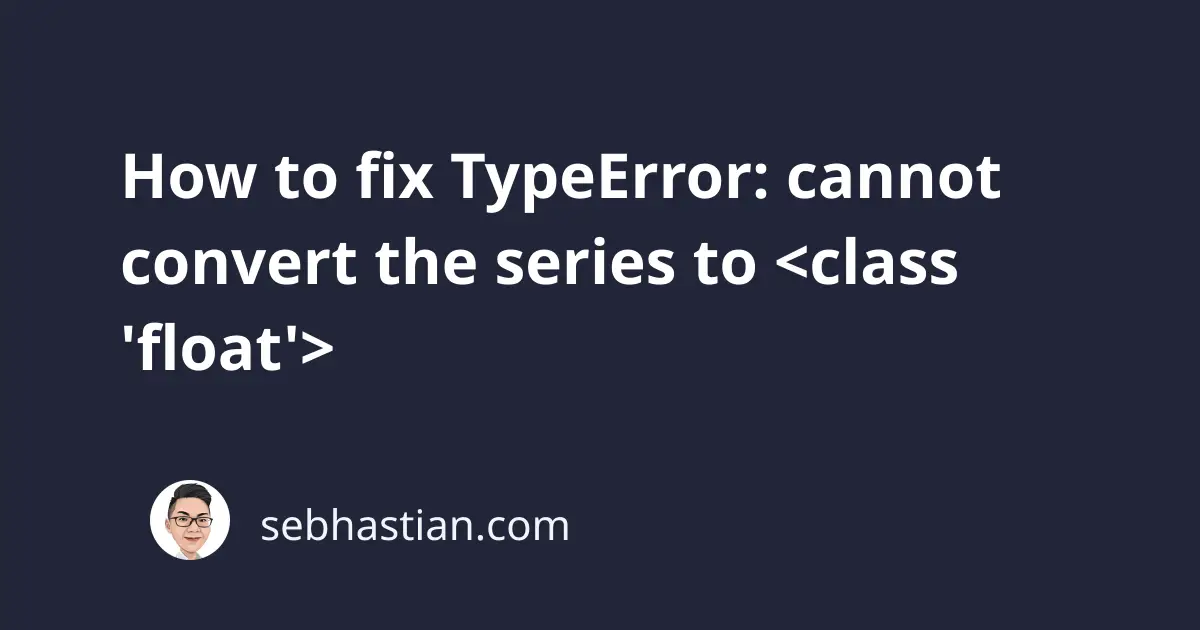
While working with pandas DataFrame, you might encounter an error that says:
TypeError: cannot convert the series to <class 'float'>
This error usually occurs when you try to do mathematical operations using a pandas DataFrame object.
This tutorial shows examples that cause this error and how to fix it.
What triggers this error
Let’s say you have a DataFrame object that has a single column called distance
.
This column contains float values that measure the distance from one address to the other in miles:
import pandas as pd
df = pd.DataFrame({"distance": [3.6, 18.3, 21.5, 25.2]})
Alright, suppose you need to create a new column that rounds the distance
values up.
You then call the math.ceil()
function and pass the distance
column to the function like this:
import pandas as pd
import math
df = pd.DataFrame({"distance": [3.6, 18.3, 21.5, 25.2]})
df['distance_rounded'] = math.ceil(df['distance'])
Output:
Traceback (most recent call last):
File "main.py", line 6, in <module>
df['distance_rounded'] = math.ceil(df['distance'])
File "/opt/homebrew/lib/python3.10/site-packages/pandas/core/series.py",
line 185, in wrapper
raise TypeError(f"cannot convert the series to {converter}")
TypeError: cannot convert the series to <class 'float'>
Whoops! Python responded with an error because the math.ceil()
function can’t process a series.
This function only accepts a single int or float object, but here we’re passing a DataFrame column, which is a series object.
Aside from the math.ceil()
function, there are many other functions and operations that can cause this error.
For example, when you use the float()
function on a pandas column as follows:
import pandas as pd
df = pd.DataFrame({"distance": [3.6, 18.3, 21.5, 25.2]})
df['float_distance'] = float(df['distance']) # ❌
You need to look into the Traceback logs and see which part of your code code is causing this error.
How to fix this error
To fix this error, you need to use a function that supports iterating over a series object.
One such function is available from the numpy
library, which has the numpy.ceil()
function.
You need to install the library using pip
as follows:
pip install numpy
# If you have pip3:
pip3 install numpy
Then import the library in your code to use its ceil()
function:
import pandas as pd
import numpy as np
df = pd.DataFrame({"distance": [3.6, 18.3, 21.5, 25.2]})
df['distance_rounded'] = np.ceil(df['distance'])
print(df)
Here’s the output:
distance distance_rounded
0 3.6 4.0
1 18.3 19.0
2 21.5 22.0
3 25.2 26.0
As you can see, this time we didn’t get an error because np.ceil()
supports a series object.
The function is able to iterate over the object and work on each value that exists in the object.
If you don’t want the .0
decimal points in your data, you can cast the result as integers using .astype(int)
like this:
df['distance_rounded'] = np.ceil(df['distance']).astype(int)
The distance_rounded
column will have an int64
series instead of float64
.
Another way to convert a series without using the numpy
library is to use Python list comprehension syntax as follows:
import pandas as pd
import math
df = pd.DataFrame({"distance": [3.6, 18.3, 21.5, 25.2]})
df['distance_rounded'] = [math.ceil(v) for v in df['distance']]
print(df)
Output:
distance distance_rounded
0 3.6 4
1 18.3 19
2 21.5 22
3 25.2 26
The list comprehension works by going through the series object and creating a new series object with the results. This can come in handy when you don’t have a ready-made function that supports a series object.
Now you’ve learned how to fix the error TypeError: cannot convert the series to <class 'float'>
while working with pandas DataFrame in Python.
I hope this tutorial is helpful. Happy coding! 👍