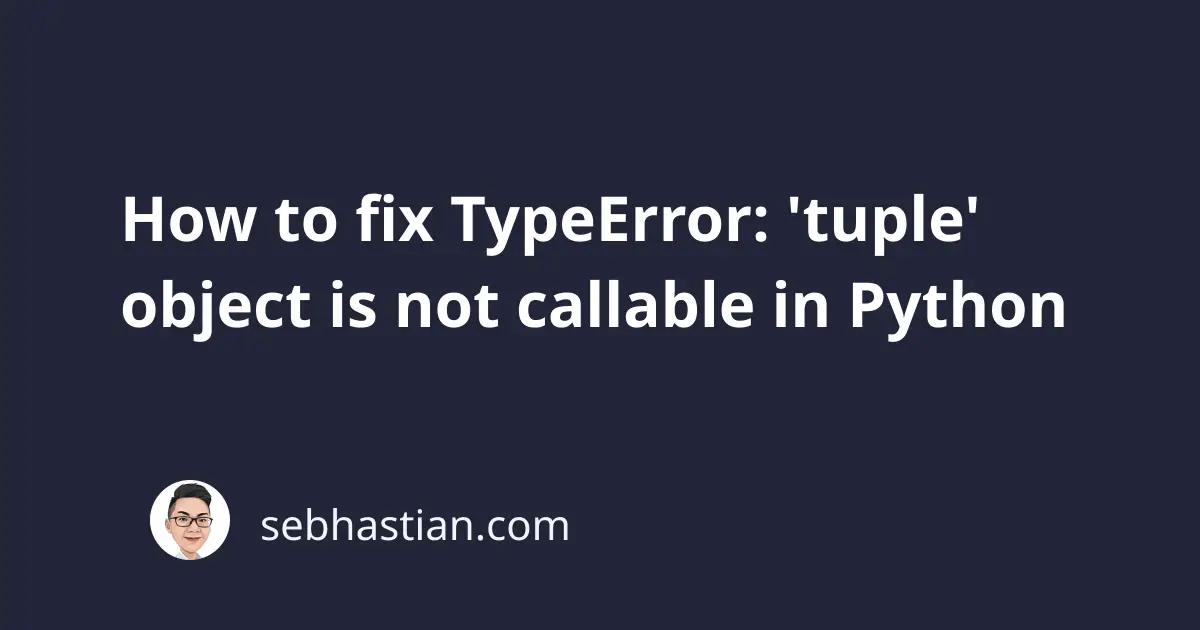
When working with Python, you might encounter the following error:
TypeError: 'tuple' object is not callable
This error occurs when you try to call a tuple object as if it was an object. There are two scenarios where this error might occur:
- You try to access a specific index using parentheses
- You create a list of tuples but forgot to use a comma to separate each tuple
- You mistakenly create a variable named
tuple
Because Python uses parentheses to initialize a tuple and call a function, many programmers unintentionally tripped on this error.
This tutorial shows how to fix the error in each scenario.
1. You used parentheses to access a tuple item at a specific index
Suppose you initialize a Python tuple as follows:
my_tuple = ('a', 'b', 'c')
Next, you try to access the second item in the tuple with the following code:
my_tuple(1)
The output:
Traceback (most recent call last):
File "main.py", line 3, in <module>
my_tuple(1)
TypeError: 'tuple' object is not callable
This error occurs because you used parentheses ()
to access the tuple at a specific index.
To access an item inside a tuple, you need to use the square brackets []
notation.
Here’s the right way to access a tuple item:
my_tuple = ('a', 'b', 'c')
x = my_tuple[1]
print(x) # b
Notice that this time we didn’t receive the error.
2. You created a list of tuples but forgot to use a comma to separate each tuple
This error also occurs when you create a list of tuples, but forgot to separate each tuple using a comma.
Here’s an example code that causes the error:
number_list = [
(1, 2, 3)
(4, 5, 6)
]
The number_list
contains two tuples, but there’s no comma separating them.
As a result, Python thinks you’re putting a function call notation next to a tuple like this:
my_tuple = (1, 2, 3)
number_list = [
my_tuple(4, 5, 6)
]
To resolve this error, you need to separate each tuple using a comma as follows:
number_list = [
(1, 2, 3),
(4, 5, 6)
]
By adding a comma between two tuples, the error should be resolved.
3. You created a variable named tuple
in your source code
The built-in function named tuple()
is used to convert an object of another type into a tuple object.
For example, here’s how to convert a list into a tuple:
my_list = [1, 2, 3]
my_tuple = tuple(my_list)
print(my_tuple) # (1, 2, 3)
If you mistakenly create a variable named tuple
, then the tuple()
function would be overwritten.
When you call the tuple()
function, the error would occur as follows:
tuple = (1, 2, 3)
another_tuple = tuple(['a', 'b'])
Output:
Traceback (most recent call last):
File "/main.py", line 3, in <module>
another_tuple = tuple(['a', 'b'])
TypeError: 'tuple' object is not callable
Because you created a variable named tuple
, the tuple()
function gets replaced with that variable, causing you to call a tuple object instead of a tuple function.
To avoid this error, you need to declare the variable using another name:
my_tuple = (1, 2, 3) # ✅
another_tuple = tuple(['a', 'b'])
This way, the keyword tuple
would still point to the tuple function, and the error won’t be raised.
Conclusion
The TypeError: 'tuple' object is not callable
occurs when you mistakenly call a tuple object as if it’s a function.
To resolve this error make sure that:
- You don’t access a tuple item using parentheses
- When creating multiple tuples, you separate each tuple with a comma
- You don’t use the
tuple
keyword as a variable name
By following the steps above, you can avoid the error appearing in your source code.
I hope this tutorial is helpful. See you in other tutorials! 👋