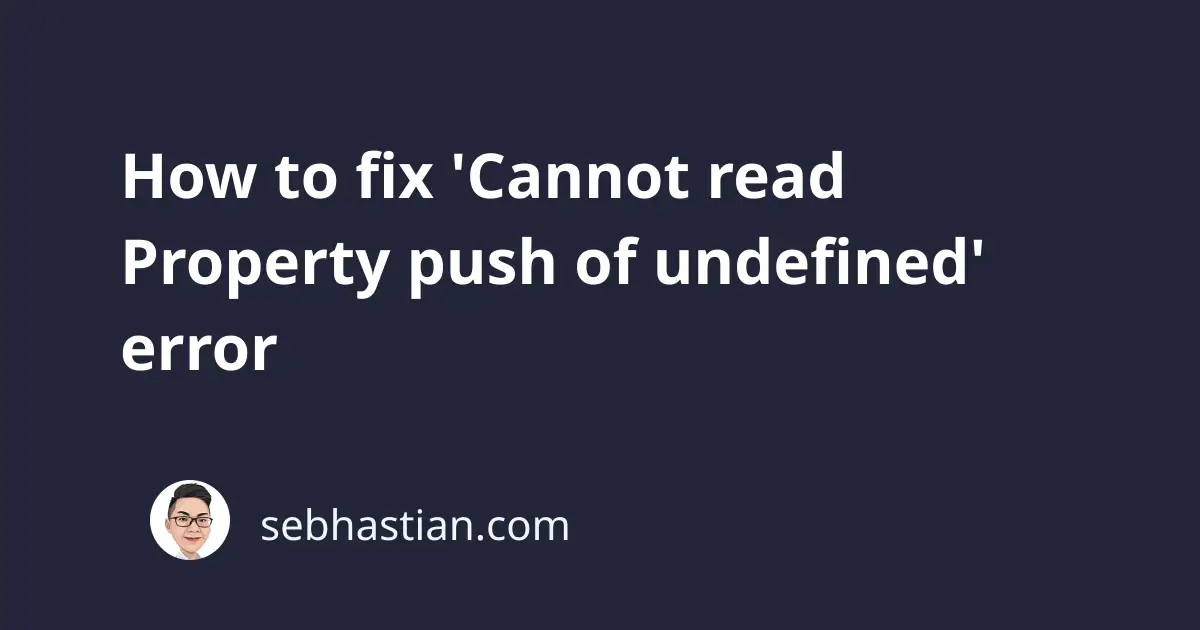
The error “cannot read property ‘push’ of undefined” occurs when you call the push()
method from an undefined
value.
push()
is a method of the array object, so you can’t call it from undefined
. There are 3 possible causes for this error:
- You call the method on a variable containing
undefined
value - You call the method on an array element instead of the array itself
- You call the method on an object property that doesn’t exist
Let’s learn how to resolve the error in each scenario above.
1. Calling push on an undefined variable
Since push()
is a method of the array object, you might see the error when calling it from an undefined
variable:
let arr = undefined;
arr.push(1);
// TypeError: cannot read properties of undefined (reading 'push')
Here, the arr
variable is initialized as undefined
, so the code above throws the error.
If you don’t set the value directly as shown above, you might be expecting a function to return an array as follows:
function getArray() {
let newArray = [1, 2, 3];
}
let arr = getArray();
arr.push(1);
In this case, the getArray()
function is expected to return an array, but notice there’s no return
statement inside the function.
When nothing is returned, a function would return the value undefined
implicitly. The arr
variable gets the value undefined
again, and the error happens.
To resolve this error, you need to specify a return
statement in the function above:
function getArray() {
return [1, 2, 3];
}
let arr = getArray();
arr.push(1);
console.log(arr); // [ 1, 2, 3, 1 ]
Awesome! The code runs and the push()
method successfully adds a new element to the array.
Sometimes, you need to create a new array to store the result of some operation on an existing array.
For example, the following code tries to make a new array containing elements of an existing array incremented by 2:
let newArray;
let myArray = [1, 2, 3];
for (const element of myArray) {
let result = element + 2;
newArray.push(result);
}
Here, the newArray
variable is an uninitialized variable, which means it implicitly contains the value undefined
.
When you call the push()
method to add the result
variable, the error is triggered.
To resolve the error, you need to initialize the newArray
variable into an empty array as follows:
let newArray = [];
let myArray = [1, 2, 3];
for (const element of myArray) {
let result = element + 2;
newArray.push(result);
}
Run the code again, and you’ll see the error now disappears.
2. Calling push on an array element instead of the array itself
One common cause for this error is that you’re trying to call the method from an array element.
Array methods can only be called from an array object, so if your array element is a number or a string, the push()
method can’t be called from it. For example:
const myArray = [1, 3, 4];
myArray[1].push(2); // ❌
In the code above, there’s a square bracket [1]
next to the myArray
variable reference. Here, we try to insert the element 2
between 1
and 3
.
But the push()
method can only insert an element at the end of the array, so this code won’t work.
If you need to insert an element at a specific index, you need to use the splice()
method as shown below:
const myArray = [1, 3, 4];
myArray.splice(1, 0, 2);
console.log(myArray); // [ 1, 2, 3, 4 ]
The splice()
method is a handy method used for adding, removing, and changing elements of an array. The method has 3 parameters:
startIndex
where splice will begin its operation, beginning from0
deleteCount
- an integer representing how many elements to delete from the start index. You can pass0
as well....elements
- elements that you want to add to the array beginning from thestartIndex
When you pass 0
as the second parameter, the method won’t delete anything. It will insert the elements you passed as the third parameter beginning at the startIndex
.
To get a sense of what the method is doing, you can change the first parameter to 2
then 3
. When you need to add an element at a specific position, use splice()
instead of push()
.
3. Calling push on an object property that doesn’t exist
This error occurs when you try to call the push()
method on an object property that doesn’t exist or is undefined
. Consider the example below:
const user = { name: 'Nathan', scores: [30] };
const user2 = { name: 'Sebhastian' };
user.scores.push(50); // ✅
user2.scores.push(50); // ❌
Here, the push()
method is called from the scores
property of user
and user2
objects. The error occurs when you call the method from user2
because that object doesn’t have the scores
property.
Essentially, the property is undefined
, and you get the error. To resolve this, you can use the optional chaining operator ?.
next to the property to ensure it’s not undefined
:
const user = { name: "Nathan", scores: [30] };
const user2 = { name: "Sebhastian" };
user.scores?.push(50);
user2.scores?.push(50);
console.log(user); // { name: 'Nathan', scores: [ 30, 50 ] }
console.log(user2); // { name: 'Sebhastian' }
When the property doesn’t exist, the optional chaining operator skips the method you want to call from that property.
If you want to push the value successfully, you need to initialize the property in user2
object as follows:
const user2 = { name: "Sebhastian", scores: [] };
Just initialize the property with an empty array so that you can push values to it.
Wrapping up
This article has shown you how to fix the error “cannot read property ‘push’ of undefined”, which occurs when you call the push()
method on an undefined
value.
There are various scenarios that may cause this error, but the root cause is always the same. To resolve the error, you need to make sure that you call the push()
method from a variable or property that exists and is an array.
I hope this article helps. Have fun coding! 👋