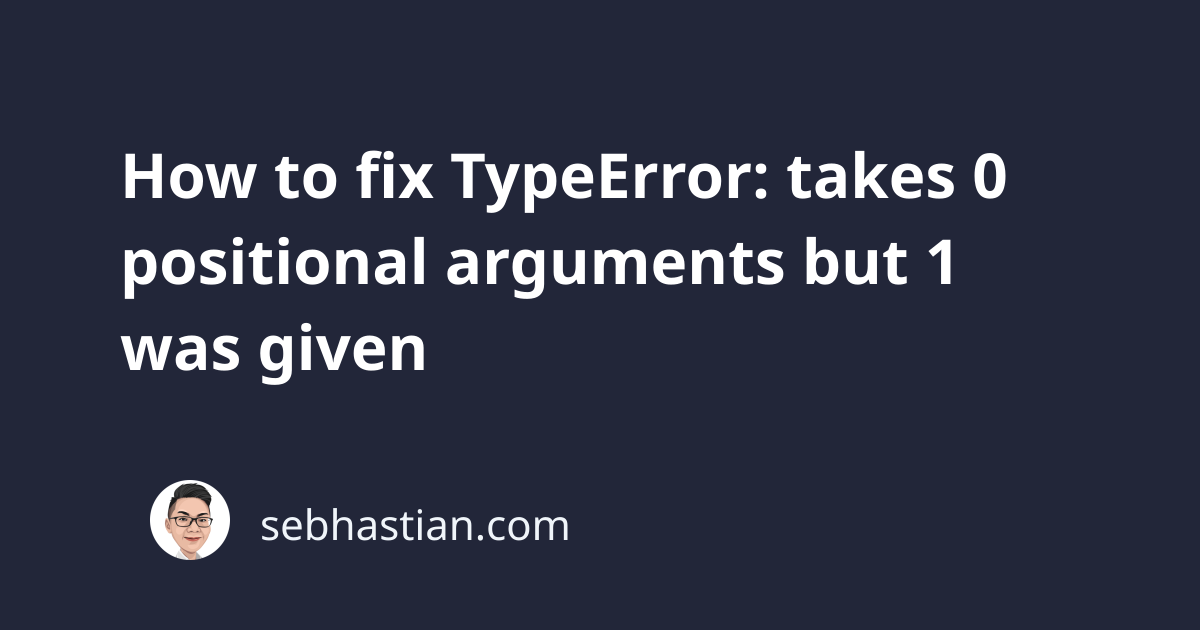
When working with Python functions and classes, you might see the following error:
TypeError: function() takes 0 positional arguments but 1 was given
This error usually occurs when you call and pass an argument to a function that accepts 0 arguments.
There are two possible reasons why this error happens:
- Your function accepts 0 arguments, but you pass it an argument when calling
- You specify a class method without the
self
parameter
The following tutorial shows how to fix this error in both scenarios.
Note: arguments are called parameters when you specify them in the function header.
1. The function accepts 0 arguments
Suppose you have a function named greet()
that has no parameter.
You then called the function and pass it an argument as shown below:
def greet():
print("Hello World!")
greet("John") # ❌
Python tries to send the argument John
to the greet()
function, but the function takes 0 arguments so the error occurs:
Traceback (most recent call last):
File "main.py", line 8, in <module>
greet("John")
TypeError: greet() takes 0 positional arguments but 1 was given
To fix this error, you need to remove the argument when you call the function, or you can specify parameters for the function as follows:
# 1. Remove the argument from the function call
def greet():
print("Hello World!")
greet() # Hello World!
# 2. Add parameter to the function
def greet(name):
print(f"Hello {name}!")
greet("John") # Hello John!
You’ll also get this error when you pass too many arguments to a function.
In the code below, the greet()
function takes one argument but you give it two:
def greet(name):
print(f"Hello {name}!")
greet("John", 29)
# TypeError: greet() takes 1 positional argument but 2 were given
When the function takes one argument, you need to pass it one argument.
2. A class method without the self
parameter
This error also happens when you specify a class method without the self
parameter.
Suppose you have the create a class named Human
that has the following definition:
class Human:
def talk():
print("Talking")
Next, you instantiate an object from the class and call the talk()
method:
person = Human()
person.talk() # ❌
Output:
TypeError: Human.talk() takes 0 positional arguments but 1 was given
This error occurs because Python internally passes the class instance (the object itself) whenever you call a method of that class.
To resolve this error, you need to specify self
as the parameter of all methods in your class even when you didn’t need it:
class Human:
def talk(self):
print("Talking")
person = Human()
person.talk() # Talking
This time, we didn’t get any error because the talk()
method accepts the self
argument that gets passed automatically when you call it.
To conclude, the error TypeError: method() takes 0 positional arguments but 1 was given
occurs when you pass one argument to a function that doesn’t take any.
You can fix this error by specifying the parameters required by the function, or you can remove the argument when calling that function.
I hope this tutorial is helpful. Enjoy coding! 👍