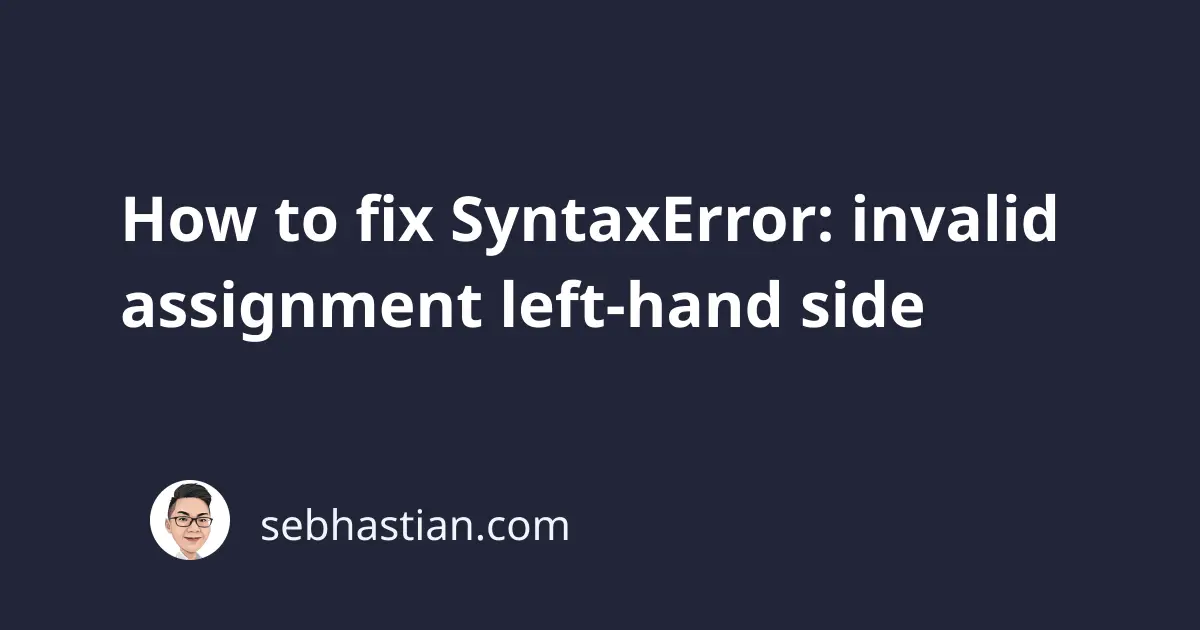
When running JavaScript code, you might encounter an error that says:
SyntaxError: invalid assignment left-hand side
or
SyntaxError: Invalid left-hand side in assignment
Both errors are the same, and they occured when you use the single equal =
sign instead of double ==
or triple ===
equals when writing a conditional statement with multiple conditions.
Let me show you an example that causes this error and how I fix it.
How to reproduce this error
Suppose you have an if
statement with two conditions that use the logical OR ||
operator.
You proceed to write the statement as follows:
let score = 1
if (score = 1 || score = 2) {
console.log("Inside if statement")
}
When you run the code above, you’ll get the error:
SyntaxError: Invalid left-hand side in assignment
This error occurs because you used the assignment operator with the logical OR operator.
An assignment operator doesn’t return anything (undefined
), so using it in a logical expression is a wrong syntax.
How to fix this error
To fix this error, you need to replace the single equal =
operator with the double ==
or triple ===
equals.
Here’s an example:
let score = 1
if (score === 1 || score === 2) {
console.log("Inside if statement")
}
By replacing the assignment operator with the comparison operator, the code now runs without any error.
The double equal is used to perform loose comparison, while the triple equal performs a strict comparison. You should always use the strict comparison operator to avoid bugs in your code.
Other causes for this error
There are other kinds of code that causes this error, but the root cause is always the same: you used a single equal =
when you should be using a double or triple equals.
For example, you might use the addition assignment +=
operator when concatenating a string:
let message = "Hello " += "World!"; ❌
The code above is wrong. You should use the +
operator without the =
operator:
let message = "Hello " + "World!"; ✅
Another common cause is that you assign a value to another value:
"name" = "John"; ❌
This is wrong because you can’t assign a value to another value.
You need to declare a variable using either let
or const
keyword, and you don’t need to wrap the variable name in quotations:
let name = "John"; ✅
You can also see this error when you use optional chaining as the assignment target.
For example, suppose you want to add a property to an object only when the object is defined:
person?.age = 19; ❌
Here, we want to assign the age
property to the person
object only when the person
object is defined.
But this will cause the invalid assignment left-hand side error. You need to use the old if
statement to fix this:
let person = {
name: "John"
}
if (person) person.age = 19;
Now the error is resolved.
Conclusion
The JavaScript error SyntaxError: invalid assignment left-hand side
occurs when you have an invalid syntax on the left-hand side of the assignment operator.
This error usually occurs because you used the assignment operator =
when you should be using comparison operators ==
or ===
.
Once you changed the operator, the error would be fixed.
I hope this tutorial helps. Happy coding!