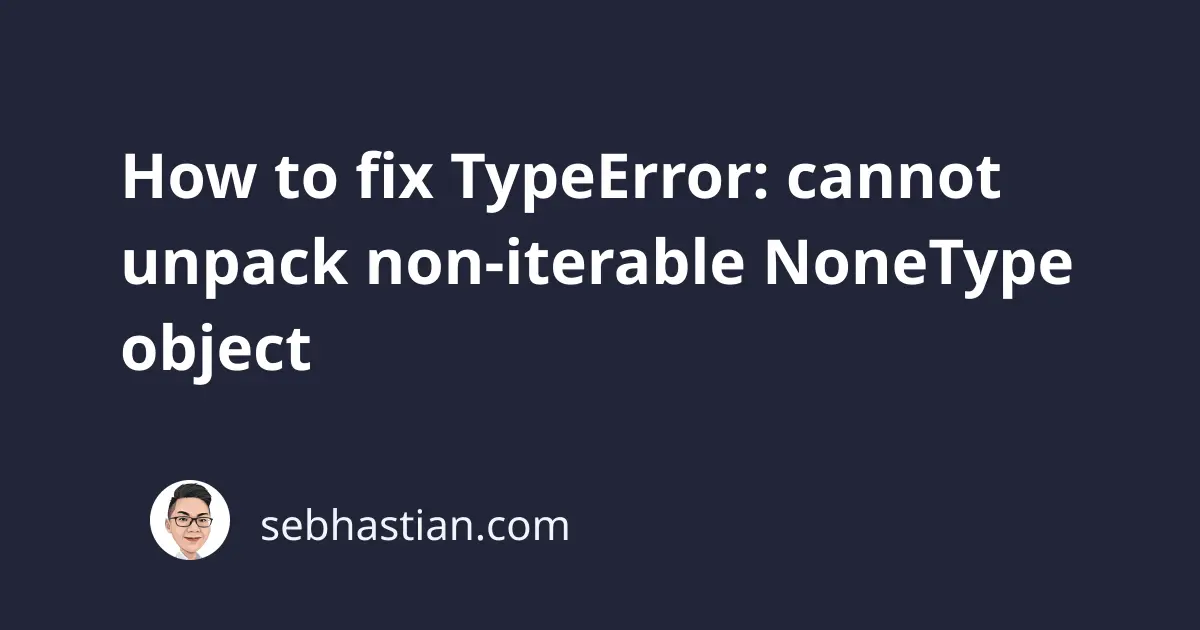
While working with Python sequences such as a List or a Tuple, you might get the following error:
TypeError: cannot unpack non-iterable NoneType object
This error usually occurs when you assign an object of None
value to a set of variables using the unpacking syntax.
There are two common scenarios when this error occurs:
- You unintentionally unpack a None object
- Forgetting the return statement in your function
The following tutorial shows an example that causes this error and how to fix it.
1. You unintentionally unpack a None object
In Python, you can set the items in a sequence to multiple variables based on the index position of those items:
fruits = ["Grape", "Apple", "Mango"]
fruit_1, fruit_2, fruit_3 = fruits
print(fruit_1) # Grape
print(fruit_2) # Apple
print(fruit_3) # Mango
As you can see, each variable gets the value defined in the fruits
list.
The error occurs when you assign the variables to a None
value as follows:
fruit_1, fruit_2, fruit_3 = None
Output:
Traceback (most recent call last):
File "main.py", line 1, in <module>
fruit_1, fruit_2, fruit_3 = None
TypeError: cannot unpack non-iterable NoneType object
To resolve this error, you need to make sure that you’re not unpacking a None
object.
You can do this by using an if
statement to check that the variable you want to unpack is not None
:
fruits = ["Grape", "Apple", "Mango"]
if fruits is not None:
fruit_1, fruit_2, fruit_3 = fruits
else:
print("The variable is None")
By using an if
statement as shown above, Python only unpacks the variable fruits
when it’s not a None
object.
Sometimes, you also get this error when you unintentionally unpack a variable that has a None
value.
For example, you unpack the variable that gets assigned the return value of the sort()
method like this:
fruits = ["Grape", "Apple", "Mango"]
fruits = fruits.sort()
fruit_1, fruit_2, fruit_3 = fruits # ❌
The error occurs because the sort()
method modifies the original list without returning anything. When you assign the return of sort()
to a variable, that variable gets assigned a None
value.
To resolve the error, you need to call sort()
without assigning the return value to a variable as follows:
fruits = ["Grape", "Apple", "Mango"]
fruits.sort()
fruit_1, fruit_2, fruit_3 = fruits # ✅
print(fruit_1) # Apple
print(fruit_2) # Grape
print(fruit_3) # Mango
Note that this time We don’t receive any error message because the unpack works as expected.
2. Forgetting the return statement in your function
Suppose you have a function that returns a list as follows:
def get_list():
my_list = [1, 2, 3]
Then, you try to unpack the object returned when you call the function as shown below:
a, b, c = get_list()
Because you don’t have a return
statement in your function, you’ll get this error:
Traceback (most recent call last):
File "main.py", line 4, in <module>
a, b, c = get_list()
TypeError: cannot unpack non-iterable NoneType object
A Python function returns None
by default when it has no return
statement, and that’s why this error occurs.
To fix this error, you need to define a return
statement in the function as follows:
def get_list():
my_list = [1, 2, 3]
return my_list
a, b, c = get_list()
print(a, b, c) # 1 2 3
The error is now resolved and we can run the code just fine.
I hope this tutorial helps. See you in other articles! 👋