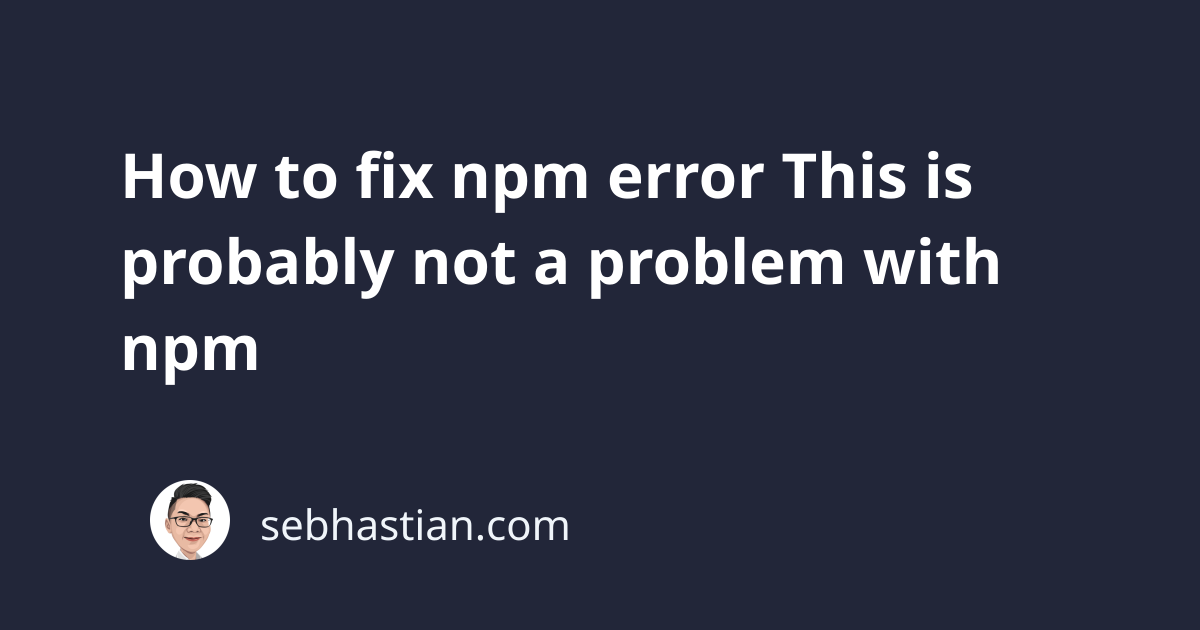
When running npm commands to build or start your JavaScript projects, you may encounter an error that says This is probably not a problem with npm.
For example, the following error is displayed when the command npm start
is executed to run a React application:
npm ERR! code ELIFECYCLE
npm ERR! errno 1
npm ERR! [email protected] start: `react-scripts start`
npm ERR! Exit status 1
npm ERR!
npm ERR! Failed at the [email protected] start script.
npm ERR! This is probably not a problem with npm.
There is likely additional logging output above.
npm ERR! A complete log of this run can be found in:
npm ERR! /Users/nsebhastian/.npm/_logs/2022-05-09T03_20_51_340Z-debug.log
Since the cause of this error varies, you need to investigate the cause of this error further to pinpoint the issue.
Usually, you can find some valuable info in the generated debug.log
file.
Try to read the log file using the cat
command as follows:
cat /Users/nsebhastian/.npm/_logs/2022-05-09T03_20_51_340Z-debug.log
Paste the absolute path to the debug.log
file into the console to see the details of the log.
If you can’t pinpoint the issue, then you need to try some fixes that may help resolve this error.
Here are six ways you can try to resolve the issue:
Run with sudo or admin privileges
The first thing you can try is to run the command using the sudo
program:
sudo npm start
# or
sudo npm build
The sudo
command will run npm
using administrator privileges. This should resolve the error when the cause is permission issues.
If you still see the error, then you need to try other fixes.
Make sure all required packages are installed.
This error can also be caused when you have a required package that’s not yet installed on your Node project.
For example, React application requires react-scripts
package to run:
"scripts": {
"start": "react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test",
"eject": "react-scripts eject"
},
When you don’t have react-scripts
installed, then the error will appear:
$ npm start
> [email protected] start /Users/nsebhastian/Desktop/DEV/react-app
> react-scripts start
sh: react-scripts: command not found
npm ERR! code ELIFECYCLE
npm ERR! syscall spawn
npm ERR! file sh
npm ERR! errno ENOENT
npm ERR! [email protected] start: `react-scripts start`
npm ERR! spawn ENOENT
npm ERR!
npm ERR! Failed at the [email protected] start script.
npm ERR! This is probably not a problem with npm.
There is likely additional logging output above.
npm ERR! A complete log of this run can be found in:
npm ERR! /Users/nsebhastian/.npm/_logs/2022-05-09T04_52_45_256Z-debug.log
To solve this error, just install the required package using npm
as follows:
npm install react-scripts
That should resolve the error when it’s caused by missing packages.
Increase the memory allocated for npm scripts
Sometimes, the error occurred because the JavaScript heap is out of memory.
Instead of just running your usual npm
command like npm run start
or npm run build
, you need to use the node
command --max-old-space-size
option.
The full command template is as follows:
node --max-old-space-size=4096 `which npm` install
The above command will run npm install
with 4GB of memory allocated for the process.
You can modify the command above to suit your needs:
# run build command
node --max-old-space-size=4096 `which npm` run build
# run start command
node --max-old-space-size=4096 `which npm` run start
The increased memory allocation should help you run the process successfully.
Clean your project node_modules and npm cache
Node modules require the exact npm version that installed those modules and scripts.
When you install multiple versions of Node using Node Version Manager (or NVM) then you need to make sure that you are using the version of Node that installed the modules in the first place.
For example, suppose you install the modules using npm version 6.14.16
which is included in Node v12
.
When you upgrade npm by installing newer versions of Node (v16
use npm v8.1.0
) then running npm commands like npm start
may cause the error.
To fix this, you need to clear your installed modules:
rm -rf node_modules
rm package-lock.json
Optionally, you might also clear your npm cache:
npm cache clean --force
Once done, run npm install
to reinstall your modules.
Now you should be able to run your npm commands successfully.
For Conda users: turn off the Conda environment
If you installed Conda on your computer, then you may trigger this error when you run React or Vue applications.
The error output looks as follows:
Error: getaddrinfo ENOTFOUND x86_64-apple-darwin13.4.0
at GetAddrInfoReqWrap.onlookup [as oncomplete] (dns.js:56:26)
npm ERR! code ELIFECYCLE
npm ERR! errno 1
npm ERR! [email protected] start: `react-scripts start`
npm ERR! Exit status 1
npm ERR!
npm ERR! Failed at the [email protected] start script.
npm ERR! This is probably not a problem with npm.
There is likely additional logging output above.
npm ERR! A complete log of this run can be found in:
npm ERR! /Users/nsebhastian/.npm/_logs/2022-05-11T03_30_07_401Z-debug.log
This is because the Webpack development server used to serve your application tries to bind the local webserver to the HOST environment variable.
Conda changes the HOST environment variable from your computer to the virtual environment. This causes the dev server unable to find your localhost
address.
To fix this, you need to unset the HOST
variable with the command below:
unset HOST
Now you should be able to run React or Vue applications successfully.
When you need to run the Conda process again, you can restart the environment:
conda deactivate
conda activate
That should set the HOST
variable back to x86_64-apple-darwin13.4.0
for Mac computers.
Alternatively, you can also turn off Conda from automatically activating the base
environment with the following command:
conda config --set auto_activate_base False
Now the base
environment of Conda will not run automatically when you open a new terminal window.
You need to turn on Conda manually with conda activate
command.
For Windows: Add system32 to your PATH environment variable
For Windows computers, try to add C:\Windows\System32
to your system’s PATH
environment variables.
First, Go to My Computer or This PC for Windows 10. Right-click on empty space and open the Properties window:
Click Advanced system settings from the left bar of the Properties window:
Now you’re in the System Properties window. Click the Environment Variables...
button:
Now you’re in the Environment Variables window. Select the Path
variable from the System Variables table and click on the Edit...
button:
See if you have the system32
on the path. It could be listed as %SystemRoot%\system32
as shown below:
When you don’t see the variable above, you can add C:\Windows\System32
as a new entry to your Path
variable.
Restart the terminal you used to run the npm
commands by closing it and opening a new one.
Conclusion
The npm error This is probably not a problem with npm
is one of the most frustrating errors you can find when developing a JavaScript application.
When the six methods described above don’t work, then the last thing you can do is to read the debug.log
file generated by npm.
You need to analyze the debug log slowly to find the root cause.
You can also try posting what you find in StackOverflow or Reddit threads to let others help you with the issue. Please make sure you include as many details as possible (including any fixes you have tried)
Good luck in resolving the error! 👍