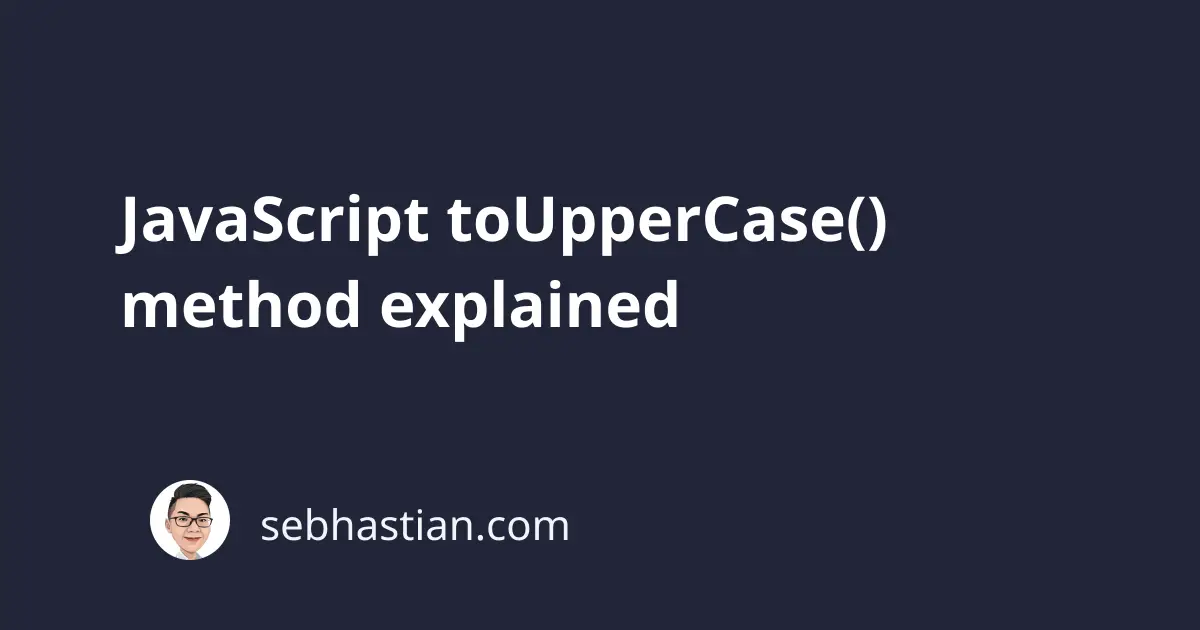
The JavaScript toUpperCase()
method is a built-in method of the String
prototype object that allows you to convert a string into its all uppercase version.
In the example below, the string good morning
is transformed using the toUpperCase()
method:
let myString = "good morning";
console.log(myString.toUpperCase());
// GOOD MORNING
The toUpperCase()
method accepts any valid string, but this method won’t have any effect if you pass a number
as a string
like this:
"50".toUpperCase();
// returns '50'
Using the toUpperCase()
method, you can capitalize the first letter of a sentence with the following code:
function upperCaseFirstLetter(str) {
return str.charAt(0).toUpperCase() + str.substring(1);
}
let myString = "good morning";
let newString = upperCaseFirstLetter(myString);
console.log(newString); // Good morning
The first letter G
in the string above is capitalized while the rest remain in lowercase.
You can also capitalize the first letter of every word by creating a custom function that makes use of the toUpperCase()
method.
First, you need to split your string sentence into an array:
let strArray = myString.split(" ");
Next, you need to map()
over the array and capitalize the first letter with the upperCaseFirstLetter()
function above:
strArray = strArray.map((str) => {
return upperCaseFirstLetter(str);
});
Finally, you need to join the strArray
back into a single string with the join()
method.
The full code is as shown below:
function upperCaseFirstLetter(str) {
return str.charAt(0).toUpperCase() + str.substring(1);
}
let myString = "good morning";
let strArray = myString.split(" ");
strArray = strArray.map((str) => {
return upperCaseFirstLetter(str);
});
let newString = strArray.join(" ");
console.log(newString); // Good Morning
The code to capitalize the string can be extracted as a function like this:
function upperCaseFirstLetter(str) {
return str.charAt(0).toUpperCase() + str.substring(1);
}
function capitalizeWords(sentence){
let strArray = sentence.split(" ");
strArray = strArray.map((str) => {
return upperCaseFirstLetter(str);
});
return strArray.join(" ");
}
let myString = "good morning you awesome human!";
console.log(capitalizeWords(myString));
// Good Morning You Awesome Human!
And that’s how you can capitalize a sentence using JavaScript toUpperCase()
method.