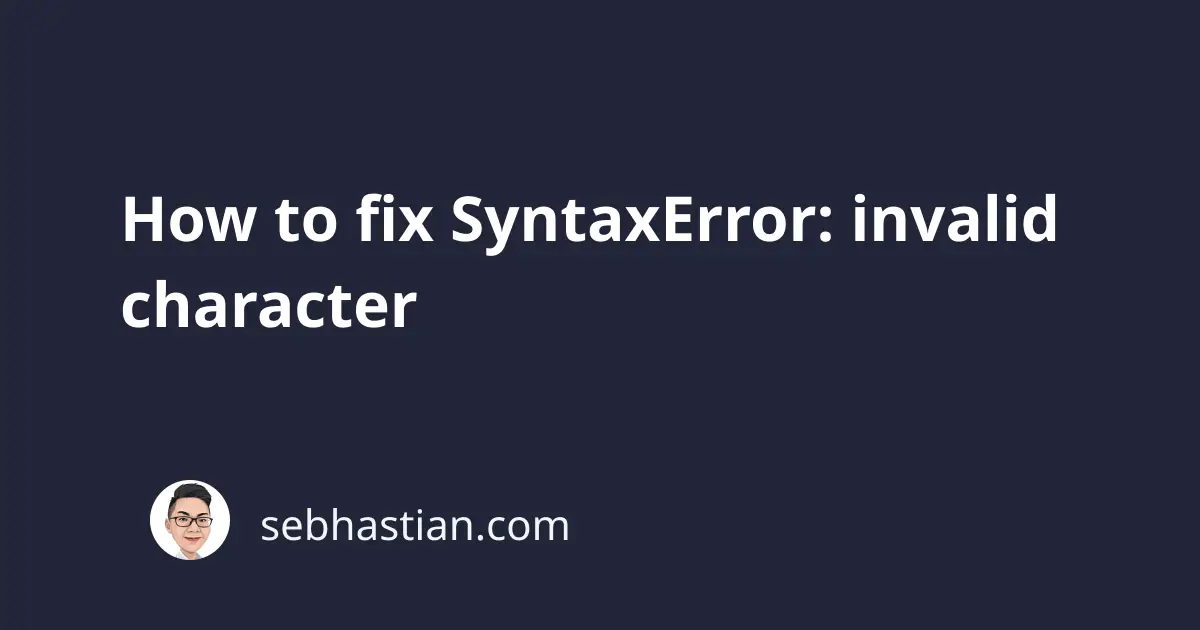
One common error that you might encounter when running Python code is:
SyntaxError: invalid character
This error usually occurs when there’s a character in your source code that can’t be processed by the Python interpreter. The character might be in your function or variable name.
This tutorial shows examples that cause this error and how to fix it.
How to reproduce this error
Suppose you call the print()
function in your code as follows:
print("Hello World")
Although it looks normal, the parentheses in the code above are actually special full width parentheses that have different Unicode characters compared to the regular parentheses.
Running the code above causes the following error:
File "main.py", line 1
print("Hello World")
^
SyntaxError: invalid character '(' (U+FF08)
The full width parentheses symbol has the Unicode hex of U+FF08
and U+FF09
, which is different from the regular parentheses U+2208
and U+2209
.
You can see the comparisons below. Notice how there’s an extra space before the left parenthesis:
print("Hello World")
print("Hello World")
These invalid characters usually come from copy pasting code from websites or PDFs. Since the Python interpreter can’t process these characters, the first line raises an error.
How to fix this error
To resolve this error, you need to make sure that there are no invalid characters in your source code.
The error message should point you to the lines where the invalid characters occur. You need to retype those characters manually:
print("Hello World")
Here’s another example in which the error occurs because of inverted commas in the code:
print(‘Hello World!’)
Output:
print(‘Hello World!’)
^
SyntaxError: invalid character '‘' (U+2018)
If you’re using an IDE with syntax highlighting, you’ll notice that some part of your code isn’t highlighted as usual. The string above is not highlighted with yellow color as in other examples.
Replace those inverted commas by typing single quotes from your keyboard:
print('Hello World!')
Now the string is highlighted as usual, and the print()
function works without any error.
Whenever you see this error, the easiest way to fix it is to retype the line that causes this error manually.
I hope this tutorial is helpful. Happy coding! 😉