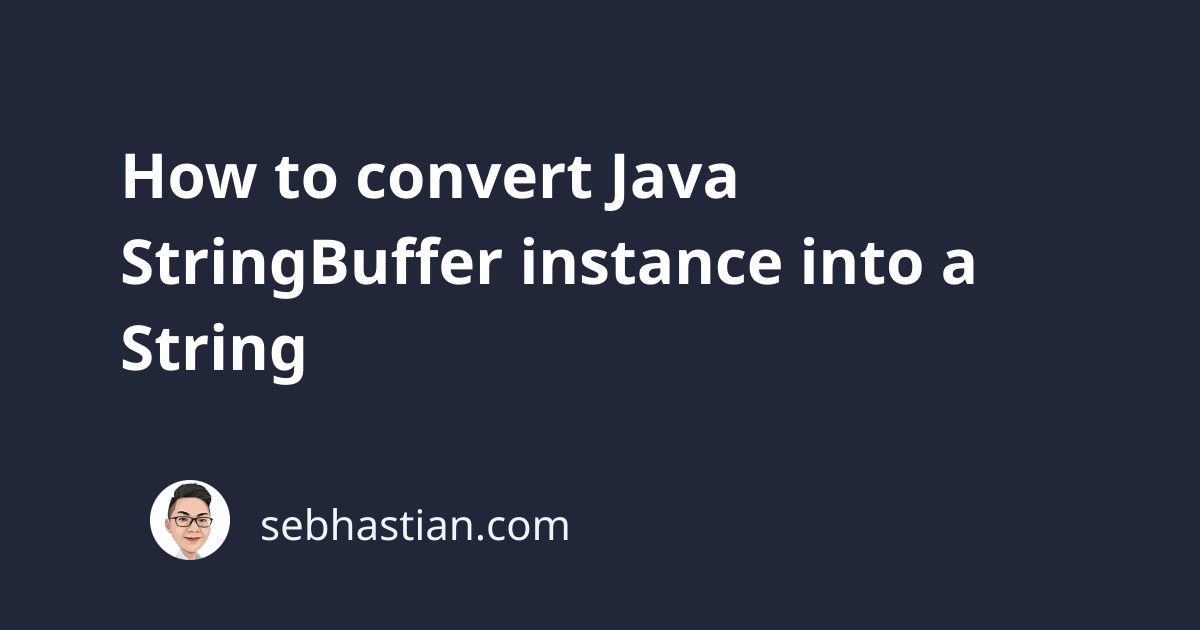
The StringBuffer
class has an built-in method named toString()
that you can use to convert a StringBuffer
class instance into a String
class instance.
The method doesn’t accept any parameter and returns a String
instance.
Here’s an example of using the toString()
method on a StringBuffer
instance.
public static void main(String[] args)
{
StringBuffer sb = new StringBuffer("Hello World!");
String str = sb.toString();
System.out.println("Variable str type: " + str.getClass().getSimpleName());
// Variable str type: String
System.out.println("Variable str content: " + str);
// Variable str content: Hello World!
}
You can also convert a String
to a StringBuffer
by passing the String
instance into the StringBuffer
constructor call:
public static void main(String[] args)
{
String str = "Hello World!";
StringBuffer sb = new StringBuffer(str);
System.out.println("Variable sb type: " + sb.getClass().getSimpleName());
// Variable sb type:StringBuffer
System.out.println(sb);
// Hello World!
}
But if your StringBuffer
instance already has some characters, then you need to use the StringBuffer.append()
method:
public StringBuffer append(Object obj)
The method accepts an Object
argument and then applies the String.valueOf()
method to the argument.
The returned value will then be appended to your StringBuffer
instance.
Here’s how you use the append()
method:
public static void main(String[] args)
{
String str = "Hello World!";
StringBuffer sb = new StringBuffer("Computer says: ");
sb.append(str);
System.out.println("Variable sb type: " + sb.getClass().getSimpleName());
// Variable sb type:StringBuffer
System.out.println(sb);
// Computer says: Hello World!
}
You can’t use the +
operator between StringBuffer
and String
instance to concatenate them as follows:
sb = sb + str;
The +
operator only works for String
instances and values, so you can still concatenate them inside the StringBuffer
constructor call:
StringBuffer sb = new StringBuffer("Computer says: " + str);
The above code works fine for creating a new StringBuffer
instance.