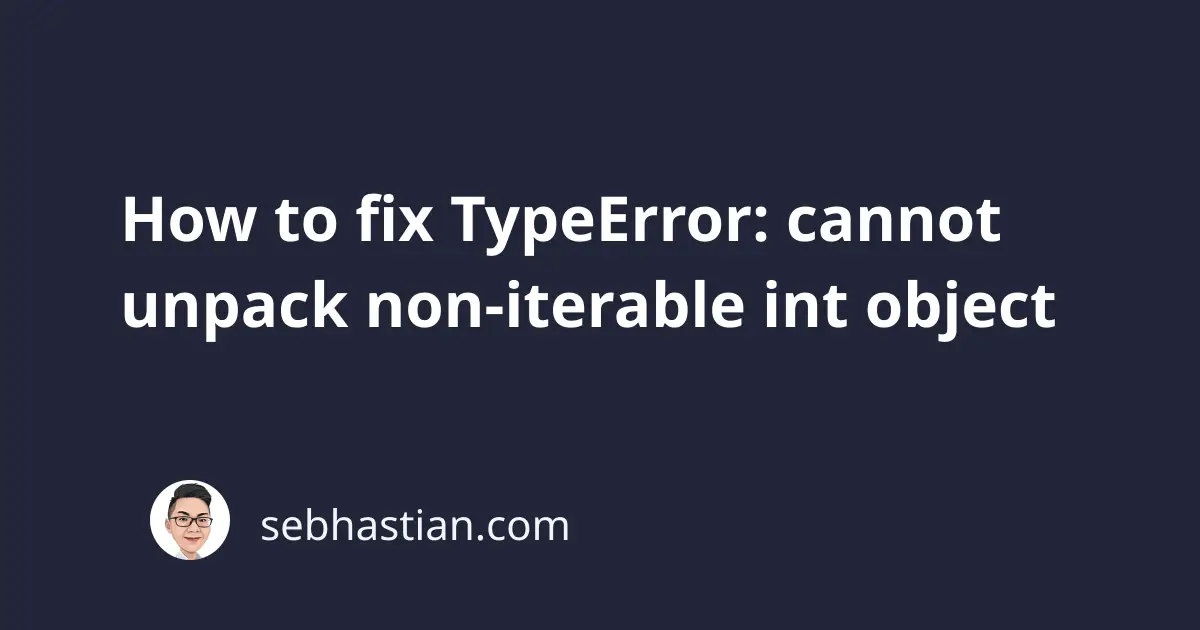
One error that you might encounter when running Python code is:
TypeError: cannot unpack non-iterable int object
This error occurs when you mistakenly attempt to unpack a single int
object.
To fix this error, you need to use the unpacking assignment only on iterable objects, like a list or a tuple.
This tutorial will show you an example that causes this error and how to fix it.
How to reproduce this error
Here’s an example code that produces this error:
first, second = 2
# TypeError: cannot unpack non-iterable int object
At times, people encounter this error when they attempt to unpack the values returned by a function.
Suppose we have a function with an if-else
condition, and the conditions have different return values as follows:
def get_coordinates(x, y):
if y > 1:
return (x , y)
else:
return x
The if
condition returns a tuple, while the else
condition only returns a single value x
.
Next, we call the function as follows:
a, b = get_coordinates(27, 0)
Because the arguments fit the else
condition, the single value is returned, and the unpacking assignment causes this error:
Traceback (most recent call last):
File "main.py", line 7, in <module>
a, b = get_coordinates(27, 0)
TypeError: cannot unpack non-iterable int object
You need to put something in the return
statement of the else
condition that will be assigned to the b
variable.
How to fix this error
To resolve this error, you need to make sure that you have an iterable object passed to the unpacking assignment.
When you have two variables to assign, specify two values in the assignment like this:
first, second = 2, 7
When you pass two values as shown above, Python will convert the values into a tuple automatically.
The same solution applies when you have a function with if-else
conditions. Adjust the else
condition to return a tuple. If you have nothing to return, then just return 0
or None
value:
def get_coordinates(x, y):
if y > 1:
return (x , y)
else:
return (x, 0)
You can call the function again. This time, the same arguments work without causing the error.
Other variants of this error
This error has several variants as shown below:
- TypeError: cannot unpack non-iterable float object
- TypeError: cannot unpack non-iterable NoneType object
- TypeError: cannot unpack non-iterable bool object
- TypeError: cannot unpack non-iterable function object
- TypeError: cannot unpack non-iterable class object
These error messages are caused by the same problem: you are sending a non-iterable object to the unpacking assignment.
1. TypeError: cannot unpack non-iterable float object
This error occurs when you specify a single floating number to the unpacking assignment:
a, b = 3.44
# TypeError: cannot unpack non-iterable float object
To resolve this error, you need to send a tuple or a list of floats to the unpacking assignment. The following examples are valid:
a, b = 3.44, 0.0
a, b = 3.44, 0
a, b = [3.44, 0.0]
a, b = [3.44, 0]
2. TypeError: cannot unpack non-iterable NoneType object
This variant error occurs when you attempt to unpack a single None
value with the unpacking assignment.
Here’s an example that causes this error:
a, b = None # ❌
To resolve this error, modify the assignment and add another value to assign to the b
variable.
You can send any value you want:
a, b = None , 0 # ✅
3. TypeError: cannot unpack non-iterable bool object
This error occurs when you try to unpack a single bool
object using the unpacking assignment:
a, b = True
To resolve this error, make sure that you’re sending as many values as the variables used in the assignment:
a, b = True, False # ✅
This way, the error should be resolved.
4. TypeError: cannot unpack non-iterable function object
This error occurs when you assign a function to a variable using the unpacking assignment:
def get_rooms():
return 3, 5
a, b = get_rooms
If you want to call the function and assign the return values to the variables, then you’re missing the parentheses after the function name:
a, b = get_rooms() # ✅
Without the parentheses, Python thinks you’re assigning the function object to another variable instead of calling the function.
Don’t forget to specify arguments for the function if you have any.
5. TypeError: cannot unpack non-iterable class object
This error occurs when you assign a class object to more than one variable using the unpacking assignment.
Suppose you have a Car
class defined as follows:
class Car:
def __init__(self, name):
self.name = name
Next, you attempt to create two Car
objects using the unpacking assignment:
my_car, my_second_car = Car('Toyota')
You receive this error:
my_car, my_second_car = Car('Toyota')
TypeError: cannot unpack non-iterable Car object
To resolve this error, you need to instantiate two objects for the two variables my_car
and my_second_car
.
my_car, my_second_car = Car('Toyota'), Car('Tesla')
Now that you have two Car
objects assigned to the two variables, the error is resolved.
Conclusion
The Python TypeError: cannot unpack non-iterable (type) object
occurs when you unpack an object that’s not iterable.
When you use the unpacking assignment, you need to specify an iterable object such as a list, a tuple, a dictionary, or a set in the assignment.
If you specify a single non-iterable object such as an integer, a boolean, a function, or a class, then this error will be raised.
I hope this tutorial is helpful. Happy coding! 👍