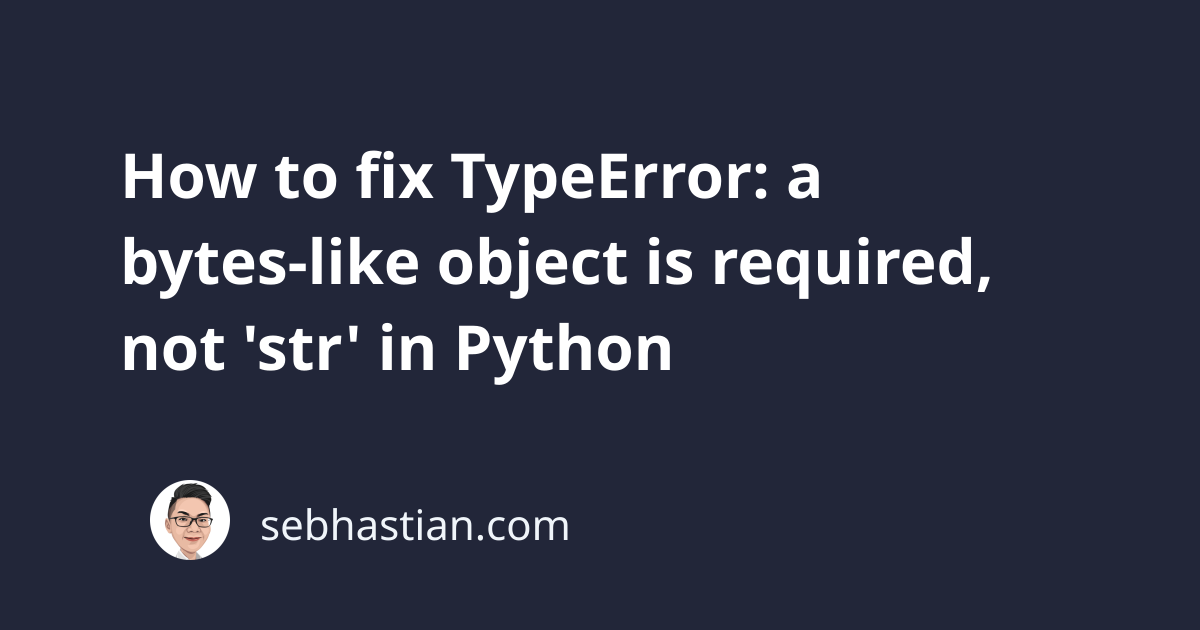
When running Python code, you might encounter this error:
TypeError: a bytes-like object is required, not 'str'
The error occurs when you pass a string type value in a place where Python expects a bytes-like object.
Most likely, you have this error when you open a file in ‘rb’ mode, which reads a file text in binary format.
In this article, I will show you how I fixed this error in practice.
How to reproduce this error
Suppose you have a text file named file.txt
that has the following contents:
Learning how to work with a file.
Python is amazing!
Now suppose you want to print a line from the file only if the line contains the word amazing
.
You used the open()
function to open the file in ‘rb’ mode as shown below:
with open("file.txt", "rb") as file:
lines = file.readlines()
for line in lines:
if "amazing" in line:
print(line)
For each line
in the lines
, you check if the line contains the word amazing
, and print the line if it does.
When running the code, you get this error:
Traceback (most recent call last):
File "main.py", line 6, in <module>
if "amazing" in text:
TypeError: a bytes-like object is required, not 'str'
This error occurs because you opened the file in ‘rb’ mode, which causes the text to be read in binary format by Python.
The code will work in Python 2 because Python 2 handles strings as bytes by default. On the other hand, Python 3 handles strings as Unicode characters.
How to fix this error
There are two ways you can resolve this error in Python 3:
- Change the
open()
function mode from ‘rb’ to ‘r’ - Use the
b
prefix in places where Python expected bytes-like object
The first solution is to use the open()
function in the regular read mode so that the strings are treated as Unicode characters:
with open("file.txt", "r") as file:
lines = file.readlines()
for line in lines:
if "amazing" in line:
print(line)
This way, Python will read the text in the Unicode format, and the print()
function will print the line as a string as well.
The second solution is to put the b
prefix in front of the string where Python expected a bytes-like object. Here’s an example:
with open("file.txt", "rb") as file:
lines = file.readlines()
for text in lines:
if b"amazing" in text:
print(text)
The b
prefix will change the string to a bytes literal, so you won’t receive the same error.
But this also makes Python print the strings in binary format as follows:
b'Python is amazing!'
Notice the letter b
got printed in the output above. When using Python 3, it’s better to use the default string encoding to handle string data.
Conclusion
The error TypeError: a bytes-like object is required, not 'str'
occurs when you put a string where Python expects a bytes-like object.
Most likely, the error happens when you run code from Python 2 in Python 3. In Python 2, the strings are handled in ASCII format, while Python 3 uses Unicode format.
To resolve this error, you need to change the read mode from ‘rb’ to ‘r’, or you can also add the b
prefix before your string in the place where Python expects a bytes-like object.
I hope this tutorial is useful. Happy coding! 👍