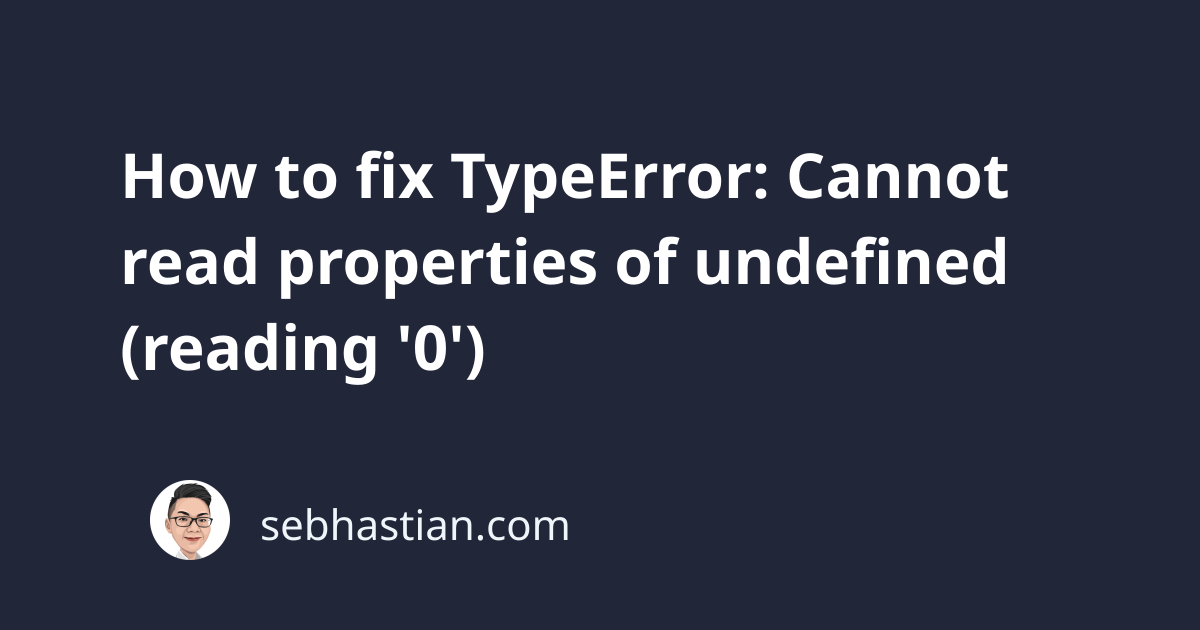
When running JavaScript code, you might encounter an error that says:
TypeError: Cannot read properties of undefined (reading '0')
This error occurs when you try to access an element at index 0 using square brackets ([0]
) but the variable you’re accessing has an undefined
value.
You probably try to access an array, a string, or an object in your code, but they haven’t been defined yet.
Let me show you an example that causes this error and how I fix it.
How to reproduce this error
Suppose you declare a variable without initializing it with any value, then you try to access the property 0 of the variable as follows:
let myVar;
console.log(myVar[0]);
When you execute the code above, JavaScript will respond with an error:
TypeError: Cannot read properties of undefined (reading '0')
Because undefined
is the default value for any variable declared in JavaScript, the error essentially means JavaScript can’t read properties of undefined
value.
It adds (reading ‘0’) because you’re trying to access property [0]
. If you change it to 1
or ['xyz']
then the message will adjust properly.
To prevent this error, you need to avoid accessing the properties of an undefined
value.
How to fix this error
There are 3 possible solutions for this error:
- Provide a fallback value that will be used in place of undefined
- Initialize any variable you have with an empty value
- Use an
if
statement to check forundefined
values - For nested array, use the optional chaining
?.
operator
Let’s explore these solutions one by one.
Provide a fallback value that will be used in place of undefined
To fix the error, you can provide a fallback value that will be used in place of undefined.
For example, you can use the logical OR ||
operator like this:
let result;
// Provide an array as fallback value
let myVar = result || ["a", "b", "c"];
console.log(myVar[0]); // a
As you can see, because the variable result
is undefined
, the logical OR operator will return the value on the right-hand side.
This way, you can provide a fallback value should the value of the left-hand side is undefined
.
Another way to provide a fallback value is to use the nullish coalescing operator ??
as shown below:
let result;
// Provide a string as fallback value
let myVar = result ?? "Hello!";
console.log(myVar[0]); // H
The nullish coalescing operator ??
returns the value on the left-hand side only when it’s not null
or undefined
. Otherwise, the right-hand side will be returned.
Initialize any variable you have with an empty value
Another way to prevent this error is to initialize any variable you used with a defined value.
You can simply use an empty value as shown below:
let myVar = '';
console.log(myVar[0]);
Because an empty string is not undefined
, the error won’t show when you execute the code above.
Instead, the value of property [0]
will be undefined because the string is empty.
Use an if
statement to check for undefined
values
Before you access the property of a variable, you can check if the value of that variable is undefined
using an if
statement.
Consider the example below:
let myVar;
if (myVar) {
console.log(myVar[0]);
} else {
console.log("Nothing is printed");
}
By default the if
statement only gets executed if the expression evaluates to true
. The value undefined
, null
, and false
will evaluate as false
, so the if
block will be skipped.
If you try to run the code above, the else
block will be executed instead.
For nested array, use the optional chaining ?.
operator
When you’re working with a nested array, the error can happen when you access an element that doesn’t exists.
For example, the following code tries to access the element at index [2][1]
:
const arr = [
[1, 2],
[3]
];
console.log(arr[2][1]);
Since the array only has 2 nested arrays, the maximum length of the first index is [1]
, but where we passed [2]
so the error occurs.
To avoid the error, we need to use the optional chaining ?.
operator as follows:
const arr = [
[1, 2],
[3]
];
console.log(arr[2]?.[1]); // undefined
The optional chaining operator returns the element if it exists. Otherwise, it returns undefined
. Using the operator will prevent the error from popping up.
I hope this tutorial helps. Happy coding!