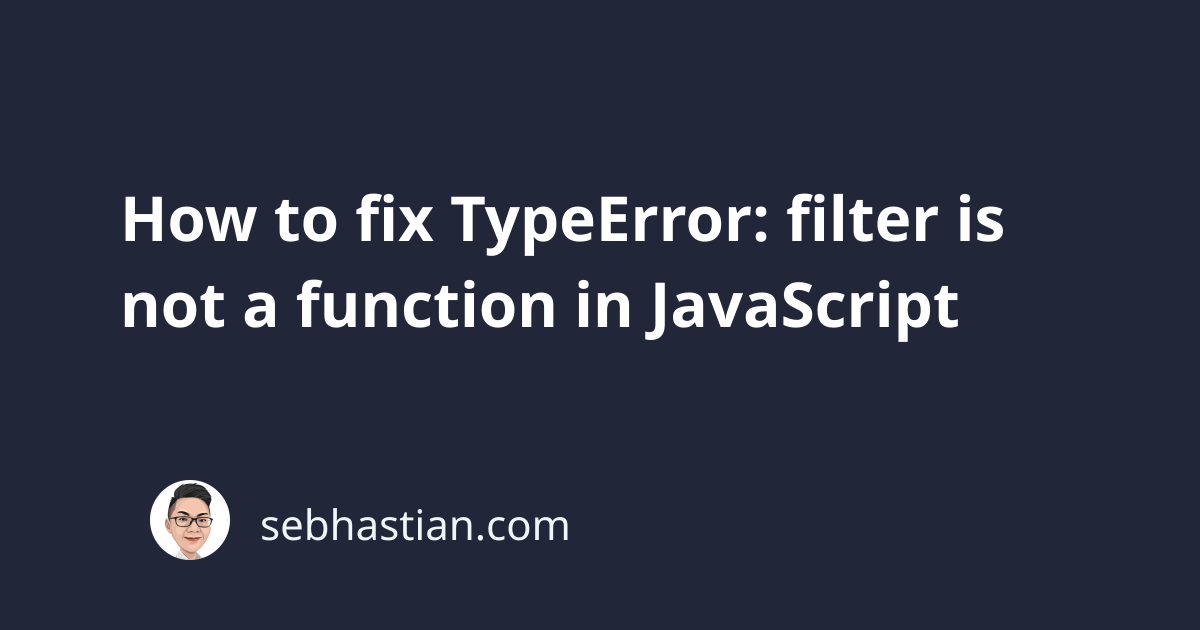
When running JavaScript code, you might encounter the following error:
TypeError: filter is not a function
This error occurs when you call the filter()
method from a variable that’s not an array.
Anytime you call the filter()
method from a variable that’s not an array, JavaScript will throw this error.
Let’s see an example that causes this error and how you can fix it.
How to reproduce this error
Suppose you try to filter an object and return objects that match specific criteria, you might write code as follows:
let products = {
1: { id: 1, name: "Apple" },
2: { id: 2, name: "Orange" },
3: { id: 3, name: "Mango" },
4: { id: 4, name: "Banana" },
};
let filteredProduct = products.filter(function (prod) {
return prod.id === 1;
});
But when you run the code above, you get the following error:
let filterProduct = products.filter(function (prod) {
^
TypeError: products.filter is not a function
This error occurs because the products
variable is an object, which means it doesn’t have the filter()
method.
You can check whether your variable is an array by calling the Array.isArray()
method as follows:
let products = {
1: { id: 1, name: "Apple" },
2: { id: 2, name: "Orange" },
3: { id: 3, name: "Mango" },
4: { id: 4, name: "Banana" },
};
console.log(Array.isArray(products)); // false
When the isArray()
method returns false
, calling the filter()
method on the variable will cause the error.
How to fix this error
To resolve this error, you need to convert the object into an array before calling the filter()
method.
You can do so by calling the Object.values()
method on the object. Assign the returned value to a variable as follows:
let products = {
1: { id: 1, name: "Apple" },
2: { id: 2, name: "Orange" },
3: { id: 3, name: "Mango" },
4: { id: 4, name: "Banana" },
};
let prods = Object.values(products);
console.log(Array.isArray(prods)); // true
Here, the prods
variable is the array form of the products
object.
You can call the filter()
method on the new variable as shown below:
let products = {
1: { id: 1, name: "Apple" },
2: { id: 2, name: "Orange" },
3: { id: 3, name: "Mango" },
4: { id: 4, name: "Banana" },
};
let prods = Object.values(products);
let filteredProduct = prods.filter(function (prod) {
return prod.id === 1;
});
console.log(filteredProduct);
Output:
[ { id: 1, name: 'Apple' } ]
Once you converted the object into an array, the filter()
method worked and the error disappeared.
If you don’t want to declare a new variable, you can also chain the call to Object.values()
and filter()
methods like this:
let products = {
1: { id: 1, name: "Apple" },
2: { id: 2, name: "Orange" },
3: { id: 3, name: "Mango" },
4: { id: 4, name: "Banana" },
};
let filteredProduct = Object.values(products).filter(prod => {
return prod.id === 1;
});
console.log(filteredProduct);
Here, we called the filter()
method on the result of the Object.values()
method immediately.
This code is shorter and more concise when you don’t need to use the array again.
Conclusion
The JavaScript TypeError: filter is not a function
occurs when you call the filter()
method on a value that’s not an array.
This usually occurs when you call the method from an object. If you pass any other non-array type, the method returns an empty array instead.
To resolve this error, you can use the Object.values()
method to convert the object into an array. The object keys will be ignored, but it’s okay because you can’t set keys on an array anyway.
I hope this tutorial helps. Happy coding! 👍