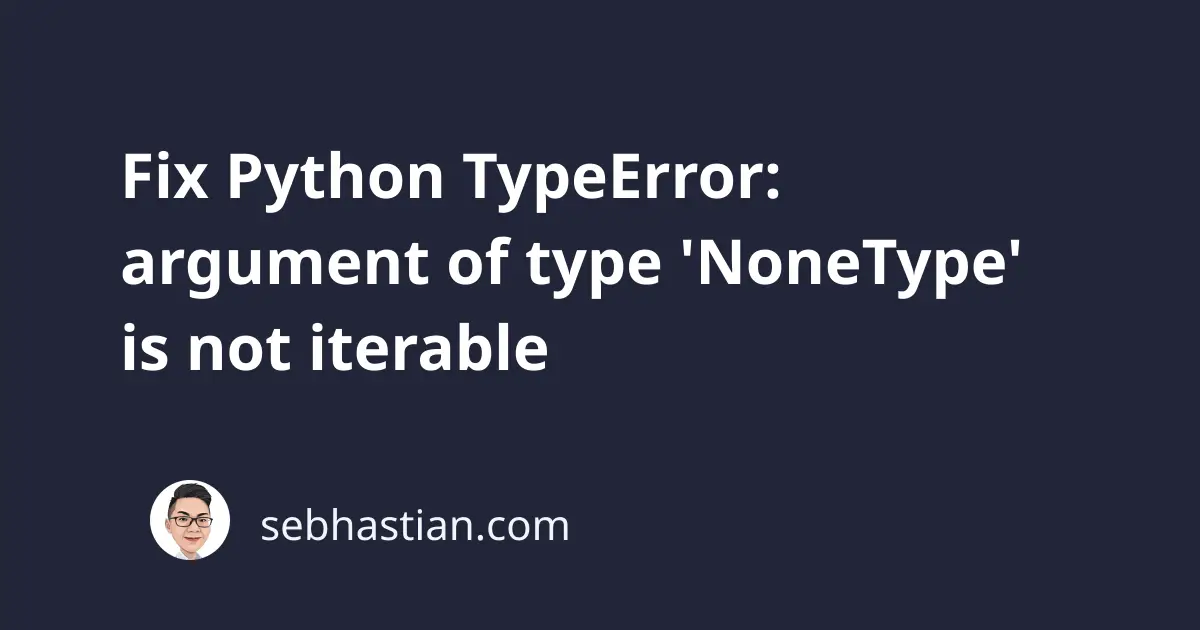
Python shows “TypeError: argument of type ‘NoneType’ is not iterable” error when you use the membership operator (in
, not in
) on a None
object.
To fix this error, you need to adjust your code so that you are not using the membership operator on a None
object. See this article to learn how.
In Python, the membership operator is used to check if an element is contained in a sequence, such as a list, tuple, or string. However, the NoneType is not a sequence, and it is not iterable.
Here’s an example of code that would trigger this error:
items = None
# ❌ TypeError: argument of type 'NoneType' is not iterable
if "a" in items:
print("a is in the list.")
The code above uses the membership operator in
to check if the string “a” is contained in the items
variable.
Since items
is None
, which is not iterable, the code will cause an error as shown below:
To fix this error, you need to make sure that you are not trying to use the membership operator on a value that is None
.
You can do this by using an if
statement to check if the variable is None
as shown below:
items = None
if items is not None and "a" in items:
print("'a' is in the list.")
else:
print("The list is empty or does not exist.")
The if
statement in the code above will perform 2 checks:
The if
statement in the code above uses the logical operator and
to combine two conditions: items is not None and a in items
.
The and
operator returns True
when both conditions are true, and False
if either condition is not true.
Alternatively, you can also use an is None
statement and provide a default value for the items
variable when its value is None
.
Consider the code below:
items = None
if items is None:
items = [] # Set items as empty array
print("a" in items) # False
The code above assigns an empty array to the items
variable when that variable has a None
value.
Finally, Keep in mind that Python produces a None
value on certain occasions. You need to be aware of them to avoid this error.
Several common sources of None
value when running Python code are:
- Assigned
None
to a variable explicitly - Calling a function that returns nothing
- Calling a function that returns only under a certain condition
You’ve seen an example of the first case, so let’s look at the second and third cases.
When you call a function that has no return
statement, the function will return a None
value implicitly.
Consider the code below:
def greet():
pass
result = greet()
print(result) # None
When a function doesn’t have a return
statement defined, it returns None
.
This also applies to Python built-in functions, such as the sort()
method of the list
object.
listings = [5, 3, 1]
# call sort() on list
output = listings.sort()
print(output) # None
print(1 in listings) # True ✅
The sort()
method sorts the original listings
variable without returning anything. When you assign the result to a variable, that variable contains None
.
Finally, you can also get None
when you have a function that has a conditional return
statement.
Consider the greet()
function below:
def greet(name):
if name:
return f"Hello {name}!"
# Call greet with empty string
output = greet("")
print(output) # None
output = greet("Nathan")
print("n" in output) # True ✅
The greet
function only returns a string value when the name
variable evaluates to True
.
Since an empty string evaluates to False
, the first call to the greet
function returns None
.
To avoid having None
returned by your function, you need to add another return
statement as follows:
def greet(name):
if name:
return f"Hello {name}!"
return "Hello Unknown!"
In the code above, the second return
statement will be executed when the name
variable evaluates to False
.
Now you’ve learned several common cases that may cause a None
value to enter your code.
By being aware of the sources of the None
value, you can avoid causing the TypeError in your code.
Conclusion
The “TypeError: argument of type ‘NoneType’ is not iterable” error in Python occurs when you use the membership operator (in
, not in
) on a None
object.
To fix this error, you need to adjust your code so that you are not using the membership operator on a None
object.
You can do this by using an if .. is not ..
statement to check if the variable is None before using the operator with and
keyword.
You can also use the is None
expression and provide a default value if the variable contains None
.
Finally, you should be aware of some common sources of the None
value in Python so that you avoid unintentionally causing the error in the first place.