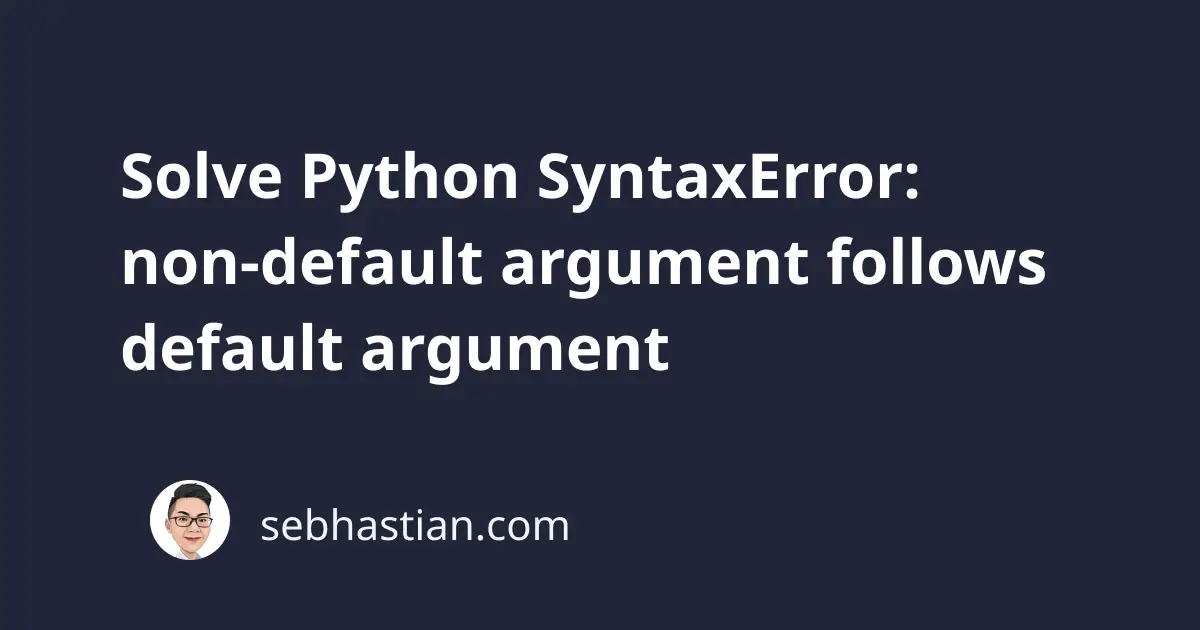
A SyntaxError: non-default argument follows default argument occurs in Python when you define an argument with no default value after an argument with a default value.
To solve this error, you need to reorder your function’s definition so that all arguments with no defaults are defined before arguments with defaults.
I know it might sound confusing, so see the code below for a quick fix:
def greet(name, greeting="Hello", title): # ❗️SyntaxError
def greet(name, title, greeting="Hello"): # ✅ Correct
To understand why this error occurs and how to resolve it, it’s important to first understand what default arguments are and how they work in Python.
What are default arguments?
In Python, you can define default arguments for a function by specifying a default value for a parameter in the function definition.
Default arguments allow you to specify a default value for a parameter in case the user does not provide a value for that parameter when calling the function.
For example, consider the following function definition:
def greet(name, greeting="Hello"):
print(greeting + ", " + name)
In this function, the greeting
parameter has a default value of “Hello”.
This means that if the user does not provide a value for the greeting
parameter when calling the function, the default value of “Hello” will be used.
Here’s how you can call this function with and without a value for the greeting parameter:
# 👇 Use the greeting default value
greet("John") # Hello, John
# 👇 Provide a custom value for the greeting parameter
greet("John", "Hi there") # Hi there, John
As you can see, default arguments allow a function to be called without requiring you to explicitly pass an argument for that parameter.
Now that you know how default arguments work, let’s look at why the SyntaxError: non-default argument follows default argument error occurs.
Why the SyntaxError occurs and how to fix it
This error occurs because Python does not allow you to define a non-default argument after a default argument in the same function definition.
This is because non-default arguments are required parameters, while default arguments are optional parameters.
Optional parameters must be defined after the required parameters.
Consider the example below:
def greet(greeting="Hello", name): # ❗️ SyntaxError
print(greeting + ", " + name)
When you put the optional greeting
parameter before the required name
parameter, Python will raise the error.
This is a rule enforced in Python so that you can avoid introducing errors in your code.
To solve this error, you need to make sure that all default arguments are defined after all non-default arguments in the function definition.
Here’s the correct way to define the function above:
def greet(name, greeting="Hello"): # ✅ Correct
print(greeting + ", " + name)
You can even have a mix of default and non-default arguments in a function definition, as long as all default arguments are defined after all non-default arguments.
The following example has two non-default arguments and one default argument:
def greet(name, title, greeting="Hello"): # ✅ Correct
print(greeting + ", " + title + ' ' + name)
greet("John", "Mr.") # Hello, Mr. John
Conclusion
To conclude, the SyntaxError: non-default argument follows default argument error occurs in Python when defining a function with default arguments.
This error happens because Python does not allow you to define a non-default argument after a default argument when defining a function.
To fix this error, you need to make sure that all default arguments are defined after all non-default arguments in the function definition.
By following the examples in this article, you can correctly define functions with default arguments in Python and avoid this error.
I hope this article helps you become better with Python. 🙏