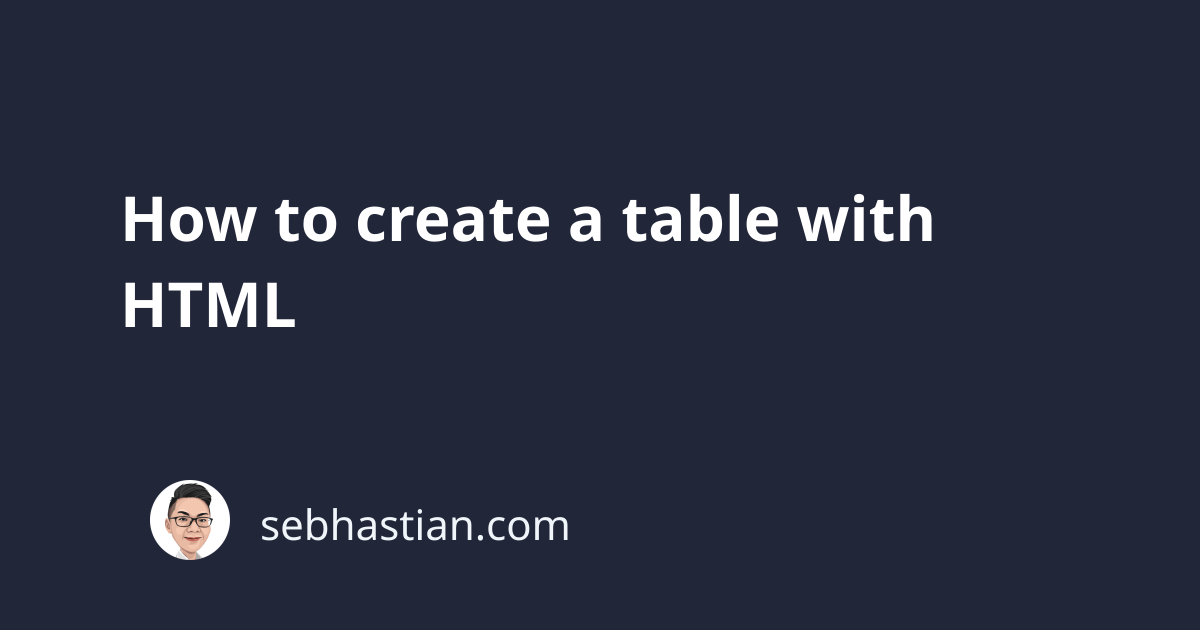
To create a table using HTML, you can use the provided <table>
tag and its helper tags.
The HTML structure of a basic table is as shown below:
<body>
<table>
<tr>
<td>Cell one</td>
<td>Cell two</td>
</tr>
<tr>
<td>Cell three</td>
<td>Cell four</td>
</tr>
</table>
</body>
The output of the HTML above will be as follows:
By default, an HTML table only provides the structure for organizing your tabular data.
You need to add some CSS style to improve the display of your table. The following CSS will do:
table,
td {
border: 1px solid black;
}
Using the CSS above, your HTML table will look as follows:
To remove the double border between the table and the cells, you need to add the border-collapse
CSS property to the table
as follows:
table {
border-collapse: collapse;
}
Now the borders will collapse into one as shown below:
HTML <table>
tag has many helper tags to help you structure and organize the display of your data neatly. The helper tags are as follows:
<tr>
tag to create a table row<td>
tag to create a table cell<th>
tag to create a header cell, specifying the data group
And then there’s threee table grouping tags:
<thead>
- specifies the header portion to your table<tbody>
- specifies the body portion to your table<tfoot>
- specifies the footer portion to your table
Let’s see how they work, starting with the <tr>
tag.
HTML table tr tag
The <tr>
tag allows you to add a new table row inside your <table>
element. It usually contains one or more <th>
or <td>
tag.
You’ve already seen how the <tr>
tag is used in the example table above:
Once you have the <tr>
tag placed inside the <table>
tag, it’s time to add the <td>
tags.
HTML table td tag
The <td>
tag allows you to add a new table cell inside your table row. A table requires at least one <tr>
tag and one <td>
tag to make it viable.
The example table above has four <td>
tags separated in two <tr>
tags:
The <td>
tag also has two attributes that can help you to merge cells:
rowspan
- spans the cell into several rowscolspan
- spans the cell into several columns
For example, here’s how to use rowspan
to span a cell into two rows:
<body>
<table>
<tr>
<td rowspan="2">Cell one</td>
<td>Cell two</td>
<td>Cell three</td>
</tr>
<tr>
<td>Cell four</td>
<td>Cell five</td>
</tr>
</table>
</body>
The output will be as shown below:
Finally, you can also add the colspan
attribute so that the <td>
cell will span horizontally:
<body>
<table>
<tr>
<td colspan="2">Cell one</td>
</tr>
<tr>
<td>Cell two</td>
<td>Cell three</td>
</tr>
</table>
</body>
The output will be as follows:
Next, let’s look at the <th>
tag.
HTML th tag
The <th>
tag allows you to create a table header cell. A header cell is used to group all the data below it, informing the user of the data specification.
For example, suppose you have a table listing all your expenses between January and March.
You can display your expenses using HTML table as follows:
<body>
<table>
<tr>
<th>Month</th>
<th>Expense (in USD)</th>
</tr>
<tr>
<td>January</td>
<td>700</td>
</tr>
<tr>
<td>February</td>
<td>500</td>
</tr>
<tr>
<td>March</td>
<td>800</td>
</tr>
</table>
</body>
Next, add the border
CSS attribute to the th
element as shown below:
table,
td,
th {
border: 1px solid black;
}
Your output table will be as follows:
Usually, the browser will render a <th>
element text with bold style and aligned on the center of the cell.
HTML table grouping with thead, tbody, and tfoot tags
The HTML <thead>
, <tbody>
, and <tfoot>
tags are used to specify the portion of the table as the header, the body and the footer.
The tags are used to futher organize your table. Any row that you add to the <thead>
and <tfoot>
tags will not be scrolled when you have a scrolling table.
Here’s an example of an HTML table using the grouping tags:
<body>
<table>
<thead>
<tr>
<th>Month</th>
<th>Expense (in USD)</th>
</tr>
</thead>
<tbody>
<tr>
<td>January</td>
<td>700</td>
</tr>
<tr>
<td>February</td>
<td>500</td>
</tr>
<tr>
<td>March</td>
<td>800</td>
</tr>
</tbody>
<tfoot>
<tr>
<td>Total</td>
<td>2000</td>
</tr>
</tfoot>
</table>
</body>
A few notes on customizing HTML table
Changing table alignment
You can change the alignment of your HTML table by adding the margin
CSS property to your <table>
tag.
For example, to center the table, you need to add margin-left: auto
and margin-right: auto
properties:
table.center {
margin-left: auto;
margin-right: auto;
}
Then add the class center
to your <table>
tag:
<table class="center">
</table>
To align the table to the right, only add the margin-left:auto
property to the <table>
tag:
table.right {
margin-left: auto;
}
To change the alignment of each cell content, you can add the text-align
property to the corresponding element.
When you group the table content using <tbody>
tag, you can add the text-align
property to the <tbody>
tag to affect all <tr>
and <td>
tags inside it:
tbody.center {
text-align: center;
}
Next, you need to add the center
class to the <tbody>
tag.
Changing table width
You can also set the width
attribute of the <table>
tag to 100%
to fit the table width as the container element’s width:
table.expand {
width: 100%;
}
The height of the table usually grows as the size of the table rows increase, so you don’t have to set it.
td vertical-align property
There’s also the vertical-align
property that you can use to modify the vertical alignment of each cell.
For example, you can set the vertical-align
property to top
so that the text content will be vertically aligned to the top:
td {
vertical-align: top;
height: 40px;
}
If you want to bold the text inside each cell, then you need to add the font-style: bold
property to your <td>
tags.