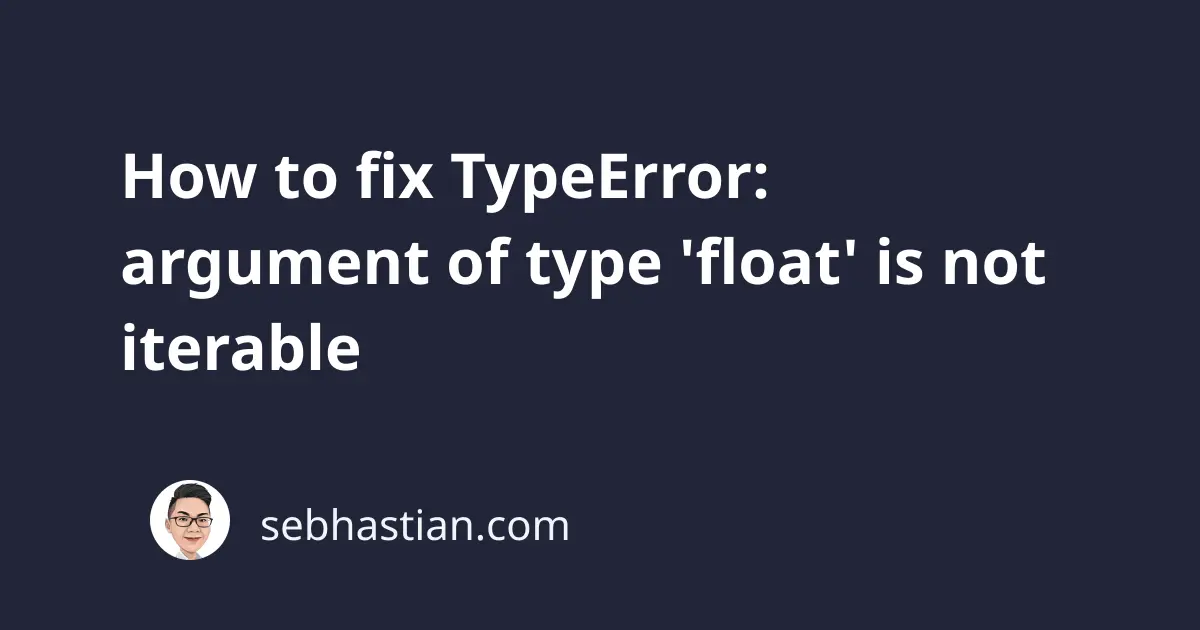
One error that you might encounter in Python is:
TypeError: argument of type 'float' is not iterable
This error usually occurs when you use the membership operators to test the membership of a value in a float object.
This tutorial shows you an example that causes this error and how to fix it.
How to reproduce this error
Suppose you have a float value in your code, and you try to check if that float value contains a certain number as follows:
x = 5.0
if 5 in x:
print('5 exists in x')
The membership operator in
and not in
can only test for membership in a sequence, list, tuple, etc.
When you pass a float variable as shown above, you’ll get this error:
Traceback (most recent call last):
File "main.py", line 4, in <module>
if 5 in x:
TypeError: argument of type 'float' is not iterable
Membership operators can’t be used with float values.
How to fix this error
If you want to test a float, you can convert it to a list by surrounding the variable in square brackets:
x = 5.0
if 5 in [x]:
print('5 exists in x')
Another way you can fix this error is by using the comparison operator ==
to compare two float values:
x = 5.0
if 5 == x:
print('5 exists in x')
When you don’t know the type of the variable, you can use the try-except
statement to gracefully handle the error when the variable is not iterable.
Consider the code below:
x = 5.0
try:
if 5 in x:
print('5 exists in x')
except:
print("The variable x is not an iterable")
Whenever x
is not an iterable, the except
block will be executed.
Conclusion
The error “argument of type ‘float’ is not iterable” occurs when you use the membership operator in
and not in
with a float object.
The membership operator requires an iterable object to execute properly, so you need to convert a float into an iterable to fix this error.
I hope this tutorial is helpful. Until next time! 🙏