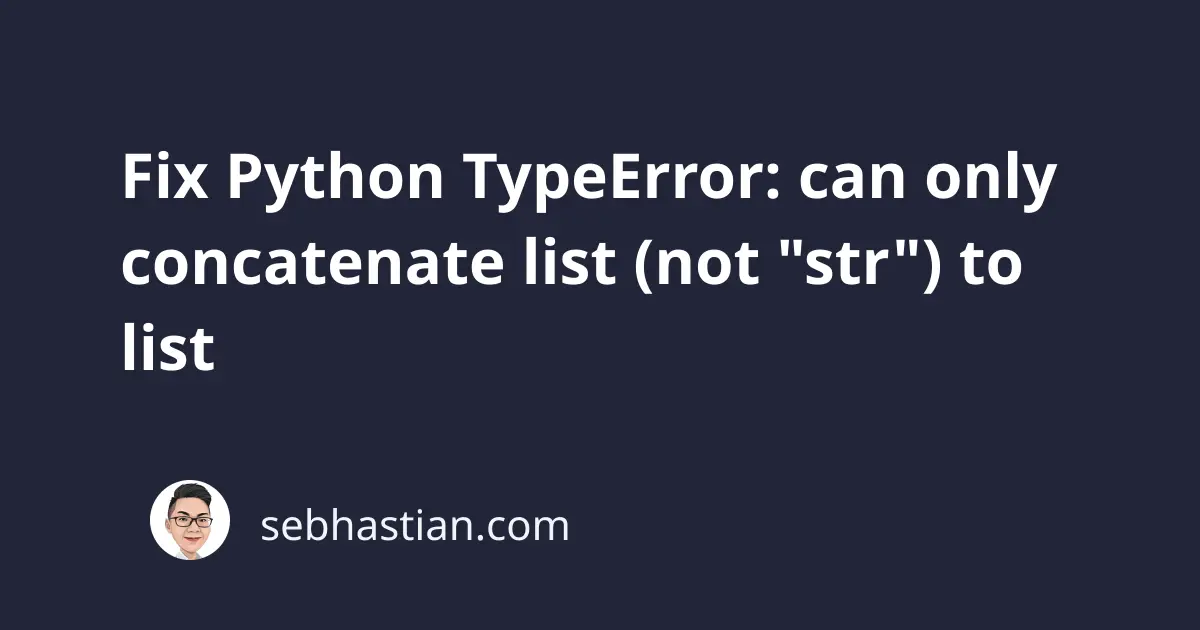
Your Python code shows TypeError: can only concatenate list (not “str”) to list when you try to concatenate or join a string with a list.
To fix this error, you need to add the string to the list using the append()
method.
Let’s see an example where this problem can happen. Suppose you have a list of bird names called birds
as shown below:
birds = ["eagle", "falcon", "hawk"]
new_list = birds + "crow" # ❗️TypeError
In the above code, we are trying to add crow
to the birds
list.
But since crow
is a string type and not a list, Python responds with a TypeError as shown below:
In Python, the +
operator can only be used with two values of the same type (a list with a list, a string with a string)
To add a string to a list, you need to use the append()
method provided in the list object as shown below:
birds = ["eagle", "falcon", "hawk"]
birds.append("crow") # ✅
print(birds)
# ['eagle', 'falcon', 'hawk', 'crow']
Alternatively, you can also convert the string value into a list by surrounding it with square brackets:
list_from_string = ["crow"]
This is useful when you have more than one value to add to a list as follows:
birds = ["eagle", "falcon"]
# 👇 concatenate a list with a list
new_list = birds + ["hawk", "crow"]
print(new_list)
# ['eagle', 'falcon', 'hawk', 'crow']
While Python doesn’t allow concatenating a string with a list, you can merge two lists as one just fine.
Keep in mind that the append()
method mutates the original list, so you need to create a copy if you still need it:
numbers = [1, 2, 3]
# copying a list
numbers_copy = numbers.copy()
print('Copied List:', numbers_copy)
# Copied List: [1, 2, 3]
On the other hand, concatenating lists using the +
operator returns a new value without changing the original list.
You can also concatenate two lists as one using the extend()
method as shown below:
list_a = [1, 2]
list_b = ['a', 'b', 'c']
list_a.extend(list_b)
print(list_a)
# [1, 2, 'a', 'b', 'c']
The extend()
method adds all the elements of an iterable such as a list, tuple, or string to the end of the list.
Just like the append()
method, the extend()
method changes the original list.
These solutions are good for adding values to a list, so choose the one you prefer.
Conclusion
To conclude, the Python TypeError: can only concatenate list (not “str”) to list occurs when you try to merge a list with a string using the +
operator.
This error is raised because the +
operator can only be used with values of the same type.
To fix this error, you need to make sure that you are only trying to concatenate two lists or convert the string to a list before concatenating it.
You can use the list()
function to convert a string to a list, or you can enclose the string with square brackets []
.
Finally, you can also use the append()
or extend()
method to add values to the original list.
The examples in this article should help you fix this TypeError.